import cv2
import numpy as np
img = cv2.imread('lett.jpg')
def mousehandler(event, x, y, flags, param):
if event == cv2.EVENT_LBUTTONDOWN:
print(x, y)
cv2.namedWindow('img')
cv2.setMouseCallback('img', mousehandler)
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
import cv2
import numpy as np
points = []
color = (0, 255, 255)
img = cv2.imread('lett.jpg')
def mousehandler(event, x, y, flags, param):
if event == cv2.EVENT_LBUTTONDOWN:
points.append((x, y))
for point in points:
cv2.circle(img, point, 5, color, cv2.FILLED)
if len(points) == 4:
show_result()
cv2.imshow('img', img)
def show_result():
width, height = 530, 710
src = np.float32(points)
dst = np.array([[0, 0], [width, 0], [width, height], [0, height]], dtype = np.float32)
matrix = cv2.getPerspectiveTransform(src, dst)
result = cv2.warpPerspective(img, matrix, (width, height))
cv2.imshow('result', result)
cv2.namedWindow('img')
cv2.setMouseCallback('img', mousehandler)
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
라인 그려 이미지 추출하기
import cv2
import numpy as np
points = []
color = (0, 255, 255)
thickness = 3
drawing = False
img = cv2.imread('lett.jpg')
def mousehandler(event, x, y, flags, param):
global drawing
img2 = img.copy()
if event == cv2.EVENT_LBUTTONDOWN:
drawing = True
points.append((x, y))
if drawing:
prev_point = None
for point in points:
cv2.circle(img2, point, 5, color, cv2.FILLED)
if prev_point:
cv2.line(img2, prev_point, point, color, thickness, cv2.LINE_AA)
prev_point = point
next_point = (x, y)
if len(points) == 4:
show_result()
next_point = points[0]
cv2.line(img2, prev_point, next_point, color, thickness, cv2.LINE_AA)
cv2.imshow('img', img2)
def show_result():
width, height = 530, 710
src = np.float32(points)
dst = np.array([[0, 0], [width, 0], [width, height], [0, height]], dtype = np.float32)
matrix = cv2.getPerspectiveTransform(src, dst)
result = cv2.warpPerspective(img, matrix, (width, height))
cv2.imshow('result', result)
cv2.namedWindow('img')
cv2.setMouseCallback('img', mousehandler)
cv2.imshow('img', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
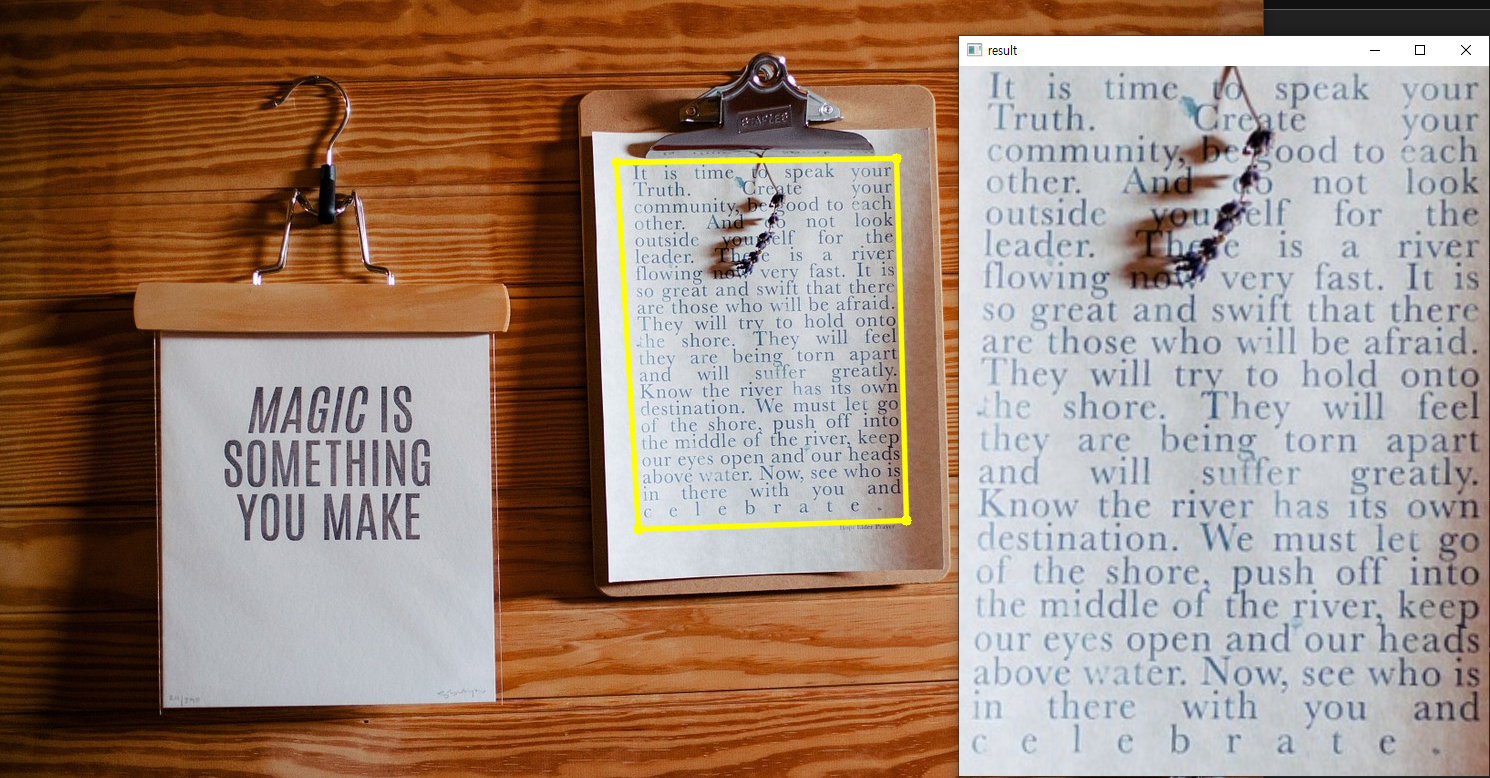