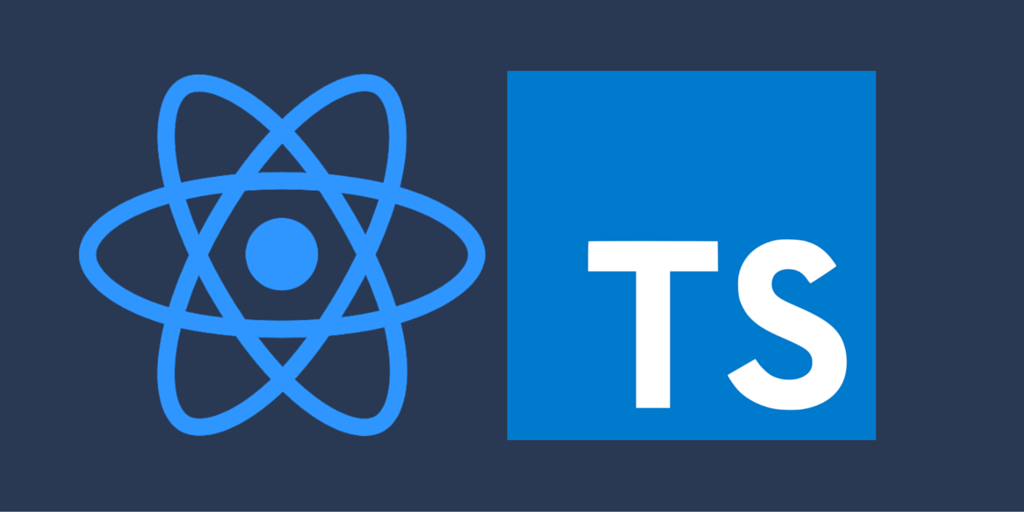
Wrap HTML Elements
- Create custom components by adding types
- Use omit keyword to take type and remove the specified properties.
import { CustomButton } from './components/html/Button'
function App() {
return (
<div className='App'>
<CustomButton variant='primary' onClick={() =>
console.log('Clicked')}>
Button Label
</CustomButton>
</div>
)
}
import React from 'react';
type ButtonProps = {
variant: 'primary' | 'secondary'
} React.ComponentProps<'button'>
export const CustomButton = ({ variant, children, ...rest }: ButtonProps) => {
return (
<button className={`class-with-${variant}`} {...rest}>
{children}
</button>
)
}
import React from 'react';
type ButtonProps = {
variant: 'primary' | 'secondary'
children: string
} & Omit<React.ComponentProps<'button'>, 'children'>
export const CustomButton = ({ variant, children, ...rest }: ButtonProps) => {
return (
<button className={`class-with-${variant}`} {...rest}>
{children}
</button>
)
}