1. 현재 경로 위치 확인
$currentPath = getcwd();
echo "현재 위치: " . $currentPath;
2. k번째 약수
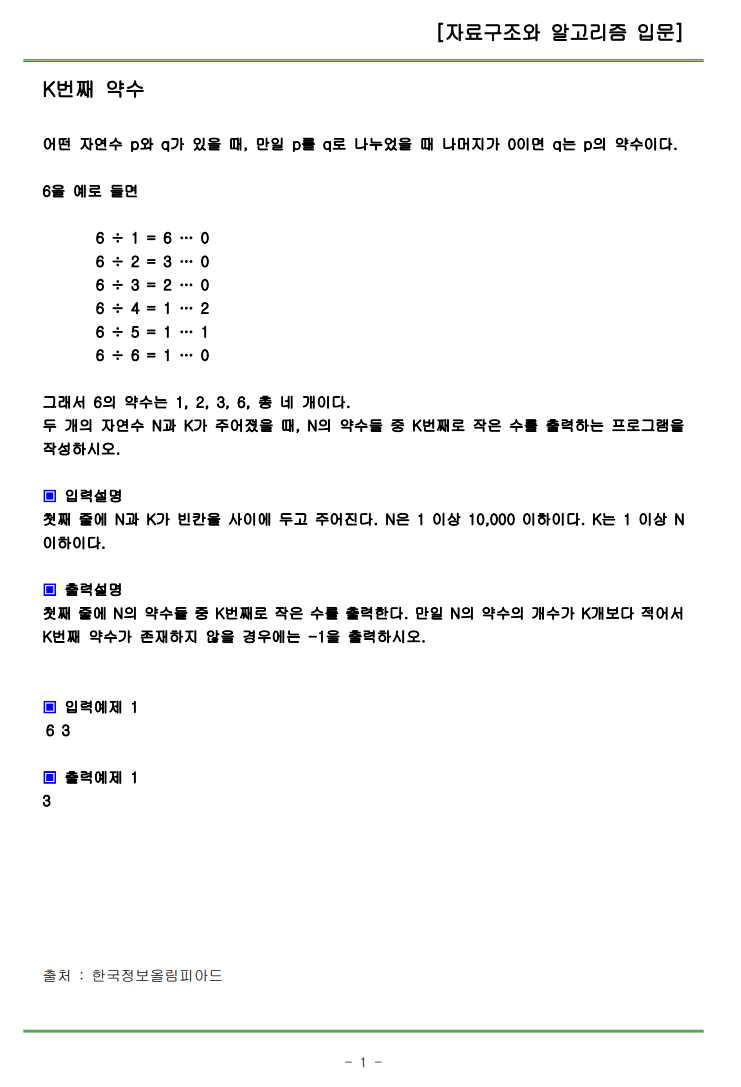
Code-1
<?php
$input_result = function ($path) {
$file = fopen($path, "r");
$line = fgets($file);
fclose($file);
return array_map('intval', explode(' ', $line));
};
$output_result = function ($path) {
$file = fopen($path, "r");
$line = fgets($file);
fclose($file);
return intval(trim($line));
};
$kth_divisor = function ($n, $k) {
$cnt = 0;
for ($i = 1; $i <= $n; $i++) {
if ($n % $i == 0) {
$cnt++;
}
if ($cnt == $k) {
return $i;
}
}
echo "return false" . "\n";
return false;
};
$test_kth_divisor = function () use ($input_result, $output_result, $kth_divisor) {
$COMMON_PATH = "inflearn/python/섹션 2/1. k번째 약수/";
$INPUT_PATH = "./" . $COMMON_PATH . "in1.txt";
$OUTPUT_PATH = "./" . $COMMON_PATH . "out1.txt";
list($n, $k) = $input_result($INPUT_PATH);
$expected = $output_result($OUTPUT_PATH);
assert($kth_divisor($n, $k) === $expected);
};
$test_kth_divisor();
echo "Test Pass";
?>
Code-2
<?php
class KthDivisorTest
{
private static $COMMON_PATH;
private static $numTestCases;
public function __construct()
{
self::$COMMON_PATH = "inflearn/python/섹션 2/1. k번째 약수/";
self::$numTestCases = 5;
}
private function readInput($path)
{
$file = fopen($path, "r");
$line = fgets($file);
fclose($file);
return array_map('intval', explode(' ', $line));
}
private function readOutput($path)
{
$file = fopen($path, "r");
$line = fgets($file);
fclose($file);
return intval(trim($line));
}
private function kthDivisor($n, $k)
{
$cnt = 0;
for ($i = 1; $i <= $n; $i++) {
if ($n % $i == 0) {
$cnt++;
}
if ($cnt == $k) {
return $i;
}
}
return -1;
}
private function runTestCases()
{
for ($i = 1; $i <= self::$numTestCases; $i++) {
$inputPath = "./" . self::$COMMON_PATH . "in$i.txt";
$outputPath = "./" . self::$COMMON_PATH . "out$i.txt";
$input = $this->readInput($inputPath);
$expected = $this->readOutput($outputPath);
$n = $input[0];
$k = $input[1];
$result = $this->kthDivisor($n, $k);
assert($result === $expected, "Test case $i failed. n=$n, k=$k, expected=$expected");
}
}
public function testKthDivisor()
{
$this->runTestCases();
}
}
$test = new KthDivisorTest();
$test->testKthDivisor();
?>
isset함수
define('ENVIRONMENT', isset($_SERVER['CI_ENV']) ? $_SERVER['CI_ENV'] : 'production');