자바스크립트
가위바위보 규칙 찾기
- 배열.includes
- ||을 사용한 코드는 배열의 includes 메서드로 반복을 줄일 수 있다.
<html>
<head>
<meta charset="utf-8" />
<title>가위바위보</title>
<style>
#computer {
width: 142px;
height: 200px;
}
</style>
</head>
<body>
<div id="computer"></div>
<div>
<button id="scissors" class="btn">가위</button>
<button id="rock" class="btn">바위</button>
<button id="paper" class="btn">보</button>
</div>
<div id="score">0</div>
<script>
const $computer = document.querySelector('#computer');
const $score = document.querySelector('#score');
const $rock = document.querySelector('#rock');
const $scissors = document.querySelector('#scissors');
const $paper = document.querySelector('#paper');
const IMG_URL = './rsp.png';
$computer.style.background = `url(${IMG_URL}) 0 0`;
$computer.style.backgroundSize = 'auto 200px';
const rspX = {
scissors: '0',
rock: '-220px',
paper: '-440px',
};
let computerChoice = 'scissors';
const changeComputerHand = () => {
if (computerChoice === 'scissors') {
computerChoice = 'rock'
} else if (computerChoice === 'rock') {
computerChoice = 'paper'
} else if (computerChoice === 'paper') {
computerChoice = 'scissors'
}
$computer.style.background = `url(${IMG_URL}) ${rspX[computerChoice]} 0`;
$computer.style.backgroundSize = 'auto 200px';
}
let intervalId = setInterval(changeComputerHand, 50);
const scoreTable = {
rock: 0,
scissors: 1,
paper: -1,
};
let clickable = true;
let me = 0;
let computer = 0;
const clickButton = () => {
if (clickable) {
clearInterval(intervalId);
clickable = false;
const myChoice = event.target.textContent === '바위'
? 'rock'
: event.target.textContent === '가위'
? 'scissors'
: 'paper';
const myScore = scoreTable[myChoice];
const computerScore = scoreTable[computerChoice];
const diff = myScore - computerScore;
let message;
if ([2, -1].includes(diff)) {
me += 1;
message = '승리';
} else if ([-2, 1].includes(diff)) {
computer += 1;
message = '패배';
} else {
message = '무승부';
}
if (me === 3) {
$score.textContent = `나의 승리 ${me}:${computer}`;
} else if (computer === 3) {
$score.textContent = `컴퓨터의 승리 ${me}:${computer}`;
} else {
$score.textContent = `${message} ${me}:${computer}`;
setTimeout(() => {
clickable = true;
intervalId = setInterval(changeComputerHand, 50);
}, 1000);
}
}
};
$rock.addEventListener('click', clickButton);
$scissors.addEventListener('click', clickButton);
$paper.addEventListener('click', clickButton);
</script>
</body>
</html>
반응속도 테스트
- 태그.classList.contains('클래스)를 통해서 해당 클래스가 들어있는지 true,false로 알 수 있다
- 태그.classList.add('클래스') // 추가
- 태그.classList.replace('기존클래스','수정클래스') //변경
- 태그.classList.remove('클래스') //제거
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>반응속도</title>
<style>
#screen {
width: 300px;
height: 200px;
text-align: center;
user-select: none;
}
#screen.waiting {
background-color: aqua;
}
#screen.ready {
background-color: red;
color: white;
}
#screen.now {
background-color: greenyellow;
}
</style>
</head>
<body>
<div id="screen" class="waiting">클릭해서 시작하세요</div>
<div id="result"></div>
<script>
const $screen = document.querySelector('#screen');
const $result = document.querySelector('#result');
let start = 0;
$screen.addEventListener('click', (event) => {
if (event.target.classList.contains('waiting')) {
$screen.classList.remove('waiting');
$screen.classList.add('ready');
$screen.textContent = '초록색이 되면 클릭하세요';
setTimeout(function () {
$screen.classList.remove('ready');
$screen.classList.add('now');
$screen.textContent = '클릭하세요!';
}, Math.floor(Math.random() * 1000) + 2000);
} else if (event.target.classList.contains('ready')) {
} else if (event.target.classList.contains('now')) {
}
});
</script>
</body>
</html>
OSI 7계층
TCP 헤더
서킷 스위칭
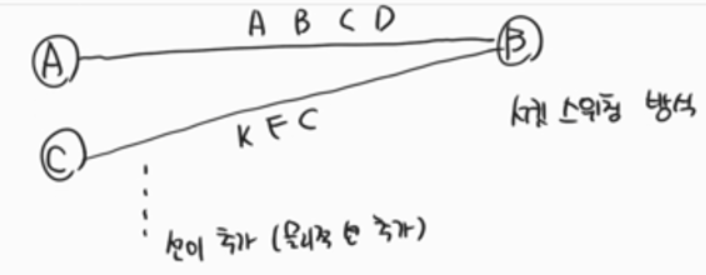
패킷 스위칭
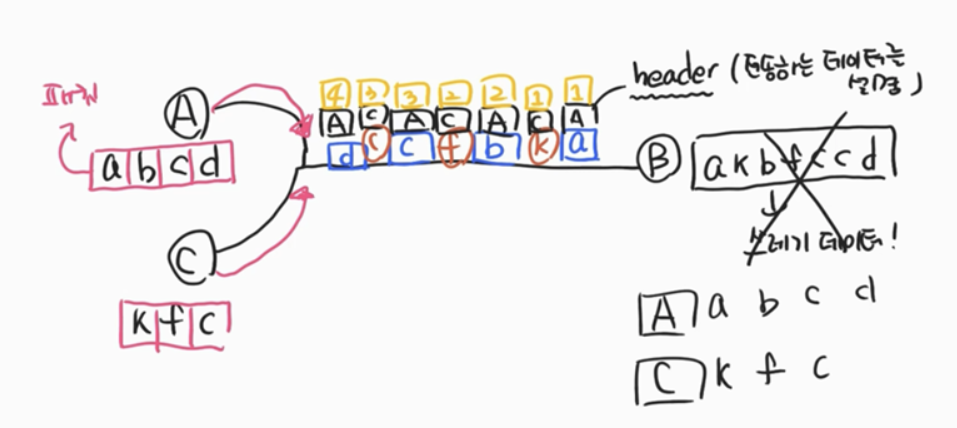
라우터
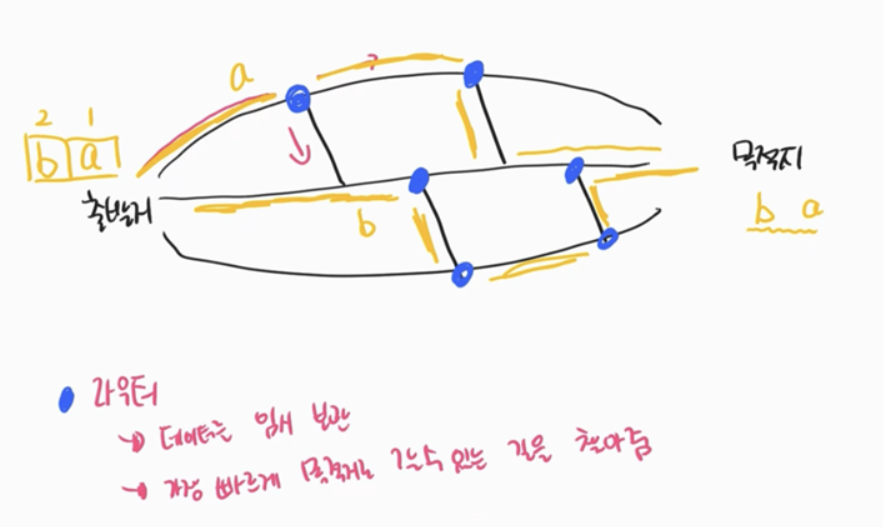
HTTP 헤더
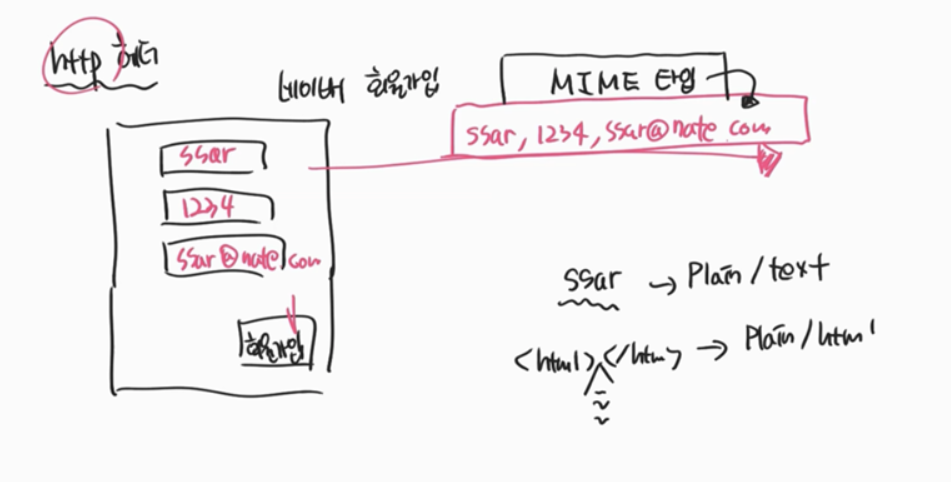