1.문제
You are given coordinates, a string that represents the coordinates of a square of the chessboard. Below is a chessboard for your reference.
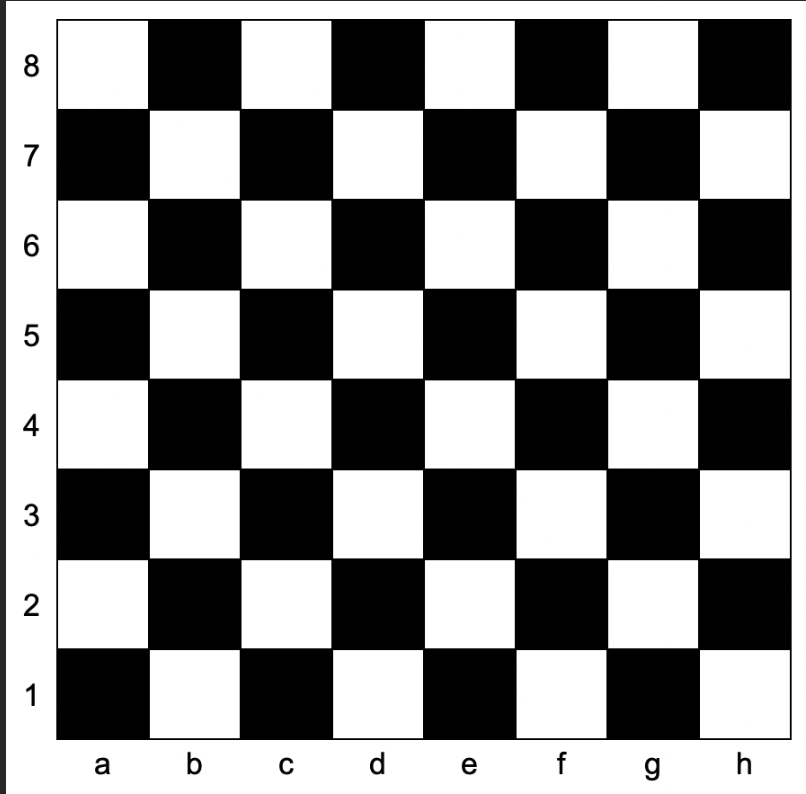
Return true if the square is white, and false if the square is black.
The coordinate will always represent a valid chessboard square. The coordinate will always have the letter first, and the number second.
체크판의 위치인 coordinates가 주어질 때 주어진 체크판의 위치가 흰색이면 true , 검은색이면 false를 리턴하는 문제이다.
Example 1
Input: coordinates = "a1"
Output: false
Explanation: From the chessboard above, the square with coordinates "a1" is black, so return false.
Example 2
Input: coordinates = "h3"
Output: true
Explanation: From the chessboard above, the square with coordinates "h3" is white, so return true.
Example 3
Input: coordinates = "c7"
Output: false
Constraints:
- coordinates.length == 2
- 'a' <= coordinates[0] <= 'h'
- '1' <= coordinates[1] <= '8'
2.풀이
- coordinates의 알파벳 부분을 a : 1 ~ h : 8 로 변환한다.
- 두 좌표의 합이 짝수이면 흰색 , 홀수이면 검은색이다.
/**
* @param {string} coordinates
* @return {boolean}
*/
const squareIsWhite = function (coordinates) {
// coordinates 의 알파벳을 a부터 1로 치환하여 숫자 부분이랑 더한 값이 짝수이면 검은색 , 홀수이면 하얀색이다.
return (coordinates[0].charCodeAt() - 96 + parseInt(coordinates[1])) % 2 === 0
? false
: true;
};
3.결과
