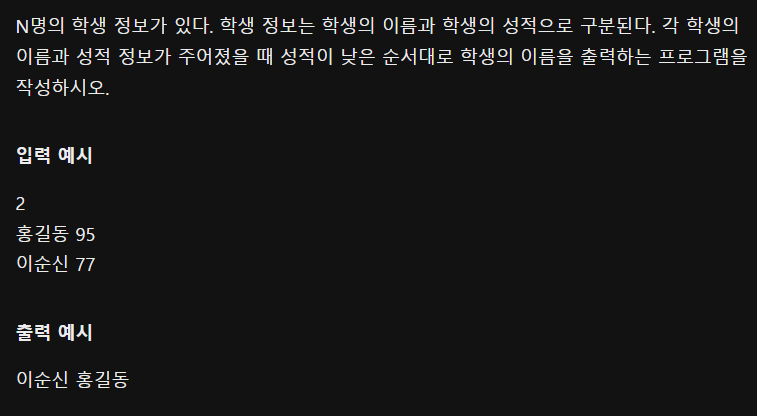
import java.util.*;
class Student implements Comparable<Student> {
private String name;
private int score;
public Student(String name, int score) {
this.name = name;
this.score = score;
}
public String getName() {
return this.name;
}
public int getScore() {
return this.score;
}
@Override
public int compareTo(Student other) {
if (this.score < other.score) {
return -1;
}
return 1;
}
}
public class Main {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
List<Student> students = new ArrayList<>();
for (int i = 0; i < n; i++) {
String name = sc.next();
int score = sc.nextInt();
students.add(new Student(name, score));
}
Collections.sort(students);
for (int i = 0; i < students.size(); i++) {
System.out.print(students.get(i).getName() + " ");
}
}
}
import java.util.*;
import java.io.*;
public class Main {
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
StringTokenizer st = new StringTokenizer(br.readLine());
int N = Integer.parseInt(st.nextToken());
List<Student> list = new ArrayList<>();
for (int i = 0; i < N; i++) {
st = new StringTokenizer(br.readLine());
String name = st.nextToken();
int score = Integer.parse(st.nextToken());
list.add(new Student(name, score));
}
Collections.sort(list);
for (int i = 0; i < list.size(); i++) {
System.out.print(list.get(i).getName() +" ");
}
}
}
class Student implements Comparable<Student> {
private String name;
private int score;
public Student(String name, int score) {
this.name = name;
this.score = score;
}
public String getName() {
return this.name;
}
public int getScore() {
return this.score;
}
@Override
public int compareTo(Student other) {
if (this.score < other.score) return -1;
return 1;
}
}