Session
- 클라이언트에 대한 정보를 서버에 저장할 수 있는 공간
- 접속하는 클라이언트 당 하나의 세션 생성
- 사물함과 같은 형식으로 저장이 되며 사물함의 번호를 클라이언트로 전송
- 번호를 분실하는 경우 새로운 세션을 생성하고 다시 클라이언트로 전송
- 설문조사와 같이 여러단계로 입력된 정보, 로그인 정보 등
클라이언트가 접속되어 있는 동안 내용을 기억해야 하는 경우에 활용
세션 페이지 구현
package com.example.basic.controller;
import javax.servlet.http.HttpSession;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import com.example.basic.model.User;
@Controller
public class SessionController {
@GetMapping("sessionLogin")
public String session(){
return "sessionLogin";
}
@PostMapping("sessionLogin")
public String session(User user, HttpSession session) {
System.out.println("userId : "+user.getUserId());
System.out.println("userPw : "+user.getUserPw());
System.out.println("userName : "+user.getUserName());
session.setAttribute("user", user);
return "redirect:/main";
}
@GetMapping("main")
public String main(){
return "main";
}
@GetMapping("logout")
public String logout(HttpSession session){
session.invalidate();
return "redirect:/";
}
}
package com.example.basic.model;
import lombok.Data;
@Data
public class User {
private String userId;
private String userPw;
private String userName;
}
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1> 세션 활용 예시 </h1>
<hr/>
<form action="/sessionLogin" method="post">
<input type="text" placeholder="아이디" name="userId"/>
<input type="password" placeholder="비밀번호" name="userPw"/>
<input type="submit" value="로그인"/>
</form>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>Home 화면 입니다.</h1>
<th:block th:each="dan : ${#numbers.sequence(2, 9)}">
<a th:href="@{/gugudan(dan=${dan})}">구구단 자동생성 2~[[${dan}]]단</a><br />
</th:block>
<a href="/userList">사용자 목록</a><br>
<a href="/login3">로그인하기(세션이용X)</a><br>
<th:block th:if="${session.user} == null">
<a href="/sessionLogin">로그인하기(세션이용O)</a>
</th:block>
<th:block th:unless="${session.user} == null">
<a href="/logout">로그아웃</a>
</th:block>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>메인 페이지입니다.</h1>
<hr />
<th:block th:if="${session.user} != null">
<p>[[${session.user.userId}]]님이 로그인 하셨습니다.</p>
</th:block>
<th:block th:unless="${session.user} != null">
<p>로그인 정보가 존재하지 않습니다.</p>
</th:block>
<a href="/">홈으로 이동</a>
</body>
</html>
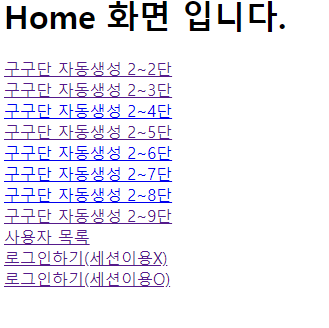
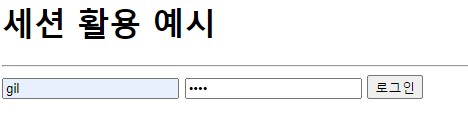
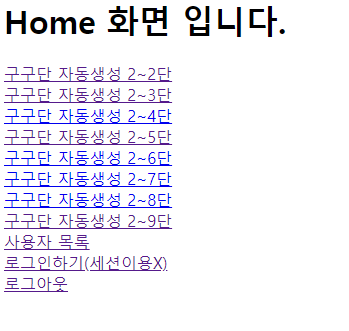
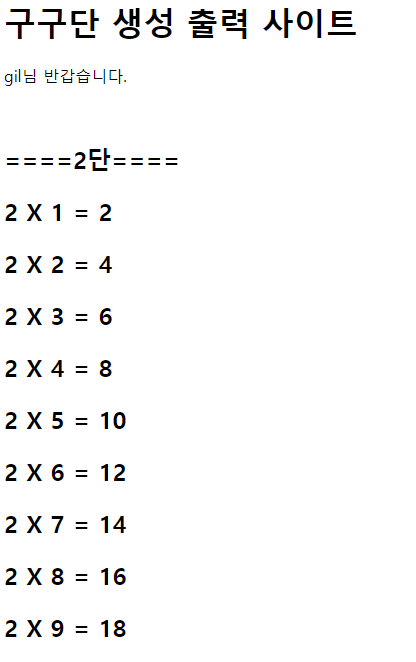
회원가입과 로그인
package com.example.sessac.first.controller;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HomeController {
@GetMapping("/")
public String home(){
return "home";
}
}
package com.example.sessac.first.controller;
import javax.servlet.http.HttpSession;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.example.sessac.first.model.User;
@Controller
@RequestMapping("user")
public class UserController {
@GetMapping("join")
public String join() {
return "user/join";
}
@PostMapping("join")
public String join(HttpSession session, User user){
session.setAttribute("joinUser", user);
return "redirect:/";
}
@GetMapping("login")
public String login(){
return "user/login";
}
@PostMapping("login")
public String login(HttpSession session, User user){
User userData = (User) session.getAttribute("joinUser");
String id = user.getUserId();
String pw = user.getUserPw();
if (userData.getUserId().equals(id) && userData.getUserPw().equals(pw)){
session.setAttribute("user", userData);
} else {
session.setAttribute("user", null);
}
return "redirect:/";
}
@GetMapping("logout")
public String logout(HttpSession session){
session.removeAttribute("user");
return "redirect:/";
}
}
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>Home 화면 입니다.</h1>
<a href="/user/join">회원가입</a>|
<th:block th:if="${session.user} == null">
<a href="/user/login">로그인</a>
</th:block>
<th:block th:unless="${session.user} == null">
<a href="/user/logout">로그아웃</a> | <span>[[${session.user.userName}]]님 환영합니다.</span>
<a href="/board/boardList">게시판</a>
</th:block>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>- 회원가입 -</h1>
<form action="/user/join" method="post">
<input type="text" placeholder="아이디" name="userId" />
<input type="text" placeholder="비밀번호" name="userPw" />
<input type="text" placeholder="이름" name="userName" />
<input type="text" placeholder="주소" name="userAddr" />
<input type="submit" value="회원가입" />
</form>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>- 로그인 -</h1>
<form action="/user/login" method="post">
<input type="text" placeholder="아이디" name="userId" />
<input type="text" placeholder="비밀번호" name="userPw" />
<input type="submit" value="로그인" />
</form>
</body>
</html>
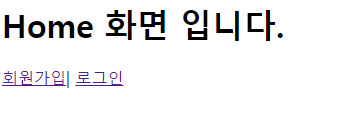

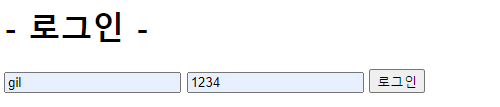
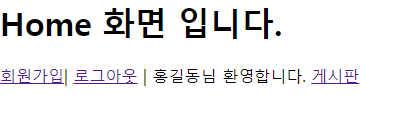
게시판
- 회원가입과 로그인을 하면 게시판을 이용할 수 있게 구현
- 게시글 목록과 내용, 작성, 수정 기능을 구현
package com.example.sessac.first.controller;
import java.util.ArrayList;
import javax.servlet.http.HttpSession;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import com.example.sessac.first.model.Board;
import com.example.sessac.first.model.User;
@Controller
@RequestMapping("board")
public class BoardController {
@GetMapping("boardList")
public String boardList(HttpSession session, Model model){
if(session.getAttribute("boardList") != null){
model.addAttribute("boardList", session.getAttribute("boardList"));
}else{
model.addAttribute("boardList", null);
}
return "board/boardList";
}
@GetMapping("boardCreate")
public String boardCreate(){
return "board/boardCreate";
}
@PostMapping("boardCreate")
public String boardCreate(HttpSession session, Board board){
ArrayList<Board> boardList = new ArrayList<>();
board.setBoardNo("1");
User user = (User) session.getAttribute("user");
board.setBoardWriter(user.getUserName());
boardList.add(board);
System.out.println(" boardList : "+boardList);
session.setAttribute("boardList", boardList);
return "redirect:/board/boardList";
}
@GetMapping("boardDetail")
public String boardDetail(HttpSession session, Model model, @RequestParam("boardNo") String boardNo){
ArrayList<Board> boardList = (ArrayList<Board>) session.getAttribute("boardList");
for(Board board : boardList){
if (board.getBoardNo().equals(boardNo)){
model.addAttribute("board", board);
}
}
return "board/boardDetail";
}
@GetMapping("boardUpdate")
public String boardupdate(HttpSession session, @RequestParam("boardNo") String boardNo, Model model){
ArrayList<Board> boardList = (ArrayList<Board>) session.getAttribute("boardList");
for(Board board : boardList){
if (board.getBoardNo().equals(boardNo)){
model.addAttribute("board", board);
}
}
return "board/boardUpdate";
}
@PostMapping("boardUpdate")
public String boardUpdate(HttpSession session, Board board){
ArrayList<Board> boardList = new ArrayList<>();
boardList.add(board);
System.out.println(" boardList : "+boardList);
session.setAttribute("boardList", boardList);
return "redirect:/board/boardList";
}
}
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<table border="1">
<tr>
<td>게시글번호</td>
<td>게시글제목</td>
<td>작성자</td>
</tr>
<th:block th:if="${boardList} !=null">
<tr th:each="board : ${boardList}">
<td th:text="${board.boardNo}"></td>
<td>
<a th:href="@{/board/boardDetail(boardNo=${board.boardNo})}">[[${board.boardTitle}]]</a>
</td>
<td th:text="${board.boardWriter}"></td>
</tr>
</th:block>
<th:block th:unless="${boardList} != null">
<tr>
<td colspan="3">게시글이 존재하지 않습니다.</td>
</tr>
</th:block>
</table>
<a href="/board/boardCreate">글쓰기</a>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>- 게시글 상세보기 -</h1>
<h3>작성자 : [[${board.boardWriter}]]</h3>
<h3>글 제목</h3>
<textarea name="boardTitle" style="width:100%;" readonly>[[${board.boardTitle}]]</textarea>
<h3>글 내용</h3>
<textarea name="boardContent" style="width:100%; height:200px;" readonly>[[${board.boardContent}]]</textarea>
<a href="/board/boardList">목록으로</a> <a th:href="@{/board/boardUpdate(boardNo=${board.boardNo})}">글수정</a>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>- 게시글 작성 -</h1>
<form action="/board/boardCreate" method="post">
<h3>작성자 : [[${session.user.userName}]]</h3>
<h3>글 제목</h3>
<textarea name="boardTitle" style="width:100%;"></textarea>
<h3>글 내용</h3>
<textarea name="boardContent" style="width:100%; height:200px;"></textarea>
<input type="submit" value="게시글작성"/>
</form>
</body>
</html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
</head>
<body>
<h1>- 게시글 수정 -</h1>
<form action="/board/boardUpdate" method="post">
<input type="hidden" name="boardNo" value="@{board.boardNo}"/>
작성자 : <input type="text" name="boardWriter" th:value="${session.user.userName}"/>
<h3>글 제목</h3>
<textarea name="boardTitle" style="width:100%;">[[${board.boardTitle}]]</textarea>
<h3>글 내용</h3>
<textarea name="boardContent" style="width:100%; height:200px;">[[${board.boardContent}]]</textarea>
<input type="submit" value="게시글수정"/>
</form>
</body>
</html>
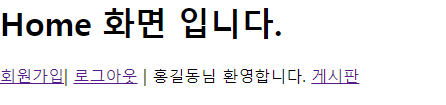
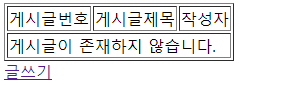
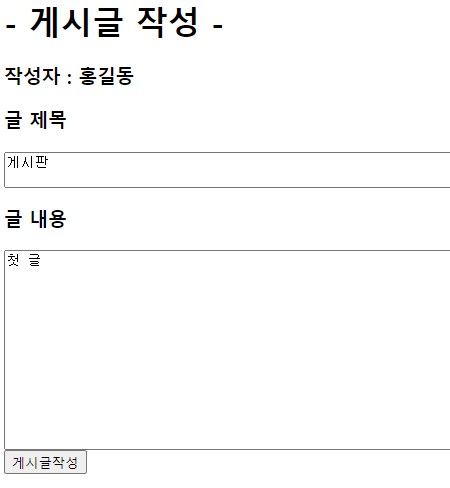
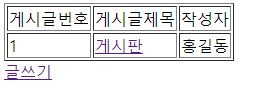
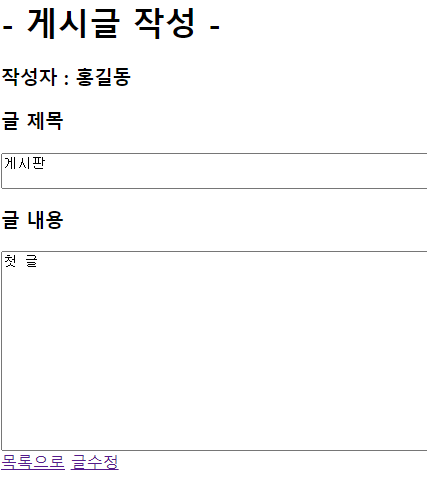
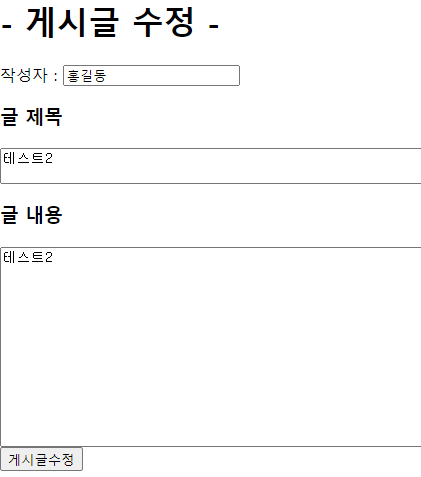