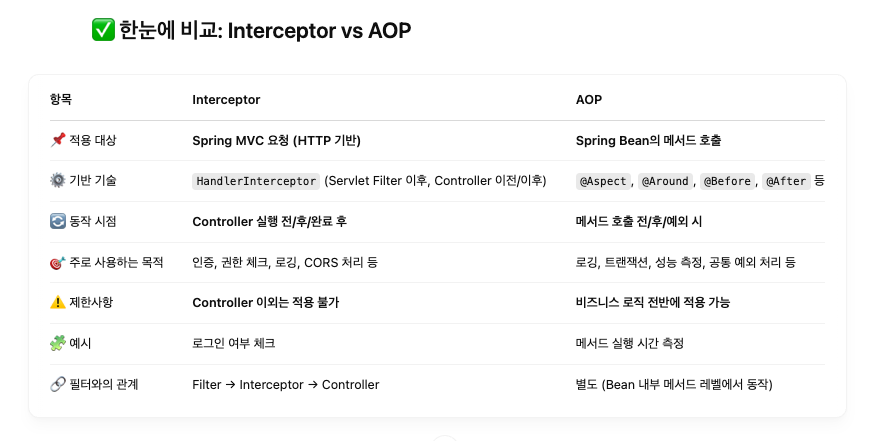
Interceptor
@Slf4j
@Component
public class LoggingInterceptor implements HandlerInterceptor {
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) {
log.info("요청 시작: {} {}", request.getMethod(), request.getRequestURI());
return true;
}
@Override
public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex) {
log.info("요청 완료: status = {}", response.getStatus());
}
}
- 주로 HTTP 요청/응답 레벨에서의 처리가 필요할 때 사용
- ex) 인증 체크, API 로깅, CORS
AOP 구조
@Aspect
@Component
@Slf4j
public class LoggingAspect {
@Around("execution(* com.example.service..*(..))")
public Object logExecutionTime(ProceedingJoinPoint joinPoint) throws Throwable {
long start = System.currentTimeMillis();
Object result = joinPoint.proceed();
long end = System.currentTimeMillis();
log.info("[{}] 실행 시간: {}ms", joinPoint.getSignature(), end - start);
return result;
}
}
- 주로 비즈니스 로직 내부에서의 공통 처리에 적합
- ex) 메서드 실행 시간 측정, 트랜잭션, 예외 감싸기
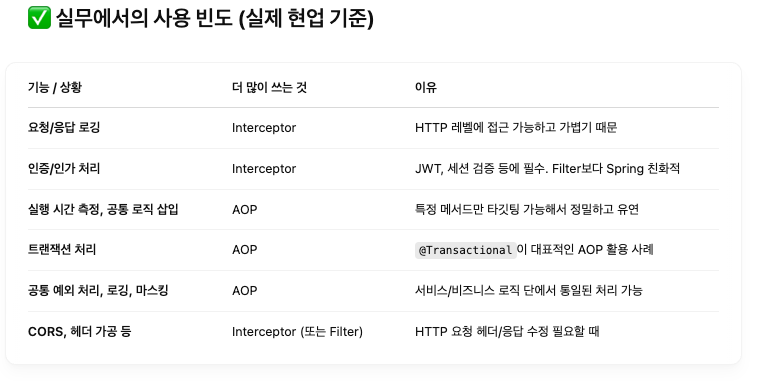
Interceptor + AOP
- Interceptor 로 API 요청을 기록하고,
- AOP 로 내부 서비스 메서드의 실행 시간도 측정하는 식으로 조합해서 사용할 수 있음