mariadb연동으로 db데이터 페이지에서 출력하기
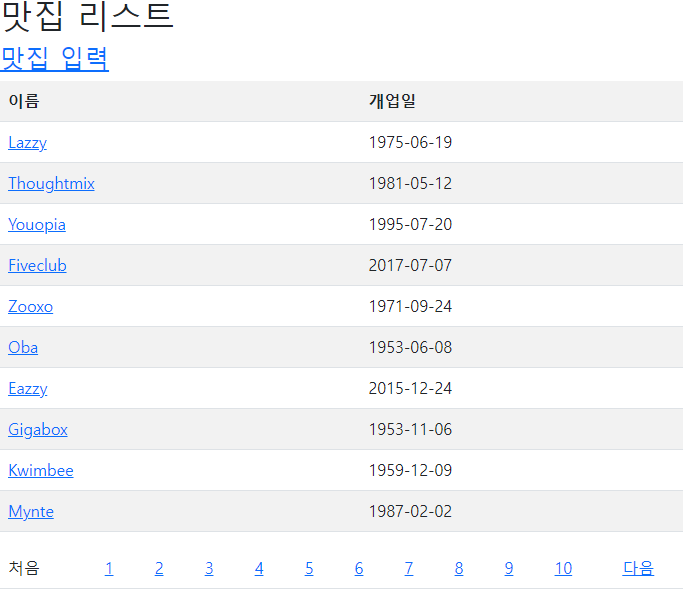
<%@page import="java.sql.PreparedStatement"%>
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.ResultSet" %>
<%@ page import="java.sql.DriverManager"%>
<%
// db연결
Class.forName("org.mariadb.jdbc.Driver");
// 디버깅
System.out.println("연결됨");
java.sql.Connection conn = DriverManager.getConnection(
"jdbc:mariadb://127.0.0.1:3306/homework0419", "root", "java1234");
// 디버깅
System.out.println("로그인됨");
// currentPage 설정
int currentPage = 1;
if (request.getParameter("currentPage") != null) {
currentPage = Integer.parseInt(request.getParameter("currentPage"));
}
// limit 설정 및 페이지 당 노출 row 수 설정
int rowPerPage = 10;
int startRow = (currentPage - 1) * rowPerPage;
String sql = "select store_no, store_name, store_begine from store order by store_no desc limit ?, ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, startRow);
stmt.setInt(2, rowPerPage);
ResultSet rs = stmt.executeQuery();
// lastPage 설정
// db의 총 갯수 파악
String sql2 = "select count(*) from store";
PreparedStatement stmt2 = conn.prepareStatement(sql2);
ResultSet rs2 = stmt2.executeQuery();
int totalRow = 0;
if (rs2.next()){
totalRow = rs2.getInt("count(*)");
}
// 디버깅
System.out.println(totalRow);
// lastPage 계산, 올림을 이용해서 계산 하였음.
// totalRow나 rowPerPage 둘다 int 형이면 int형으로 나눠져서 9가 되기 때문에 double로 형변환 시킴
int lastPage = (int)Math.ceil((double)totalRow / rowPerPage);
// 디버깅
System.out.println(lastPage);
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>storeList.jsp</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<h1>맛집 리스트</h1>
<h3>
<a href="./insertStoreForm.jsp">
맛집 입력
</a>
</h3>
<table class="table table-striped">
<tr>
<th>이름</th>
<th>개업일</th>
</tr>
<%
// rs.nest()를 활용해서 rs의 다음요소가 존재 하지 않을때까지 반복해서 출력
// 지금은 limit를 걸어서 10개까지 나온다
while (rs.next()){
%>
<tr>
<td>
<a href="./storeOne.jsp?storeNo=<%=rs.getInt("store_no")%>¤tPage=<%=currentPage%>">
<%=rs.getString("store_name")%>
</a>
</td>
<td><%=rs.getString("store_begine")%></td>
</tr>
<%
}
%>
</table>
<table class="table">
<tr>
<%
// 첫페이지
if (currentPage > 1) {
%>
<td>
<a href="./storeList.jsp?currentPage=<%=currentPage - 1%>">이전</a>
</td>
<%
} else {
%>
<td>
처음
</td>
<%
}
// 혼자서 해본 페이지 수 나누기
// 페이지 수 나열
int startPageNum = ((currentPage - 1) / 10) * 10 + 1;
// 마지막 페이지 수 나열
int maxPageNum = startPageNum + 10;
// 마지막 페이지 예외 처리
if (maxPageNum > lastPage) {
maxPageNum = lastPage + 1;
}
for (int i = startPageNum; i < maxPageNum; i++) {
%>
<td>
<a href="./storeList.jsp?currentPage=<%=i%>">
<%=i%>
</a>
</td>
<%
}
// 마지막 페이지
if (currentPage < lastPage) {
%>
<td>
<a href="./storeList.jsp?currentPage=<%=currentPage + 1%>">다음</a>
</td>
<%
} else {
%>
<td>
마지막
</td>
<%
}
%>
</tr>
</table>
</body>
</html>
선택한 데이터 출력
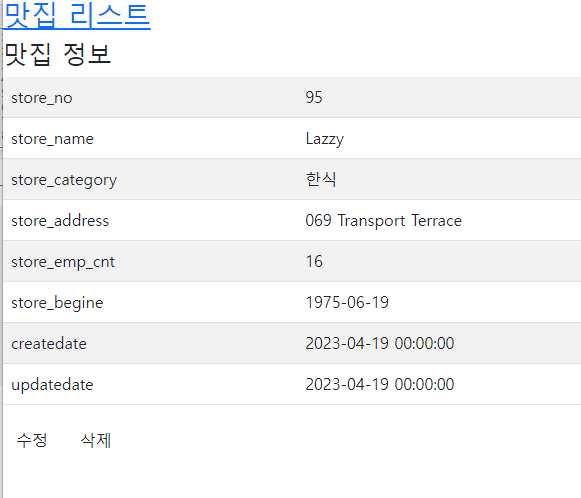
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<%@ page import="java.sql.Connection" %>
<%@ page import="java.sql.DriverManager" %>
<%@ page import="java.sql.PreparedStatement" %>
<%@page import="java.sql.ResultSet"%>
<%
if (request.getParameter("currentPage") == null){
response.sendRedirect("./storeList.jsp");
return;
}
// storeList.jsp에서 storeNo(기본키)를 가져와서 원하는 데이터를 출력할 수 있다.
int storeNo = Integer.parseInt(request.getParameter("storeNo"));
int currentPage = Integer.parseInt(request.getParameter("currentPage"));
Class.forName("org.mariadb.jdbc.Driver");
java.sql.Connection conn = DriverManager.getConnection(
"jdbc:mariadb://127.0.0.1:3306/homework0419", "root", "java1234");
String sql = "select store_no, store_name, store_category, store_address, store_emp_cnt, store_begine, createdate, updatedate from store where store_no = ?";
PreparedStatement stmt = conn.prepareStatement(sql);
stmt.setInt(1, storeNo);
System.out.println(stmt + " <-- stmt");
ResultSet rs = stmt.executeQuery();
%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.2.3/dist/js/bootstrap.bundle.min.js"></script>
</head>
<body>
<div>
<h2>
// currentPage값을 가져와서 입력해 둠으로써 storeList.jsp로 돌아갈때 이전 페이지로 넘어 갈 수 있다.
<a href="./storeList.jsp?currentPage=<%=currentPage%>">맛집 리스트</a>
</h2>
</div>
<h3>맛집 정보</h3>
<%
rs.next();
%>
<table class="table table-striped">
<tr>
<td>store_no</td>
<td><%=rs.getInt("store_no")%></td>
</tr>
<tr>
<td>store_name</td>
<td><%=rs.getString("store_name")%></td>
</tr>
<tr>
<td>store_category</td>
<td><%=rs.getString("store_category")%></td>
</tr>
<tr>
<td>store_address</td>
<td><%=rs.getString("store_address")%></td>
</tr>
<tr>
<td>store_emp_cnt</td>
<td><%=rs.getInt("store_emp_cnt")%></td>
</tr>
<tr>
<td>store_begine</td>
<td><%=rs.getString("store_begine")%></td>
</tr>
<tr>
<td>createdate</td>
<td><%=rs.getString("createdate")%></td>
</tr>
<tr>
<td>updatedate</td>
<td><%=rs.getString("updatedate")%></td>
</tr>
</table>
<div>
<a class="btn" href="./updateStoreForm.jsp?storeNo=<%=storeNo%>¤tPage=<%=currentPage%>">수정</a>
<a class="btn" href="./deleteStoreForm.jsp?storeNo=<%=storeNo%>¤tPage=<%=currentPage%>">삭제</a>
</div>
</body>
</html>