브릿지패턴
- 구조패턴
- 추상적 개념과 구현을 분리하여 이들이 독립적으로 다양성을 가질 수 있도록 하는 패턴
- 추상적 개념이 사용자의 요청을 구현 객체에 전달하는 방식
활용성
- 런타임에 구현 방법을 선택하거나 구현 내용을 변경하고 싶을때
- 추상적 개념과 구현 모두 독립적으로 확장되어야 할 때
- 추상적 개념에 대한 구현 내용을 변경하는 것이 다른 코드에 영향을 주지 않아야 할 때
구조
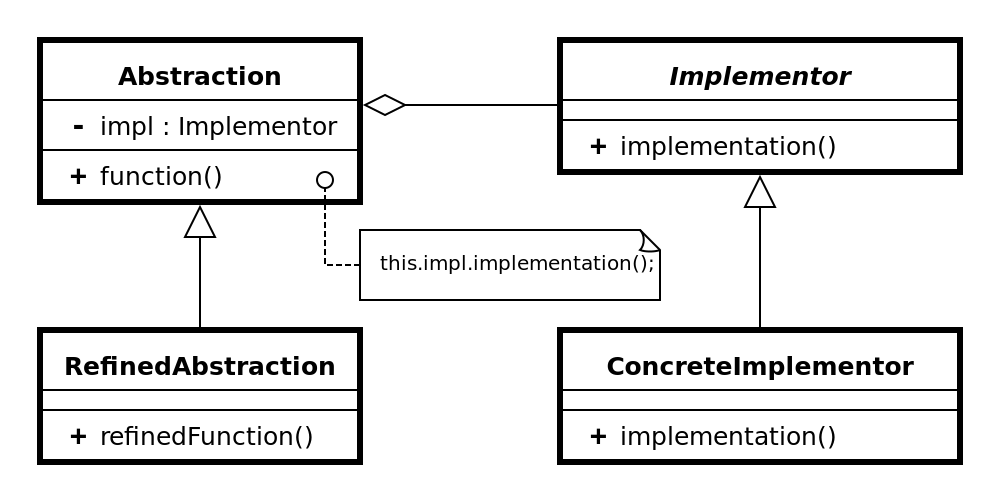
요소
- 추상적 개념(Abstraction)
- 추상적 개념에 대한 인터페이스를 제공하고 구현 객체에 의존해 실제 하위 수준 작업들을 수행
- 정제된 추상화(RefinedAbstraction), 선택사항
- 구현자 (Implementor)
- 구상 구현에 대한 인터페이스 정의
- 추상화와 다른 형태일 수 있다.
- 일반적으로 구현은 기본적인 구현 연산을 수행하고 추상화는 더 추상화된 서비스 관점의 인터페이스를 제공한다.
- 구상 구현(Concretelmplementor)
장점
- 인터페이스 구현 분리
- 런타임에 어떤 객체가 자신의 구현을 수시로 변경할 수 있다.
- 컴파일 타임 의존성 제거
- 구현 은닉화
- 단일 책임 원칙
- 추상화의 상위 수준 논리와 구현의 플랫폼 세부 정보에 집중
- 개방/폐쇄 원칙
- 새로운 추상화들과 구현들을 상호 독립적으로 도입
단점
예시 코드
protocol Abstraction {
func function(impl: Implementor)
}
protocol Implementor {
func implementation()
}
struct AbstractionA: Abstraction {
func function(impl: Implementor) {
impl.implementation()
}
}
struct ImplementorA: Implementor {
func implementation() {
print("ImplementorA")
}
}
struct ImplementorB: Implementor {
func implementation() {
print("ImplementorB")
}
}
let a = AbstractionA()
let iA = ImplementorA()
let iB = ImplementorB()
a.function(impl: iA)
a.function(impl: iB)
protocol DatabaseConnection {
func connect()
func executeQuery(query: String) -> [String]
func disconnect()
}
class SQLiteDatabaseConnection: DatabaseConnection {
func connect() {
print("SQLite: Connected to the database")
}
func executeQuery(query: String) -> [String] {
print("SQLite: Executing query - \(query)")
return ["Result 1", "Result 2"]
}
func disconnect() {
print("SQLite: Disconnected from the database")
}
}
class MySQLDatabaseConnection: DatabaseConnection {
func connect() {
print("MySQL: Connected to the database")
}
func executeQuery(query: String) -> [String] {
print("MySQL: Executing query - \(query)")
return ["Result A", "Result B"]
}
func disconnect() {
print("MySQL: Disconnected from the database")
}
}
class DatabaseClient {
let databaseConnection: DatabaseConnection
init(connection: DatabaseConnection) {
self.databaseConnection = connection
}
func performDatabaseOperation() {
databaseConnection.connect()
let results = databaseConnection.executeQuery(query: "SELECT * FROM some_table")
print("Results: \(results)")
databaseConnection.disconnect()
}
}
let sqliteConnection = SQLiteDatabaseConnection()
let mysqlConnection = MySQLDatabaseConnection()
let sqliteClient = DatabaseClient(connection: sqliteConnection)
let mysqlClient = DatabaseClient(connection: mysqlConnection)
sqliteClient.performDatabaseOperation()
mysqlClient.performDatabaseOperation()
참고