Store
개요
- 상태(데이터)를 저장하는 곳
{ createStore } from "redux";
사용하여 생성합니다.
const countStore = createStore(reducer);
- Store 는 5 개의 함수를 가지고 있습니다.
console.log(countStore);
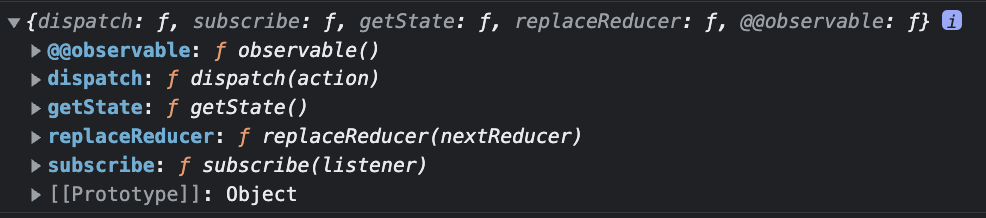
메서드
getState()
: 해당 Store 의 값을 반환하는 메서드입니다.
Ruducer
개요
- 순수함수, 상태(데이터)를 변경하는 == Setter || Set함수
- 유일하게 데이터를 변경할 수 있는 함수입니다.
인자
- 첫번째 인자로
state
, 두번째 인자로 action
을 받습니다.
action
의 type
에 따라 로직(연산)을 분기합니다.
반환값
Reducer
가 반환하는 값은 State
의 값이 됩니다.
Action
개요
- Using when
Reducer
modifies State
- 객체입니다.
type
이라는 key 가 기본으로 있습니다.
메서드
dispatch()
메서드가 있습니다.
- 해당 메서드를 사용해
Action
의 type
키값을 변경합니다.
Reducer
는 변경된 Action
의 type
을 토대로 State
값을 변경합니다.
- key 를 추가하여 데이터를
Reducer
에게 넘겨 줄 수 있습니다.
Subscribe
개요
State
의 변화를 감지하여 콜백함수를 실행합니다.
- VanillaJS 에서
State
변화에 따라 리페인팅(리렌더링) 해주는 역할을 합니다.
Vanilla Counter with Redux
리팩토링 전
import { createState } from "redux";
const add = document.getElementById("add");
const minus = document.getElementById("minus");
const number = document.querySelector("span");
number.innerTexxt = 0;
const countModifier = (count = 0, action) => {
if (action.type === "ADD") {
return count + 1;
} else if (action.type === "MINUS") {
return count - 1;
}
return count;
}
const countStore = createStore(countModifier);
countStore.subscribe(() => (number.innerText = countStore.getState());
add.addEventListener("click", () => countStore.dispatch({ type: "ADD" }));
minus.addEventListener("click", () => countStore.dispatch({ type: "MINUS" }));
리팩토링 후
import { createStore } from "redux";
const add = document.getElementById("add");
const minus = document.getElementById("minus");
const number = document.querySelector("span");
number.innerText = 0;
const ADD = "ADD";
const MINUS = "MINUS";
const countModifier = (count = 0, action) => {
switch (action.type) {
case ADD:
return count + 1;
case MINUS:
return count - 1;
default:
return count;
}
};
const countStore = createStore(countModifier);
countStore.subscribe(() => (number.innerText = countStore.getState()));
add.addEventListener("click", () => countStore.dispatch({ type: ADD }));
minus.addEventListener("click", () => countStore.dispatch({ type: MINUS }));