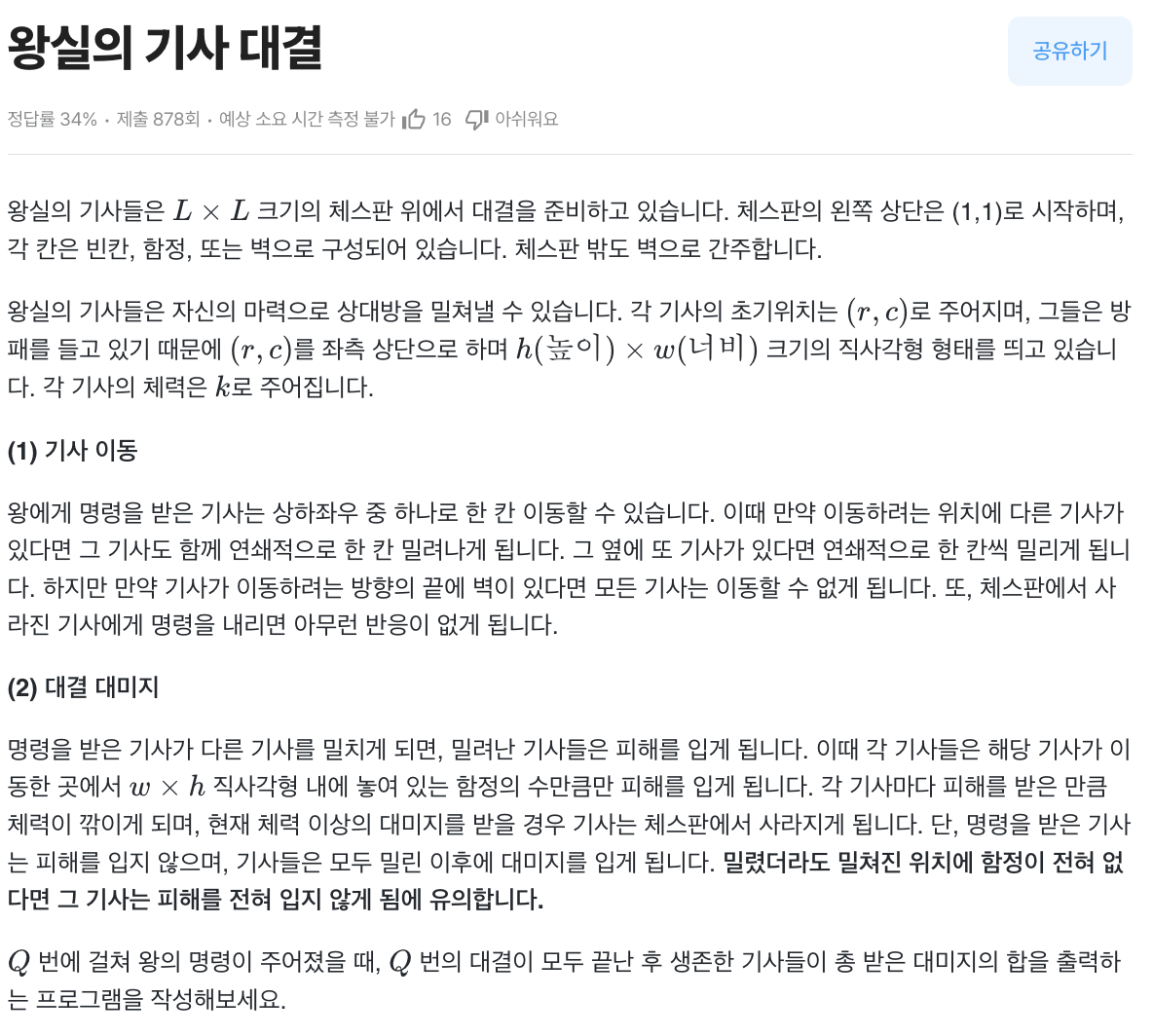
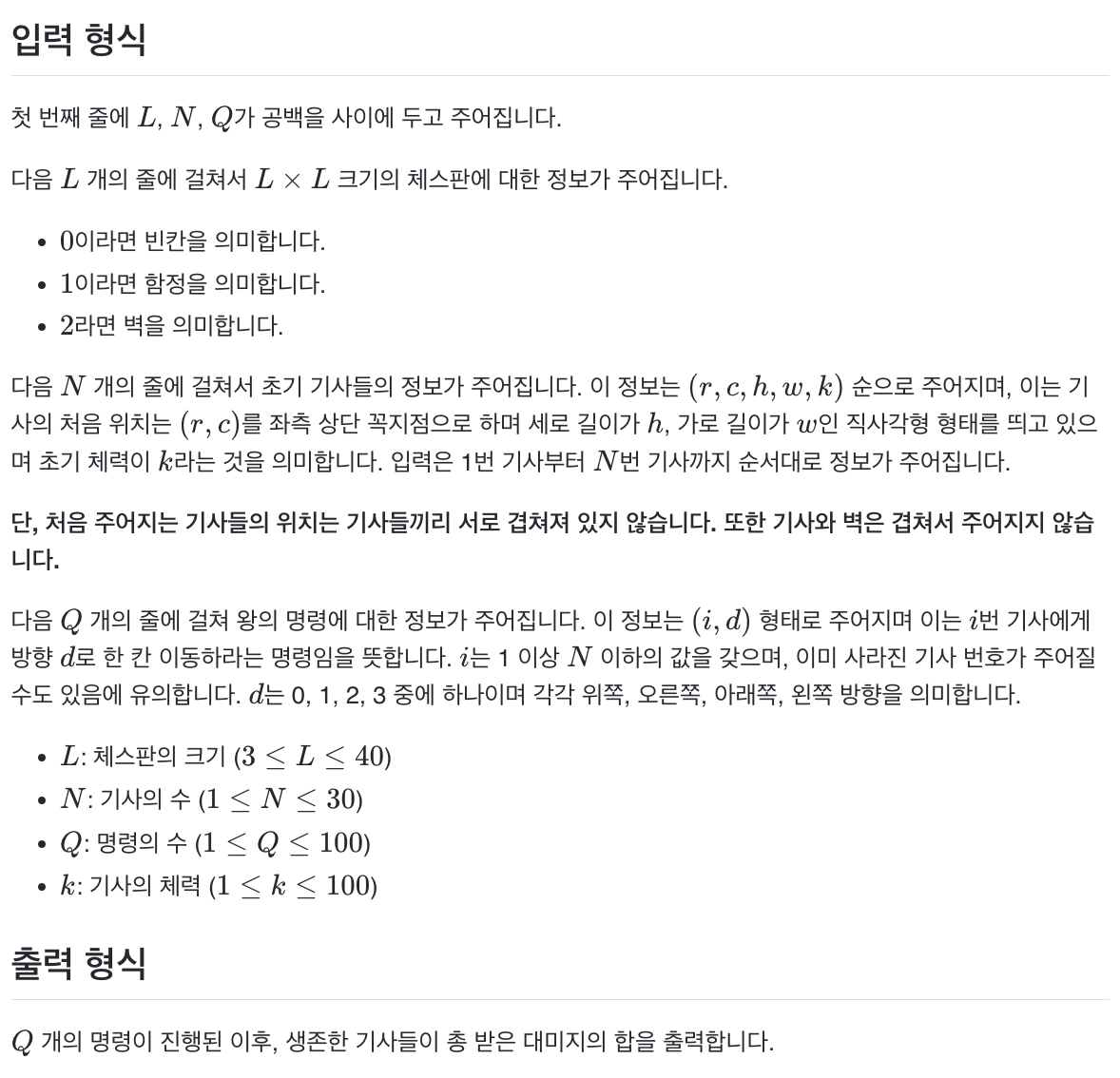
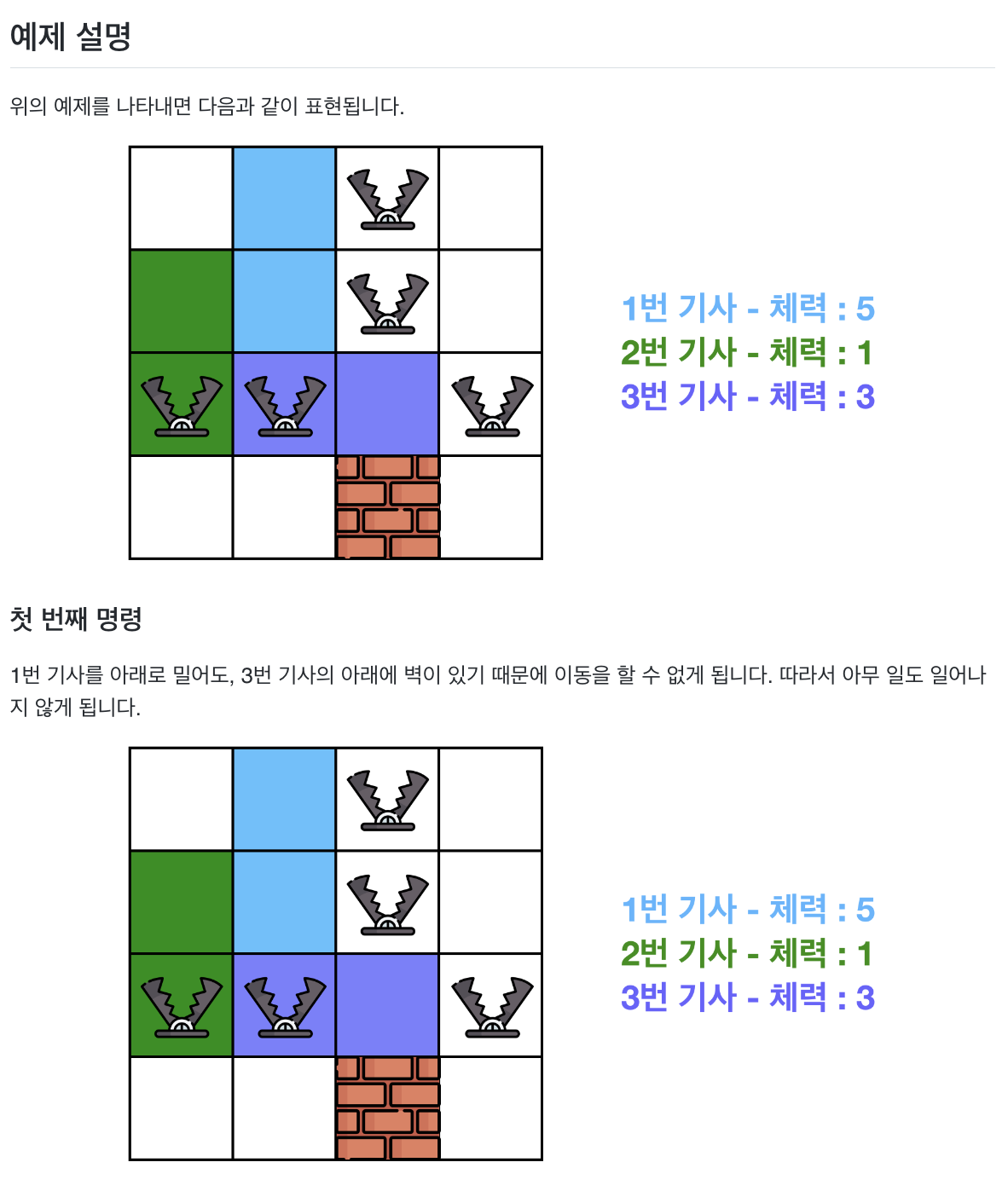
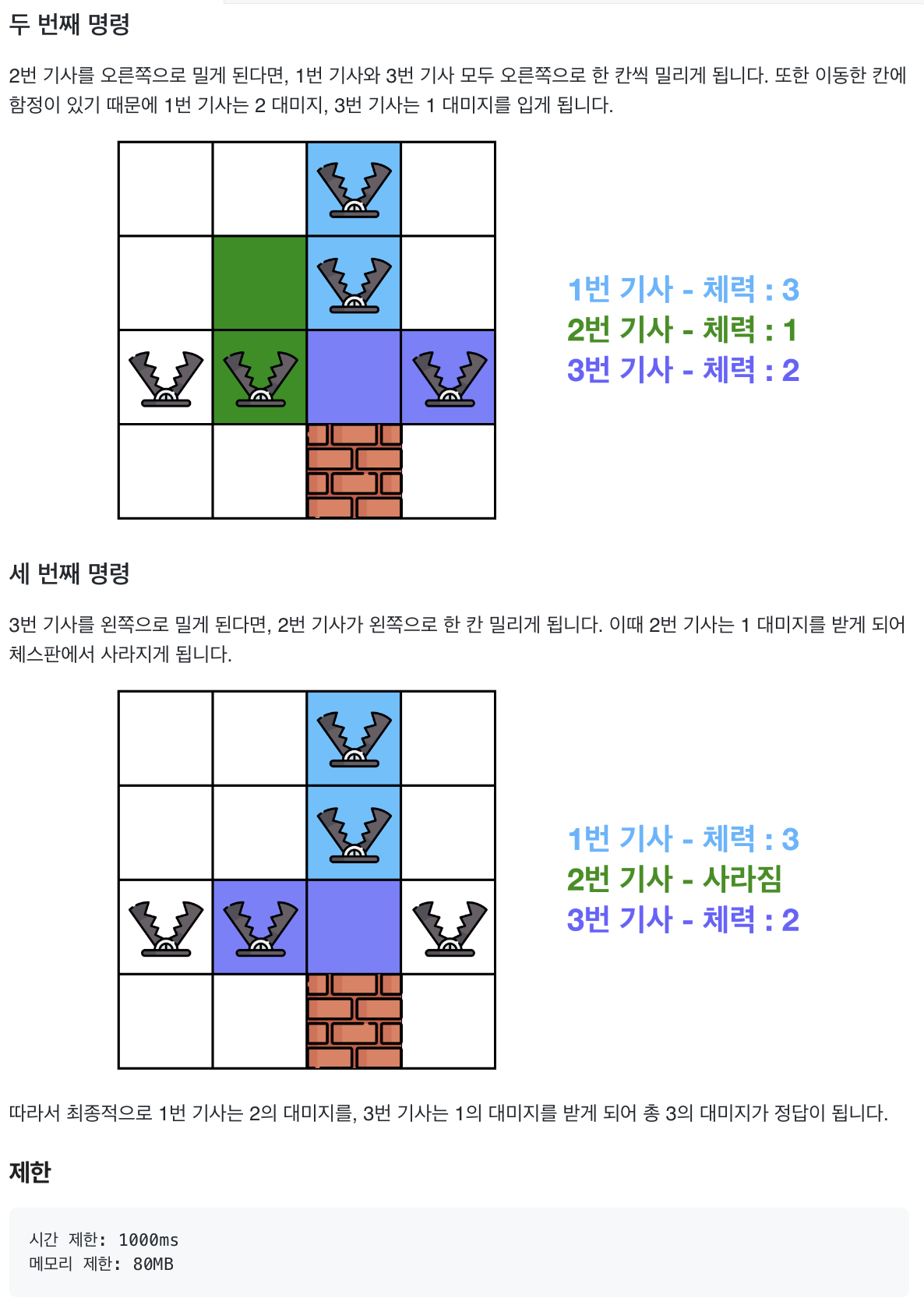
import sys
import copy
input = sys.stdin.readline
dx = (-1, 0, 1, 0)
dy = (0, 1, 0, -1)
L, N, Q = map(int, input().split())
board = [list(map(int, input().split())) for l in range(L)]
stat = [0]
for n in range(N):
r, c, h, w, k = list(map(int, input().split()))
r-=1; c-=1; stat.append([r, c, h, w, k])
k_backup = [0]
for n in range(1, N+1): k_backup.append(stat[n][4])
def isPossible(i, d):
global simul_result
r, c, h, w, _ = stat[i]
if d == 0:
nr = r-1
if 0<=nr<L:
for nc in range(c, c+w):
if board[nr][nc] != 2:
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
if simul_result: isPossible(n, d)
else: simul_result = False; return
else: simul_result = False; return
elif d == 1:
nc = c+w
if 0<=nc<L:
for nr in range(r, r+h):
if board[nr][nc] != 2:
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
if simul_result: isPossible(n, d)
else: simul_result = False; return
else: simul_result = False; return
elif d == 2:
nr = r+h
if 0<=nr<L:
for nc in range(c, c+w):
if board[nr][nc] != 2:
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
if simul_result: isPossible(n, d)
else: simul_result = False; return
else: simul_result = False; return
else:
nc = c-1
if 0<=nc<L:
for nr in range(r, r+h):
if board[nr][nc] != 2:
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
if simul_result: isPossible(n, d)
else: simul_result = False; return
else: simul_result = False; return
def doThat(first, i, d):
global will_moved
r, c, h, w, _ = stat[i]
if d == 0:
nr = r-1
for nc in range(c, c+w):
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
doThat(first, n, d); will_moved.add(n)
elif d == 1:
nc = c+w
for nr in range(r, r+h):
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
doThat(first, n, d); will_moved.add(n)
elif d == 2:
nr = r+h
for nc in range(c, c+w):
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
doThat(first, n, d); will_moved.add(n)
else:
nc = c-1
for nr in range(r, r+h):
for n in range(1, N+1):
if n != i and stat[n] != 0:
rr, cc, hh, ww, __ = stat[n]
if rr <= nr < rr+hh and cc <= nc < cc+ww:
doThat(first, n, d); will_moved.add(n)
def reDraw(target, d):
global will_moved
for n in range(1, N+1):
if stat[n] != 0:
if n in will_moved:
if d == 0: stat[n][0] -= 1
elif d == 1: stat[n][1] += 1
elif d == 2: stat[n][0] += 1
else: stat[n][1] -= 1
for n in will_moved:
if stat[n] != 0 and n != target:
r, c, h, w, k = stat[n]
cnt = 0
for nr in range(r, r+h):
for nc in range(c, c+w):
if board[nr][nc] == 1: cnt += 1
stat[n][4] -= cnt
if stat[n][4] <= 0: stat[n] = 0
for q in range(Q):
i, d = map(int, input().split())
if stat[i] == 0: continue
simul_result = True
isPossible(i, d)
if simul_result:
will_moved = set()
doThat(i, i, d)
will_moved.add(i)
reDraw(i, d)
total = 0
for n in range(1, N+1):
if stat[n] != 0: total += k_backup[n] - stat[n][4]
print(total)