2022.03.21
스프링입문
instanceof 연산자
- 만들어진 객체가 특정 클래스의 인스턴스인지 물어보는 연산자
package ex04.instanceof01;
class 동물{
}
class 조류 extends 동물{
}
class 펭귄 extends 조류 {
}
public class Driver {
public static void main(String[] args) {
동물 동물객체 = new 동물();
조류 조류객체 = new 조류();
펭귄 펭귄객체 = new 펭귄();
System.out.println(동물객체 instanceof 동물);
System.out.println(조류객체 instanceof 동물);
System.out.println(조류객체 instanceof 조류);
System.out.println(펭귄객체 instanceof 동물);
System.out.println(펭귄객체 instanceof 조류);
System.out.println(펭귄객체 instanceof 펭귄);
System.out.println(펭귄객체 instanceof Object);
}
}
- 객체 참조 변수의 타입이 아닌 실제 객체의 타입에 의해 처리
package ex04.instanceof02;
class 동물{
}
class 조류 extends 동물{
}
class 펭귄 extends 조류 {
}
public class Driver {
public static void main(String[] args) {
동물 동물객체 = new 동물();
동물 조류객체 = new 조류();
동물 펭귄객체 = new 펭귄();
System.out.println(동물객체 instanceof 동물);
System.out.println(조류객체 instanceof 동물);
System.out.println(조류객체 instanceof 조류);
System.out.println(펭귄객체 instanceof 동물);
System.out.println(펭귄객체 instanceof 조류);
System.out.println(펭귄객체 instanceof 펭귄);
System.out.println(펭귄객체 instanceof Object);
}
}
- instanceof연산자는 클래스들의 상속 관계뿐만 아니라 인터페이스 구현 관계에서도 동일하게 적용
package ex04.instanceof03;
interface 날수있는{
}
class 박쥐 implements 날수있는{
}
class 참새 implements 날수있는{
}
public class Driver {
public static void main(String[] args) {
날수있는 박쥐객체 = new 박쥐();
날수있는 참새객체 = new 참새();
System.out.println(박쥐객체 instanceof 날수있는);
System.out.println(박쥐객체 instanceof 박쥐);
System.out.println(참새객체 instanceof 날수있는);
System.out.println(참새객체 instanceof 참새);
}
}
interface 키워드와 implements 키워드
package ex04.interface01;
public interface Speakable {
double PI = 3.14159;
final double absoluteZeroPoint = -275.15;
void sayYes();
}
this 키워드
- this는 객체가 자기 자신을 지칭할 때 쓰는 키워드
- 지역 변수와 속성(객체 변수,정적 변수)의 이름이 같은 경우 지역 변수가 우선
- 객체 변수와 이름이 같은 지역 변수가 있는 경우 객체 변수를 사용하려면 this를 접두사로 사용
- 정적 변수와 이름이 같은 지역 변수가 있는 경우 정적 변수를 사용하려면 클래스명을 접두사로 사용
package ex04.This;
class 펭귄{
int var = 10;
void test() {
int var = 20;
System.out.println(var);
System.out.println(this.var);
}
}
public class Driver {
public static void main(String[] args) {
펭귄 뽀로로 = new 펭귄();
뽀로로.test();
}
}
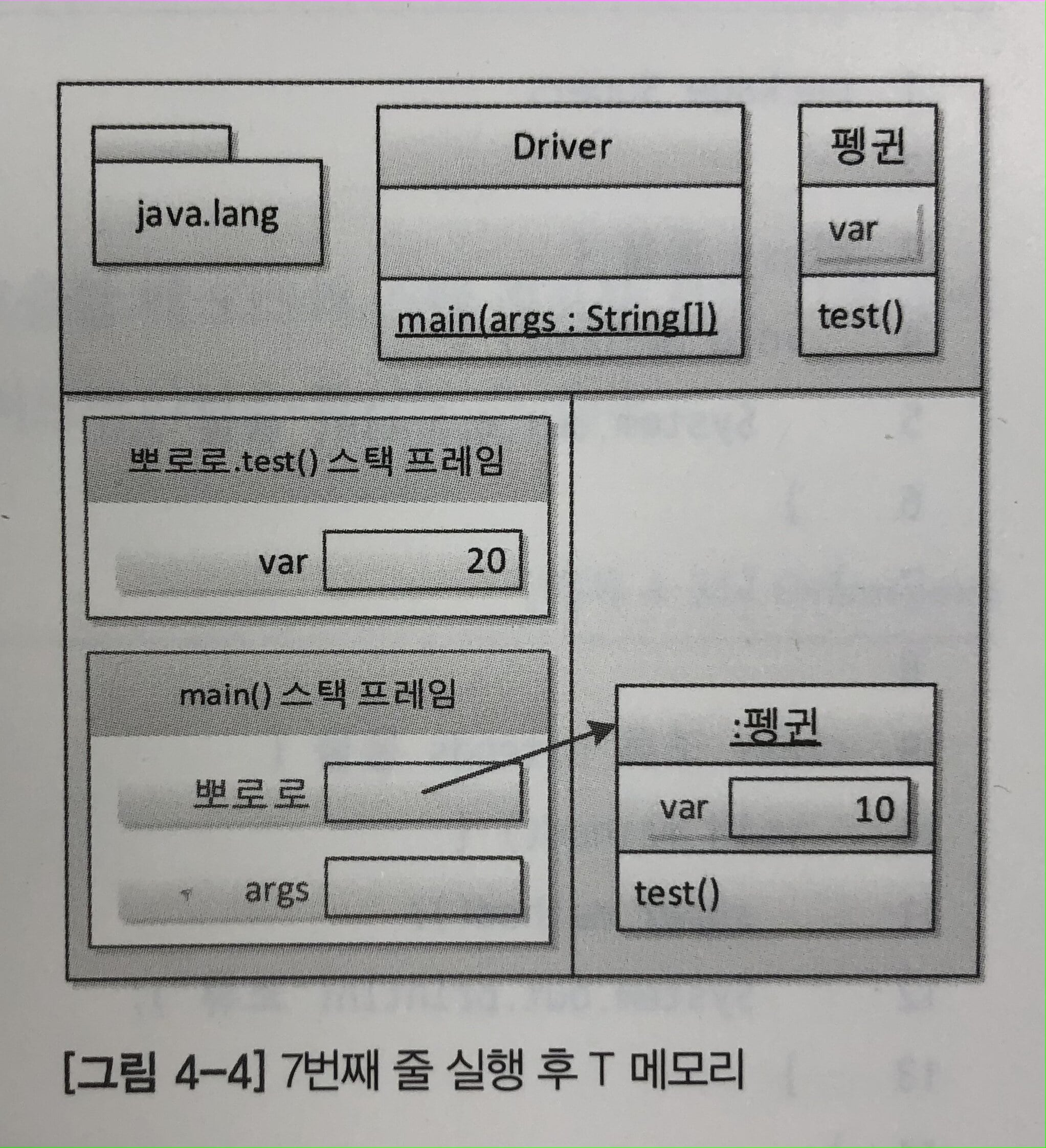
super 키워드
package ex04.Super;
class 동물{
void method() {
System.out.println("동물");
}
}
class 조류 extends 동물 {
void method() {
super.method();
System.out.println("조류");
}
}
class 펭귄 extends 조류{
void method() {
super.method();
System.out.println("펭귄");
}
}
public class Driver {
public static void main(String[] args) {
펭귄 뽀로로 = new 펭귄();
뽀로로.method();
}
}
객체 지향 설계 5원칙-SOLID
SRP - 단일 책임 원칙
- 어떤 클래스를 변경해야 하는 이유는 오직 하나뿐이어야 한다.
- 역할(책임)을 분리하라
- 속성이 단일 책임 원칙을 지키지 못한경우
class 사람{
String 군번;
}
package ex05.srp;
public class 강아지 {
final static Boolean 수컷 = true;
final static Boolean 암컷 = false;
Boolean 성별;
void 소변보다() {
if(this.성별 == 수컷) {
}else {
}
}
}
package ex05.srp;
public abstract class 강아지2 {
final static Boolean 수컷 = true;
final static Boolean 암컷 = false;
Boolean 성별;
abstract void 소변보다();
}
class 수컷강아지 extends 강아지2{
@Override
void 소변보다() {
}
}
class 암컷강아지 extends 강아지2{
@Override
void 소변보다() {
}
}