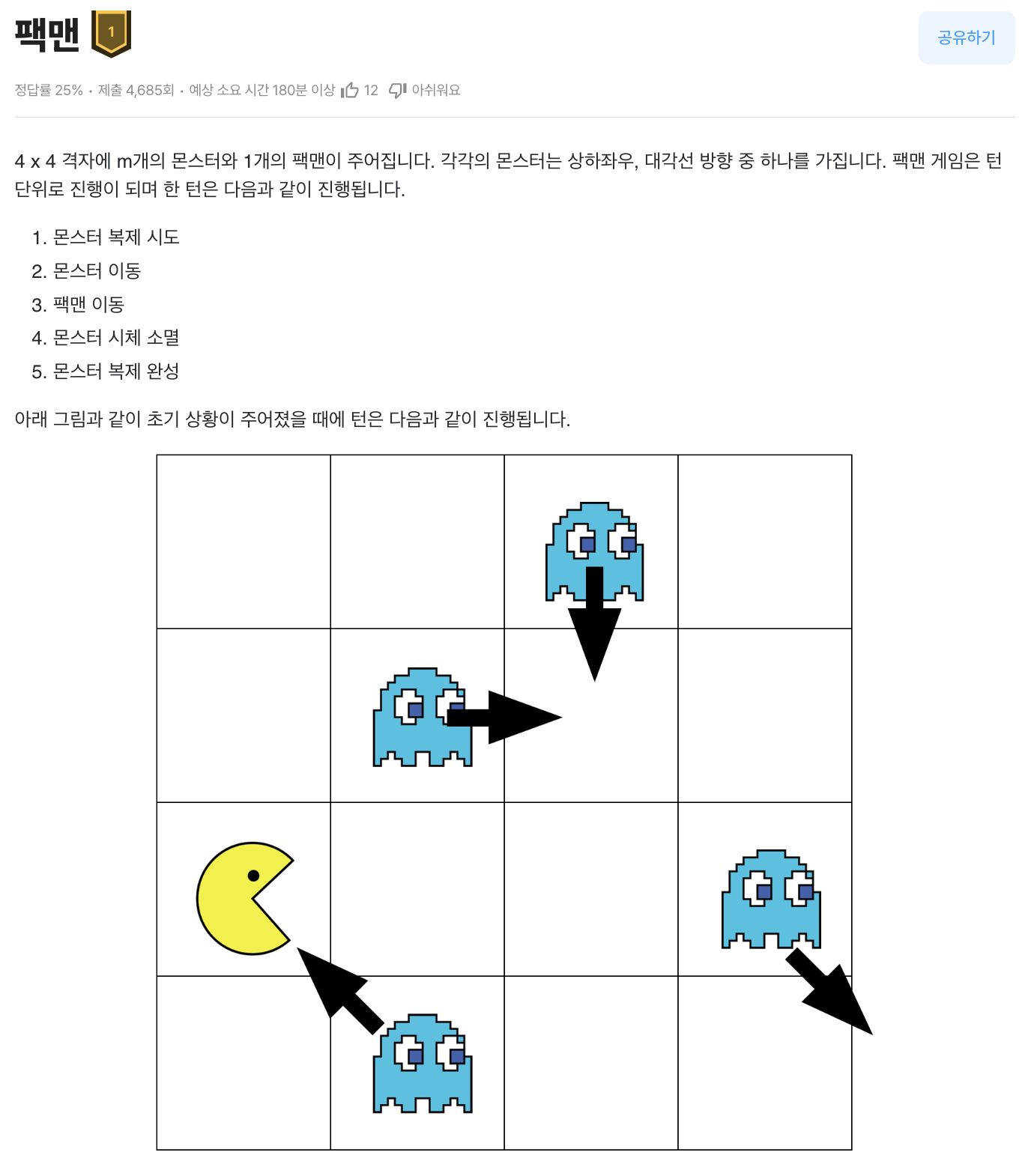
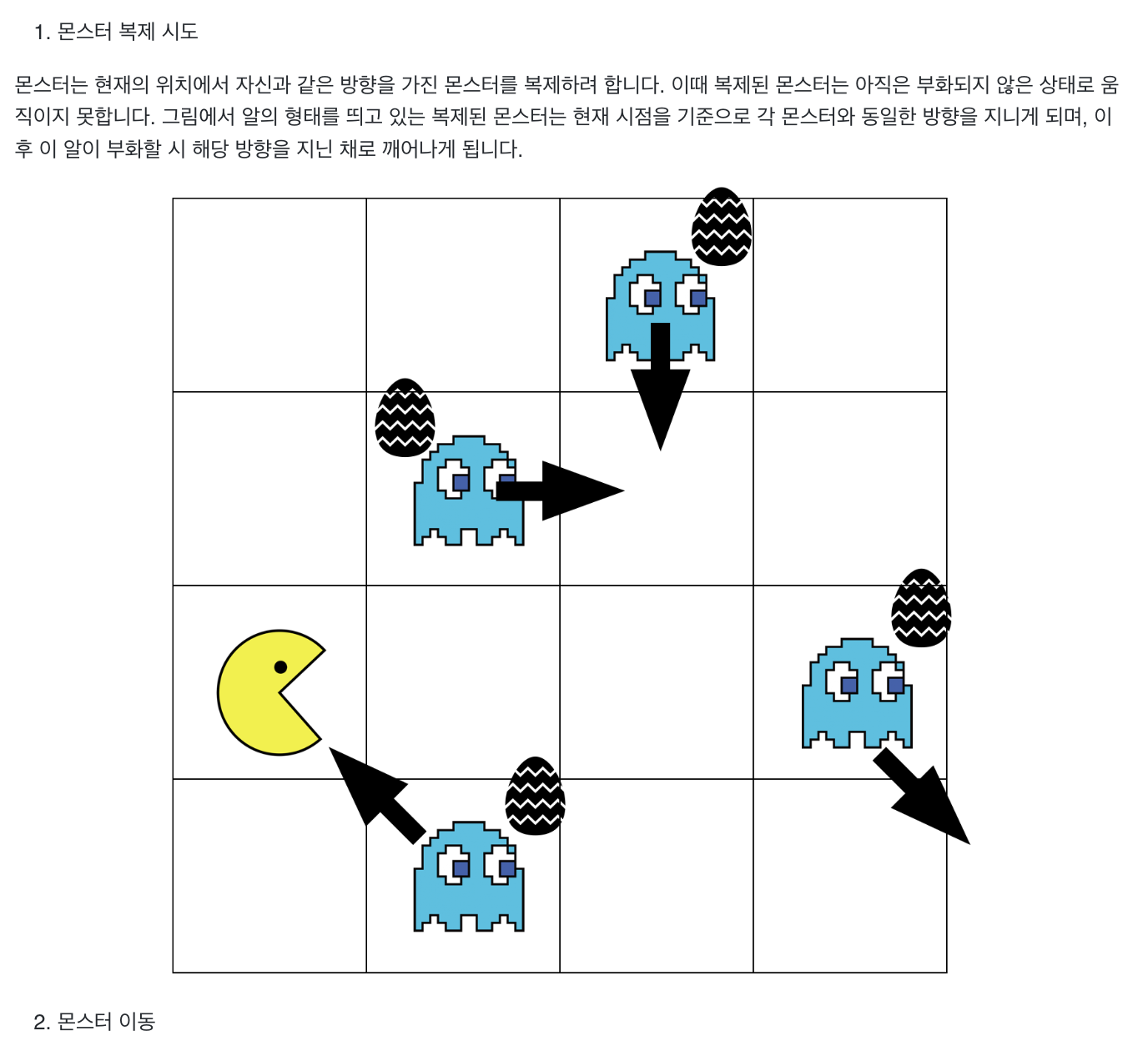
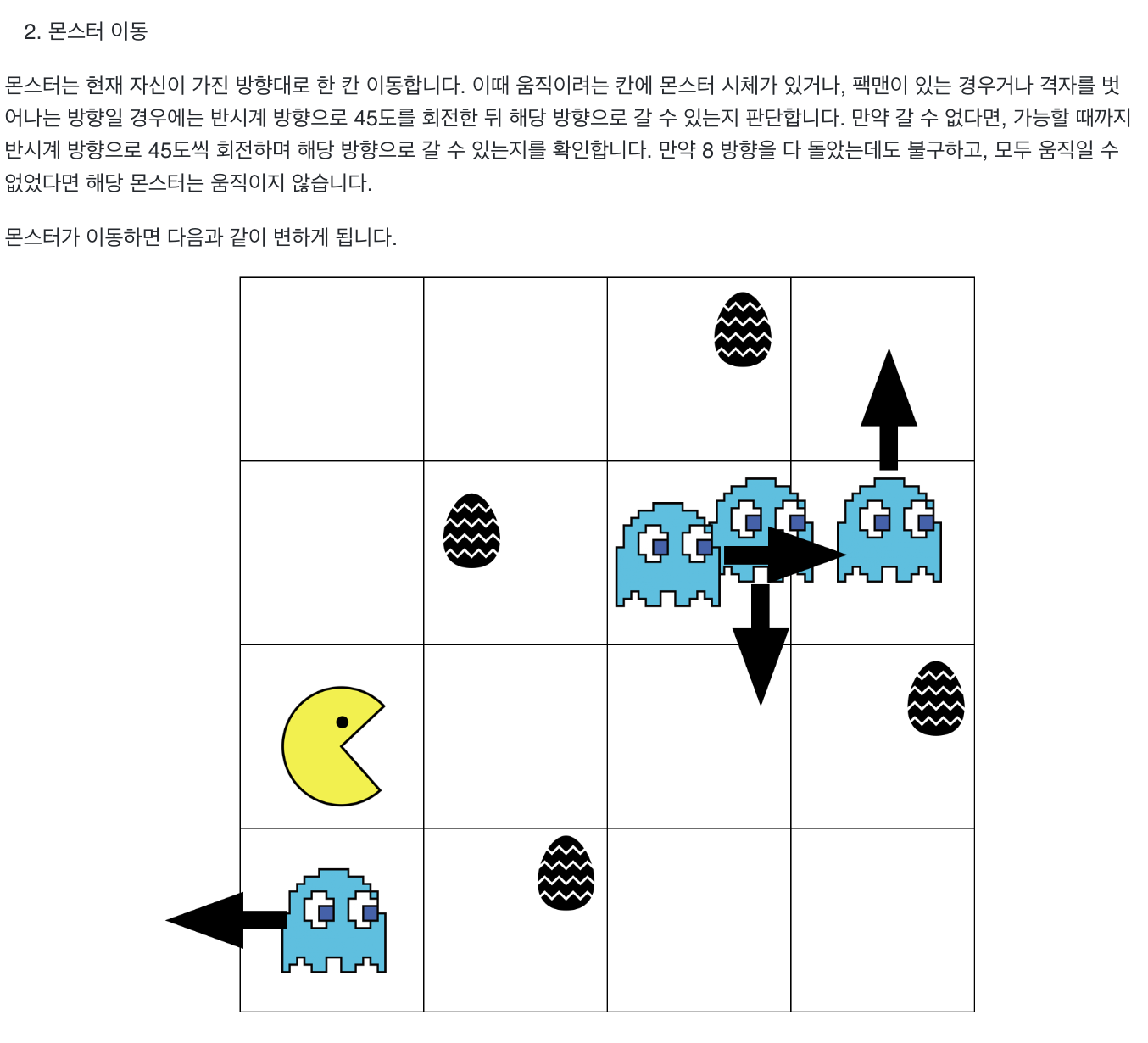
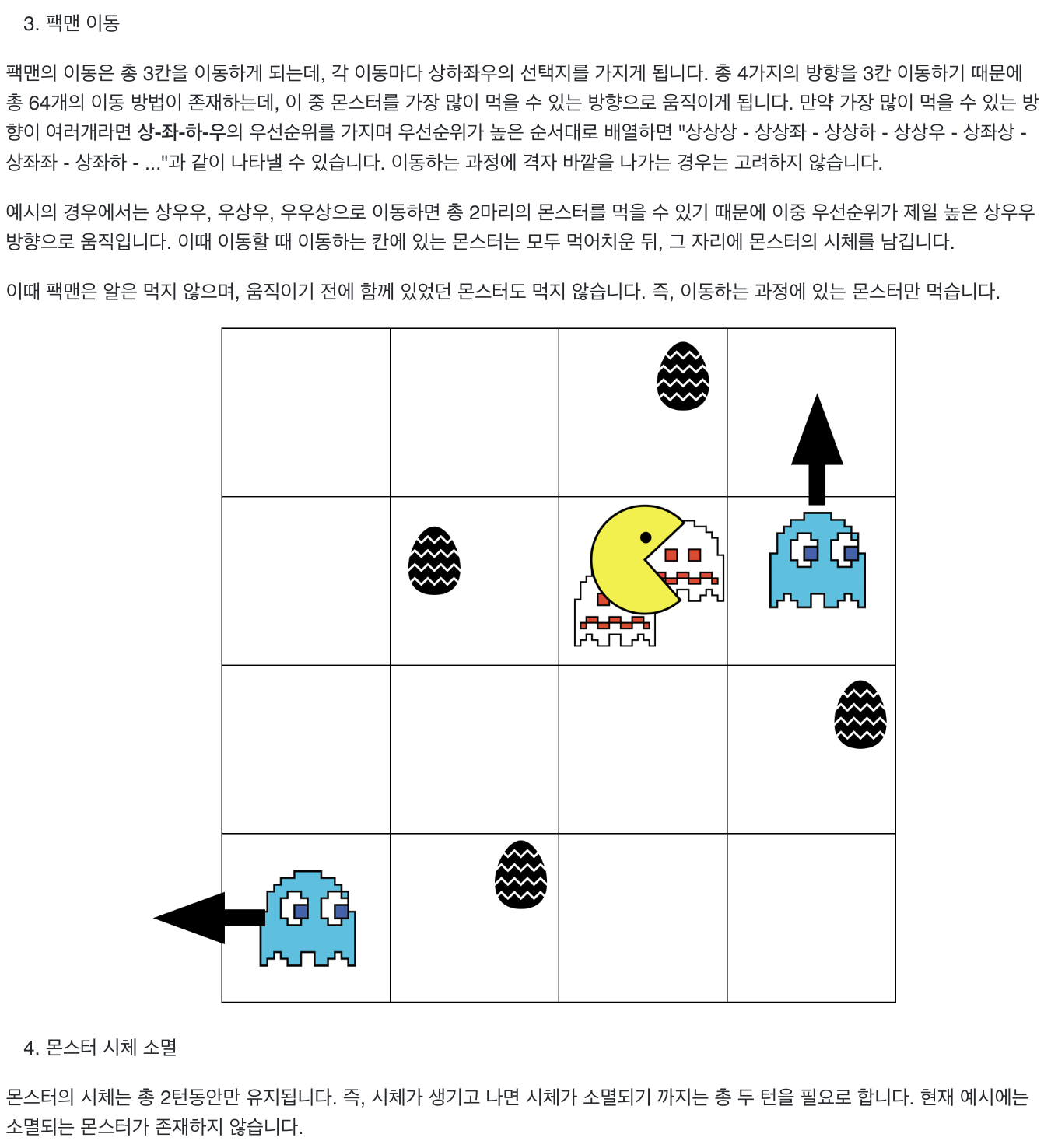
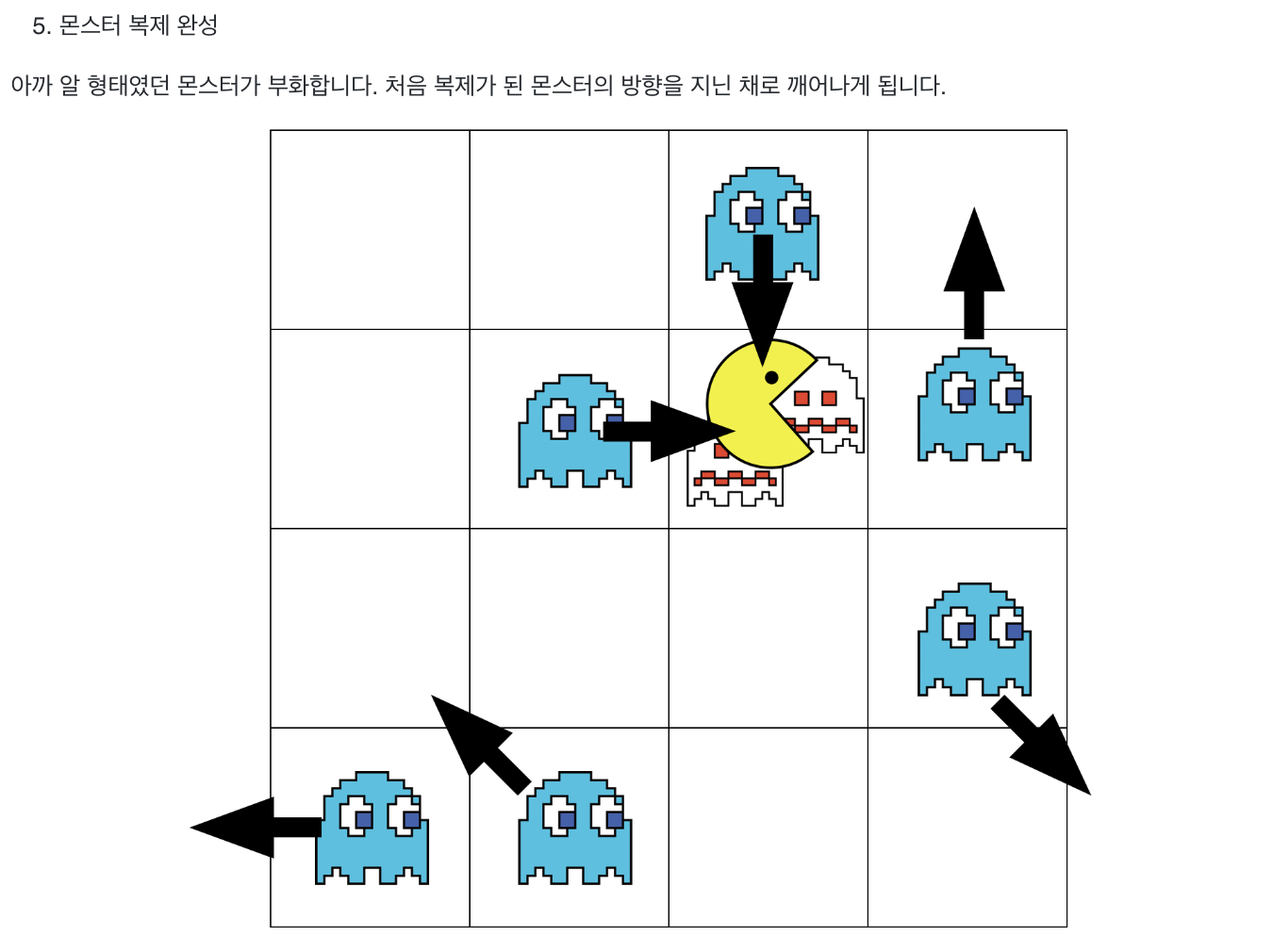
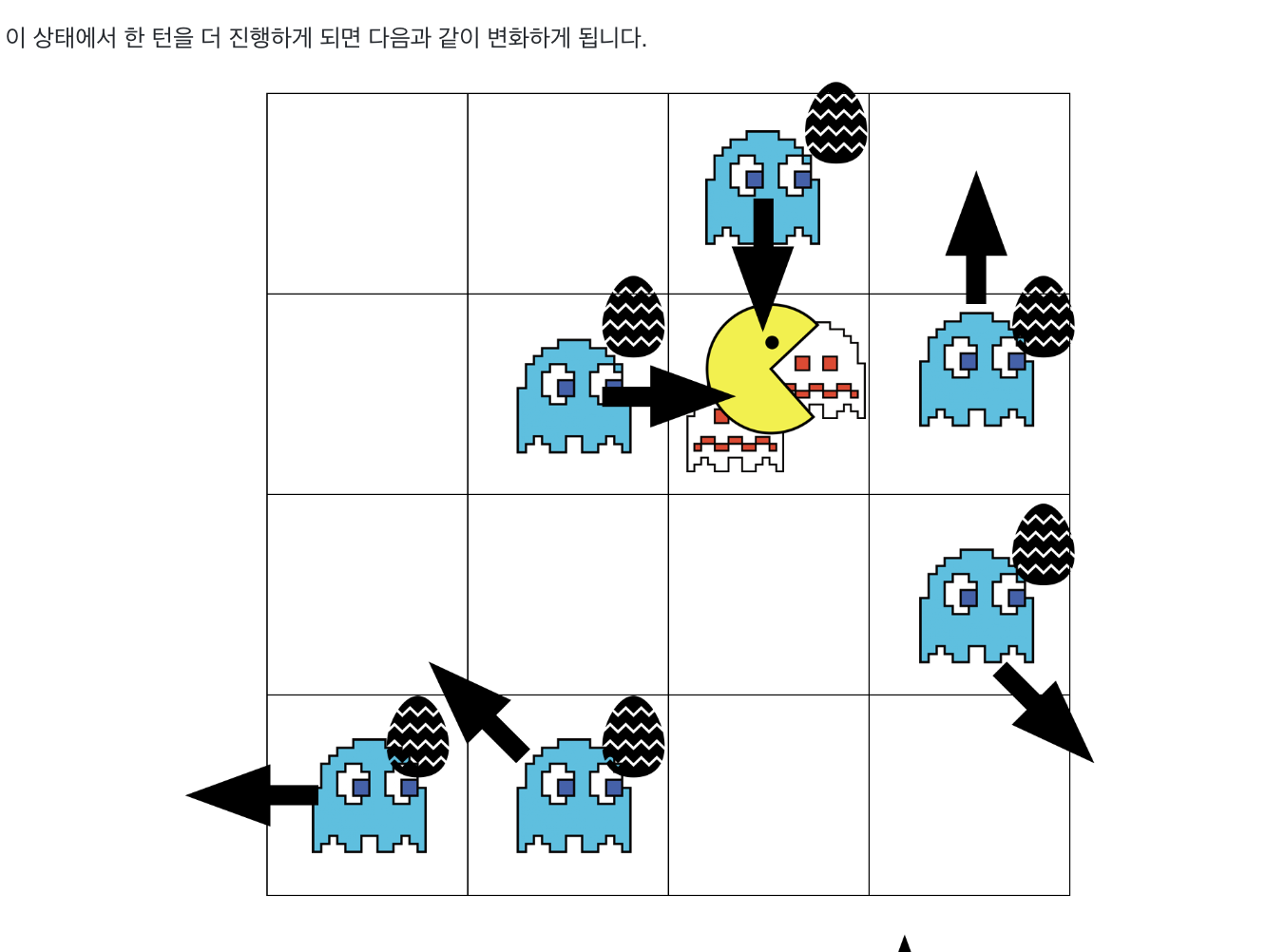
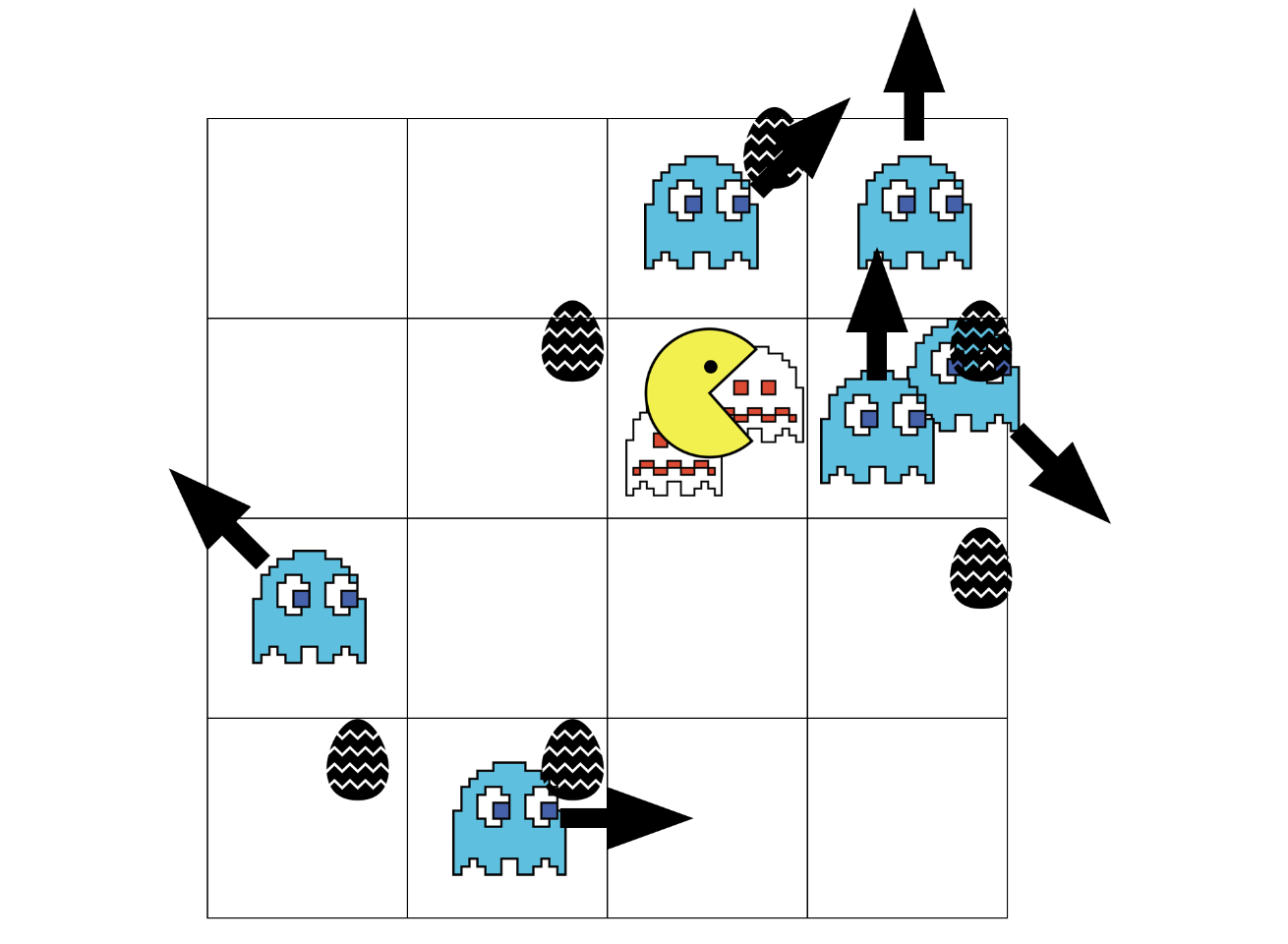
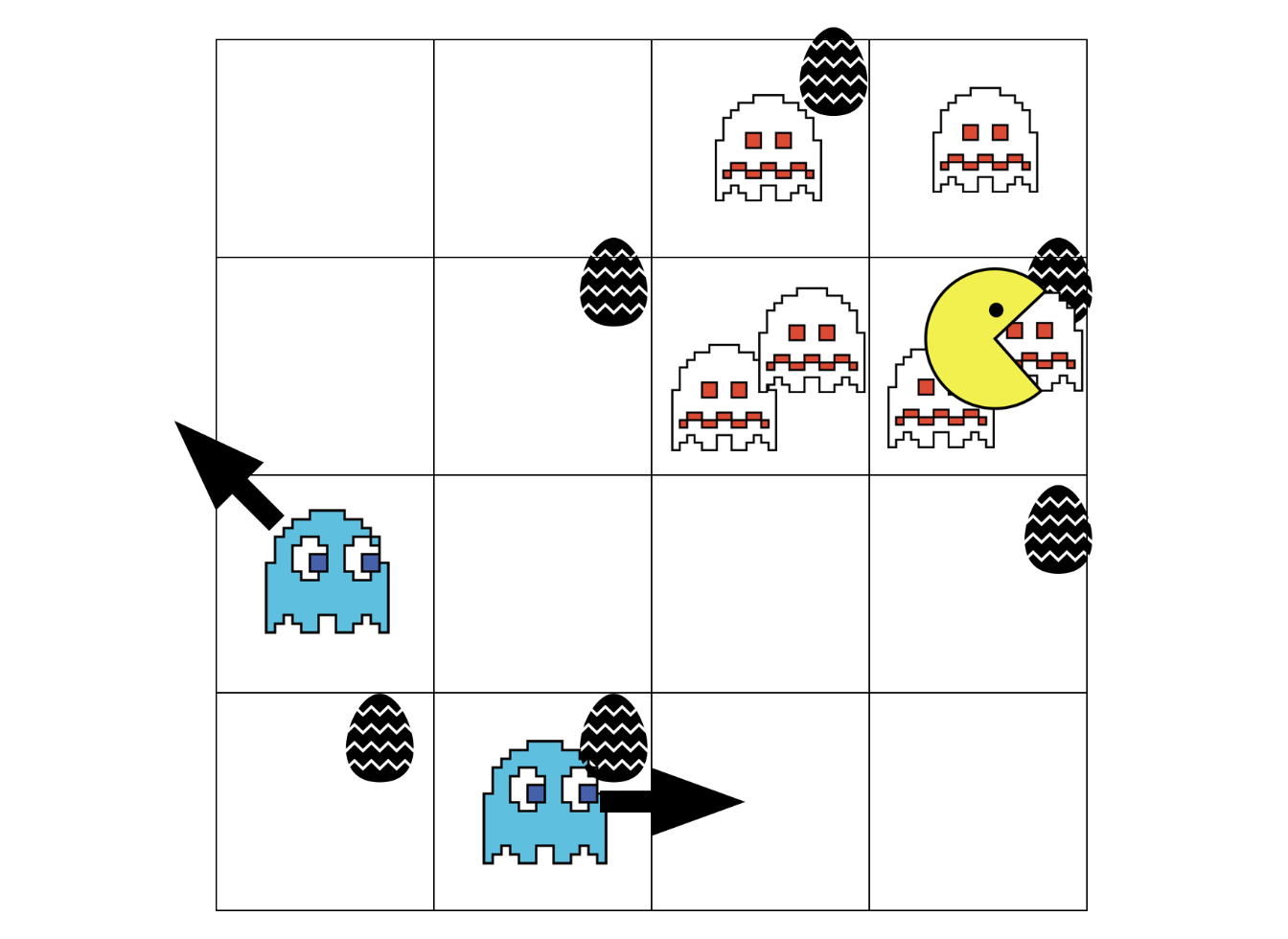
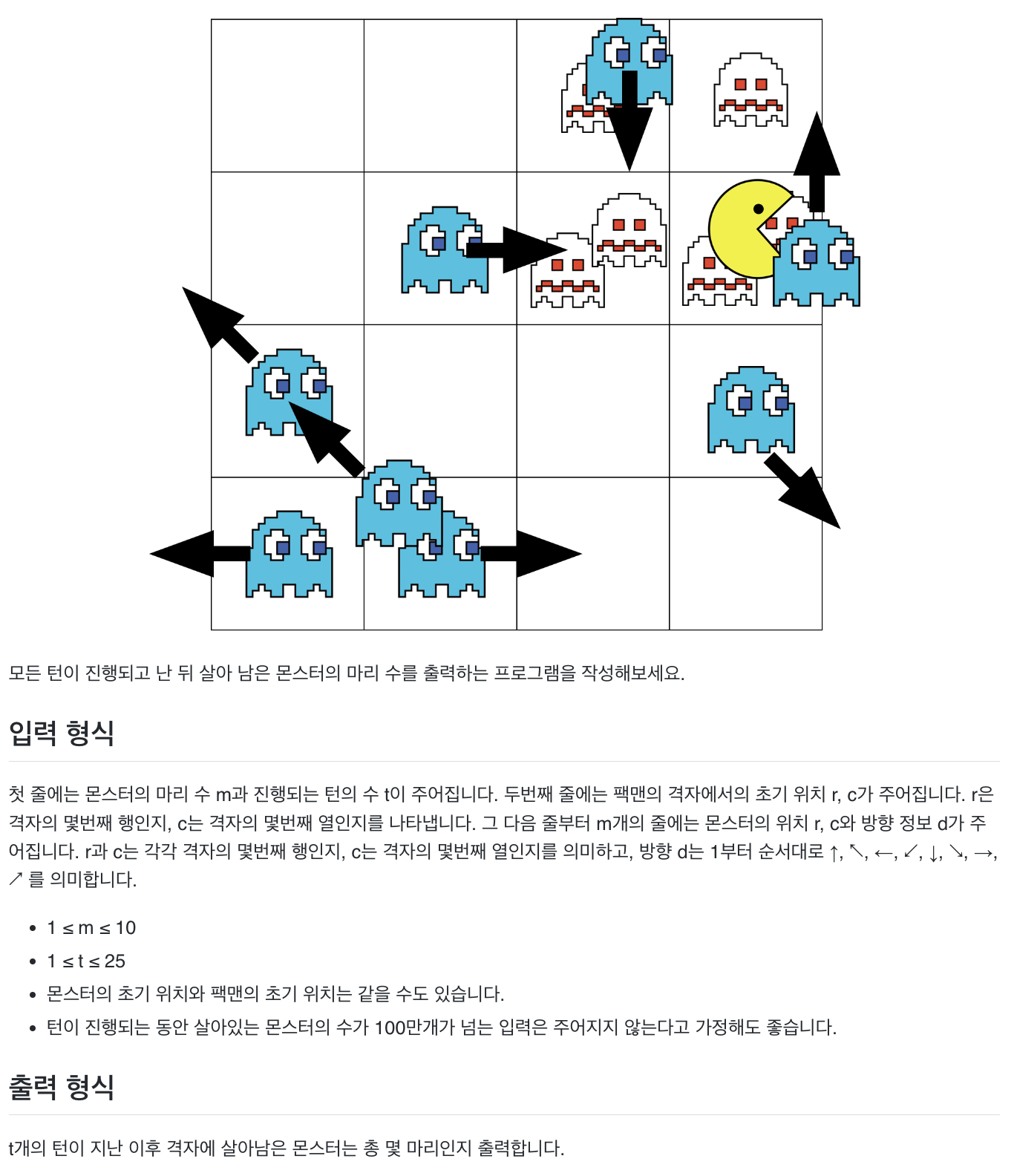
import sys
import copy
import heapq
input = sys.stdin.readline
pdx = (-1, 0, 1, 0)
pdy = (0, -1, 0, 1)
mdx = (-1, -1, 0, 1, 1, 1, 0, -1)
mdy = (0, -1, -1, -1, 0, 1, 1, 1)
M, T = map(int, input().split())
Pack = list(map(int, input().split())); Pack[0]-=1; Pack[1]-=1
Monster = [[[0 for _ in range(8)] for _ in range(4)] for _ in range(4)]
MonsterDead = [[0 for _ in range(4)] for _ in range(4)]
for m in range(M):
r, c, d = map(int, input().split()); r-=1; c-=1; d-=1
Monster[r][c][d] += 1
def MoveMonster():
global Monster
TMonster = [[[0 for _ in range(8)] for _ in range(4)] for _ in range(4)]
for x in range(4):
for y in range(4):
for d in range(8):
if Monster[x][y][d] > 0:
nx, ny, nd = CheckLocation(x, y, d)
TMonster[nx][ny][nd] += Monster[x][y][d]
Monster = copy.deepcopy(TMonster)
def CheckLocation(x, y, d):
global MonsterDead, Pack
cnt = 0
while cnt < 8:
nx, ny = x+mdx[d], y+mdy[d]
if 0<=nx<4 and 0<=ny<4 and MonsterDead[nx][ny] == 0 and (nx, ny) != (Pack[0], Pack[1]):
return nx, ny, d
else:
cnt += 1
d = (d+1)%8
return x, y, d
def MovePack(px, py):
heap = []
for first in range(4):
for second in range(4):
for third in range(4):
tcnt = 0; flag = True
x, y = px, py
visit = []
for d in [first, second, third]:
x += pdx[d]; y += pdy[d]
if 0<=x<4 and 0<=y<4:
if (x, y) not in visit:
tcnt += sum(Monster[x][y])
visit.append((x, y))
else: flag = False; break
if flag: heapq.heappush(heap, (-tcnt, first, second, third))
_, first, second, third = heapq.heappop(heap)
for d in [first, second, third]:
px += pdx[d]; py += pdy[d]
for i in range(8):
if Monster[px][py][i] > 0: MonsterDead[px][py] = 3; break
Monster[px][py] = [0 for _ in range(8)]
Pack[0] = px; Pack[1] = py
def MonsterMinusOne():
for x in range(4):
for y in range(4):
if MonsterDead[x][y] > 0:
MonsterDead[x][y] -= 1
def MonsterDuplicate():
for x in range(4):
for y in range(4):
for d in range(8):
Monster[x][y][d] += MonsterBackup[x][y][d]
for t in range(T):
MonsterBackup = copy.deepcopy(Monster)
MoveMonster()
MovePack(Pack[0], Pack[1])
MonsterMinusOne()
MonsterDuplicate()
ans = 0
for x in range(4):
for y in range(4):
ans += sum(Monster[x][y])
print(ans)