3.1 Process Concept
프로세스란 무엇인가?
- 프로세스는 실행중인 프로그램을 말한다.
-> a.out이 메모리에 올라가서 실행될 때 이것이 프로세스
- 프로세스는 운영체제의 작업 단위이다.
프로세스 메모리 구조 - The memory layout of a process
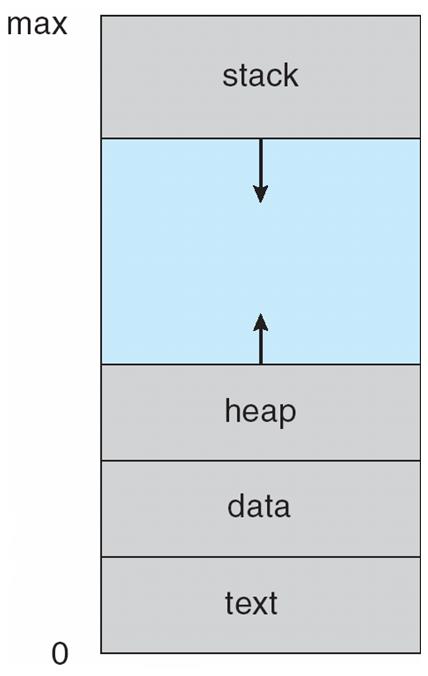
- Text section: 실행 코드
- Data section: 전역 변수
- Heap section: 프로그램 실행 중 동적으로 할당되는 메모리
- Stack section: 함수와 관련된 정보들
#include <stdio.h>
#include <stdlib.h>
int x;
int y = 15;
int main(int argc, char *argv[])
{
int *values;
int i;
values = (int *)malloc(sizeof(int)*5);
for (i = 0; i < 5; i++)
values[i] = i;
return 0;
}
프로세스의 생명주기
- New: 프로세스가 막 만들어진 상태
- Running: CPU를 점유해서 명령어를 수행하는 상태
- Waiting: I/O 또는 이벤트를 기다리느라 CPU를 점유하지 않은 상태
- Ready: CPU를 점유하기 위해 대기하는 상태
- Terminated: exit() 또는 return으로 종료된 상태
-> Running에서 Ready로 가는 것과 Running에서 Waiting으로 가는 것이 헷갈림
- 스케줄러가 Ready에서 Running으로 보내는 것을 dispatch라 한다.
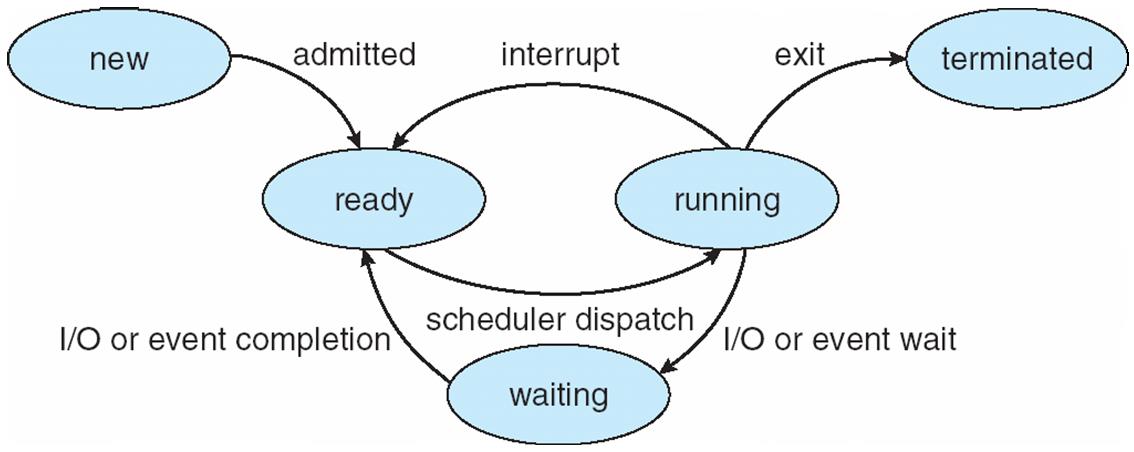
PCB(Process Controls Block)
운영체제가 관리해야 하는 프로세스 컨트롤 블록
- Process state: 프로세스 상태(new/running/waiting/ready/terminated)
- Program counter: 명령어의 메모리 주소를 보관하는 레지스터
- CPU registers: IR, DR…
* Program counter, CPU registers 포함해서 context(문맥)라고 한다.
- CPU-scheduling information: CPU를 사용하거나 릴리즈하기 위해 사용하는 스케줄링 정보
- Memory-management information: malloc 정보 들
- Accounting information: 유저 정보
- I/O status information: 파일 등 자원 락 등
3.2 Process Scheduling
Scheduling Queues
- ready queue: 프로세스가 running 상태로 가기 위한 대기열
- wait queue: ready 상태로 가기 위한 대기열
Context Switch - 문맥 교환
어떤 태스크가 다른 프로세스에게 CPU를 넘겨주는 것
- 현재 프로세스의 문맥을 저장하고,
- 다른(새로 진행할) 프로세스의 문맥을 보관한다.
-> CPU의 레지스터에 PCB 정보를 로드하고, 저장한다.
- 시분할과 문맥교환을 통해 프로그램을 동시에(concurrently) 실행할 수 있다.
3.3 Operations on Processes
fork()
OS에게 프로세스를 복제해달라고 요청하는 system call
- system call
- execute concurrently
- wait
#include <stdio.h>
#include <unistd.h>
#include <wait.h>
int main() {
pid_t pid;
pid = fork();
if (pid < 0) {
fprintf(stderr, "Fork Failed");
return 1;
}
else if (pid == 0) {
execlp("/bin/ls", "ls", NULL);
}
else {
wait(NULL);
printf("Child Complete");
}
return 0;
}
Zombie and Orphan
- zombie process: 부모 클래스가 wait()를 안하고, 자식 프로세스를 신경쓰지 않는 경우(자식 프로세스는 backround에 계속 남게 됨)
- orphan process: 부모 클래스가 wait()를 하지 않고 종료한 경우, 고아 프로세스