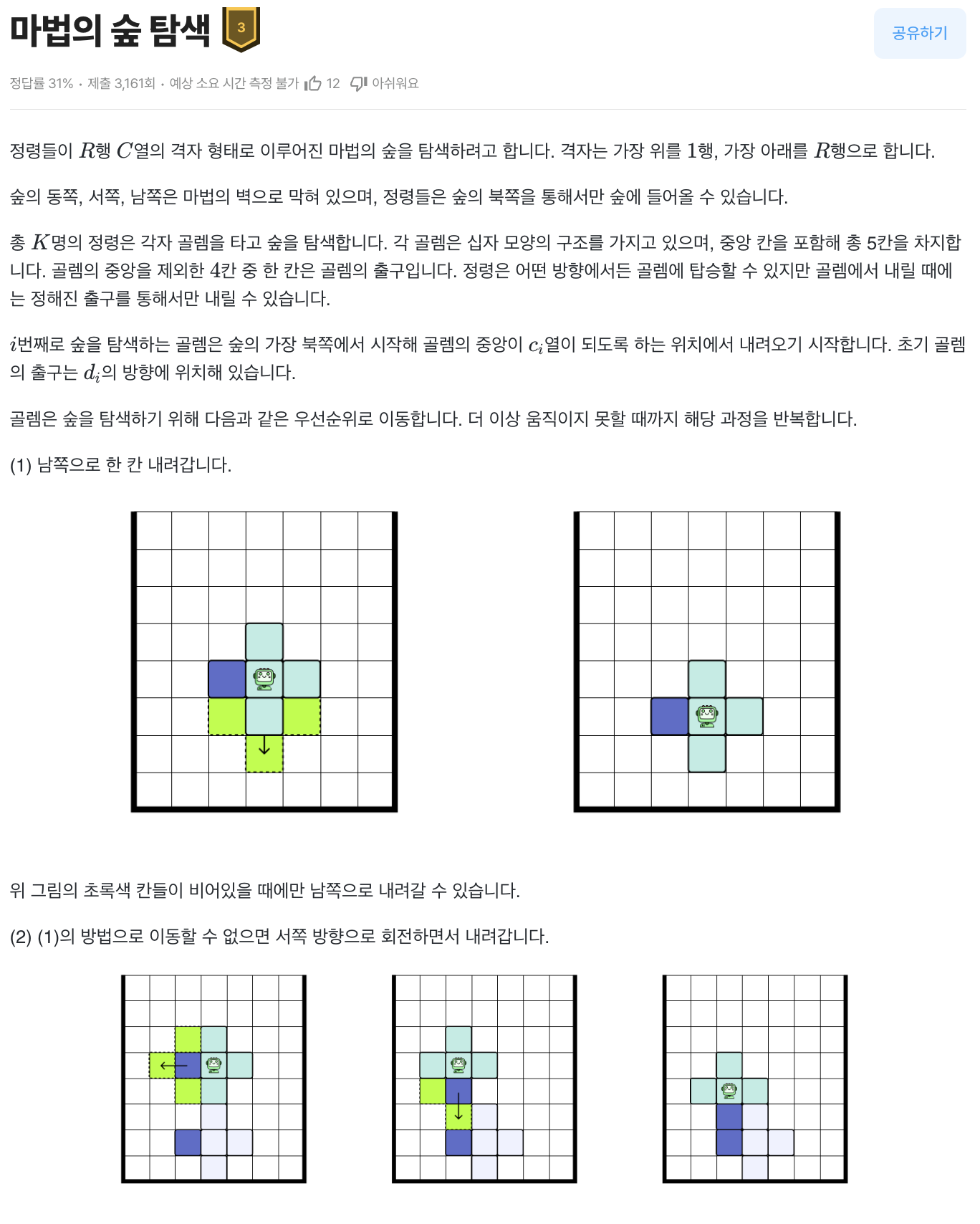
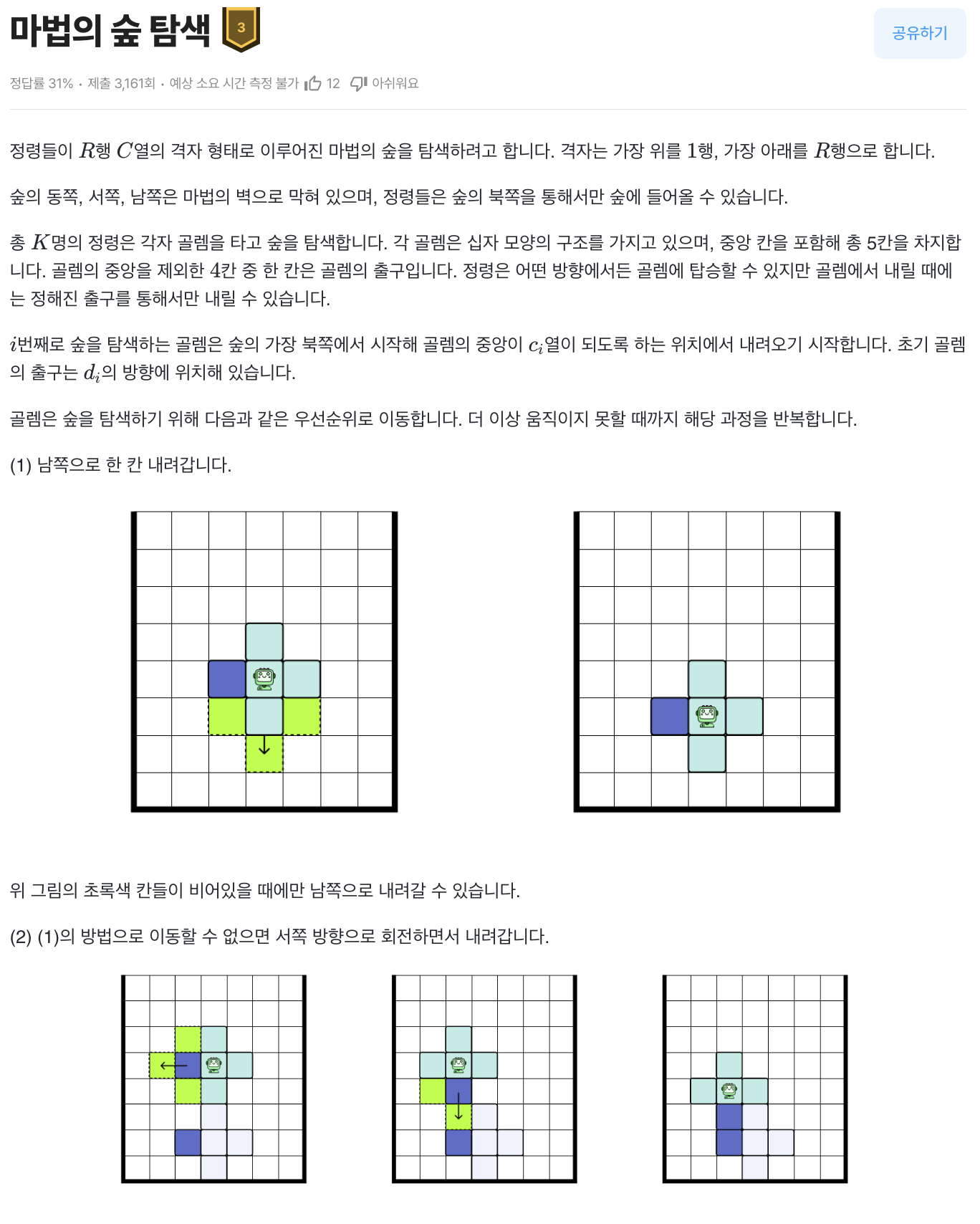
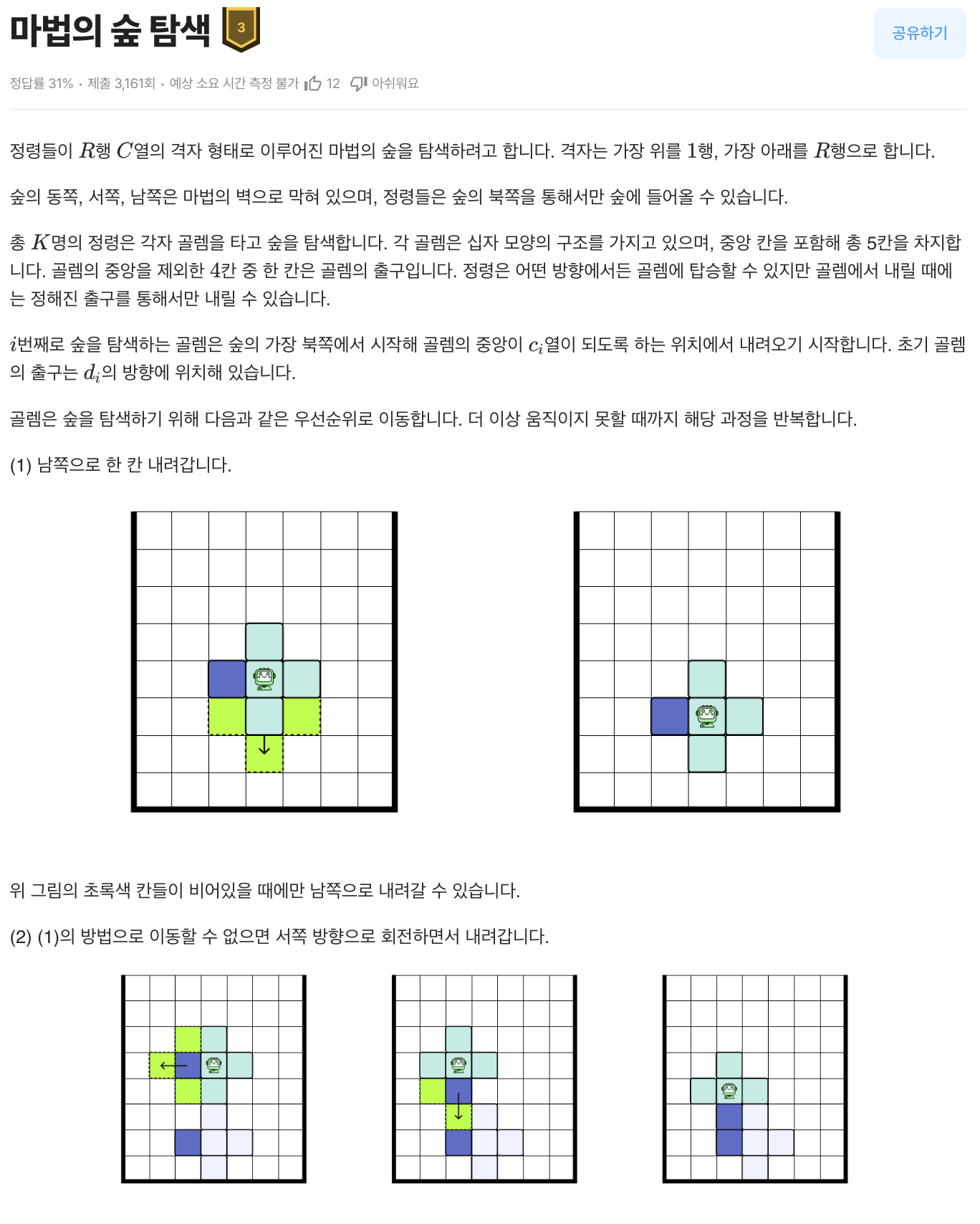
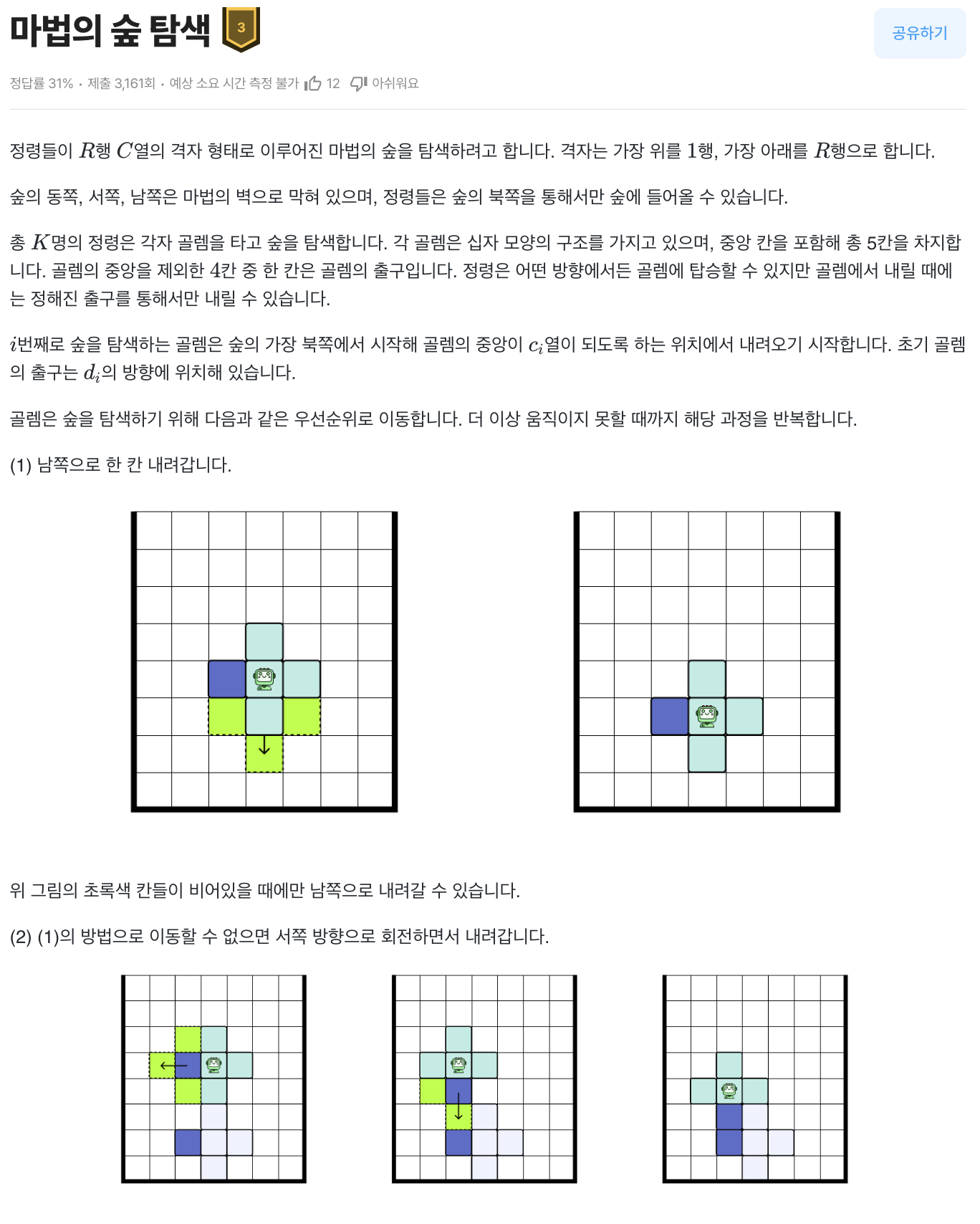
import sys
input = sys.stdin.readline
R, C, K = map(int, input().split())
board = [[0]*C for r in range(R)]
dx = (-1, 0, 1, 0)
dy = (0, 1, 0, -1)
def MoveGolem(Col, D):
x, y = -2, Col
while True:
flag = False
if x == -2:
if board[x+2][y] == 0:
x, y = x+1, y; flag = True
else:
if board[x+1][y-1] == 0 and board[x+2][y] == 0 and board[x+1][y+1] == 0:
x, y = x+1, y; flag = True
if not flag:
if y >= 2:
if x == -2:
if board[x+2][y-1] == 0:
x, y = x+1, y-1; flag = True
D = (D+3)%4
elif x == -1:
if board[x+1][y-1] == 0 and board[x+1][y-2] == 0 and board[x+2][y-1] == 0:
x, y = x+1, y-1; flag = True
D = (D+3)%4
elif x == 0:
if board[x][y-2] == 0 and board[x+1][y-1] == 0 and board[x+1][y-2] == 0 and board[x+2][y-1] == 0:
x, y = x+1, y-1; flag = True
D = (D+3)%4
else:
if board[x-1][y-1] == 0 and board[x][y-2] == 0 and board[x+1][y-1] == 0 and board[x+1][y-2] == 0 and board[x+2][y-1] == 0:
x, y = x+1, y-1; flag = True
D = (D+3)%4
if not flag:
if y <= C-3:
if x == -2:
if board[x+2][y+1] == 0:
x, y = x+1, y+1; flag = True
D = (D+1)%4
elif x == -1:
if board[x+1][y+1] == 0 and board[x+1][y+2] == 0 and board[x+2][y+1] == 0:
x, y = x+1, y+1; flag = True
D = (D+1)%4
elif x == 0:
if board[x][y+2] == 0 and board[x+1][y+1] == 0 and board[x+1][y+2] == 0 and board[x+2][y+1] == 0:
x, y = x+1, y+1; flag = True
D = (D+1)%4
else:
if board[x-1][y+1] == 0 and board[x][y+2] == 0 and board[x+1][y+1] == 0 and board[x+1][y+2] == 0 and board[x+2][y+1] == 0:
x, y = x+1, y+1; flag = True
D = (D+1)%4
if not flag or x == R-1-1: break
return x, y, D
def GetScore(x, y, color):
global max_x, exit
max_x = max(max_x, x)
for i in range(4):
nx, ny = x+dx[i], y+dy[i]
if 0<=nx<R and 0<=ny<C:
if not visit[nx][ny] and board[nx][ny] != 0:
if exit[color] == (x, y):
visit[nx][ny] = True
GetScore(nx, ny, board[nx][ny])
else:
if board[nx][ny] == color:
visit[nx][ny] = True
GetScore(nx, ny, board[nx][ny])
ans = 0
exit = dict()
for k in range(1, K+1):
Col, Dir = map(int, input().split()); Col -= 1
rx, ry, rdir = MoveGolem(Col, Dir)
if rx < 1:
board = [[0]*C for r in range(R)]
else:
board[rx-1][ry] = k
board[rx][ry-1] = k
board[rx][ry] = k
board[rx][ry+1] = k
board[rx+1][ry] = k
exit[k] = (rx+dx[rdir], ry+dy[rdir])
max_x = -2
visit = [[False]*C for r in range(R)]; visit[rx][ry] = True
GetScore(rx, ry, k)
max_x += 1
ans += max_x
print(ans)