폼
- 서버로 데이터를 전송하기 위한 수단
- jQuery는 폼을 제어하는데 필요한 이벤트와 메소드를 제공
- jQuery 폼 API 문서 참고
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<body>
<p>
<input type="text"/>
<span></span>
</p>
<script>
$('input').focus(function(){
$(this).next('span').html('focus');
}).blur(function(){
$(this).next('span').html('blur');
}).change(function(e){
$(this).next('span').html($(e.target).val());
}).select(function(){
$(this).next('span').html('select');
})
</script>
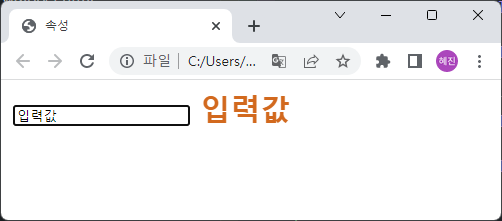
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<style>
p {
margin: 0;
color: blue;
}
span {
color: chocolate;
}
div {
margin-left: 10px;
}
</style>
<body>
<p>
Type 'correct' to validate.
</p>
<!-- focus, blur, change, select -->
<form action="">
<div>
<input type="text"/>
<input type="submit" value="질의보내기"/>
</div>
</form>
<span></span>
<script>
$('form').submit(function() {
if($('input:first').val() == 'correct')
{
$('span').text('validated...').show().fadeIn(3000);
return true;
}
$('span').text('not valid...').show().fadeOut(2000);
return false;
})
</script>
</body>
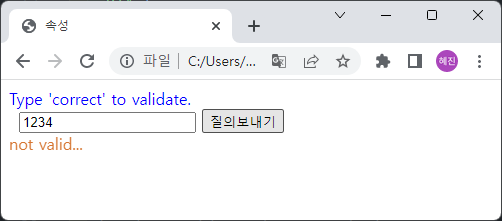
애니메이션
효과란
- 자바스크립트와 CSS를 이용해서 HTML 엘리먼트에 동적인 효과를 줄 수 있다.
- jQuery의 효과 메소드를 호출하는 것만으로도 간단한 효과를 줄 수 있다.
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<style>
div {
margin: 3px;
width: 80px;
height: 80px;
background-color: cornflowerblue;
}
</style>
<body>
<input type="button" id="fadeout" value="fadeout"/>
<input type="button" id="fadein" value="fadein"/>
<input type="button" id="hide" value="hide"/>
<input type="button" id="show" value="show"/>
<input type="button" id="slidedown" value="slidedown"/>
<input type="button" id="slideup" value="slideup"/>
<input type="button" id="mix" value="mix"/>
<div id="target">target</div>
<script>
$('input[type="button"]').click(function(e){
switch($(e.target).attr('id'))
{
case 'fadeout':
$('#target').fadeOut(5000);
break;
case 'fadein':
$('#target').fadeIn(2000);
break;
case 'hide':
$('#target').hide();
break;
case 'show':
$('#target').show();
break;
case 'slidedown':
$('#target').hide().slideDown(5000);
break;
case 'slideup':
$('#target').slideUp('slow');
break;
case 'mix':
$('#target').fadeOut(5000).fadeIn(3000).delay(1000).
slideUp().slideDown('slow', function(){alert('end')});
break;
}
})
</script>
</body>
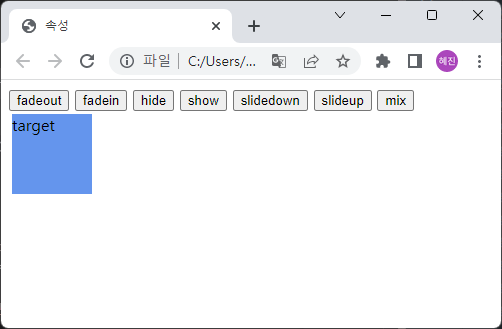
<script src="http://code.jquery.com/jquery-latest.min.js"></script>
<style>
div {
width: 300px;
background-color: skyblue;
border: 1px solid green;
}
</style>
<body>
<button id="go">
전방에 5초간 함성발사
</button>
<div id="block">
이건 사야해~
</div>
<script>
$('#go').click(function(){
$('#block').animate({
width: "800px",
opacity : 0.4,
marginLeft : "50px",
fontSize : "70px",
borderWidth : "10px"
}, 5000);
})
</script>
</body>
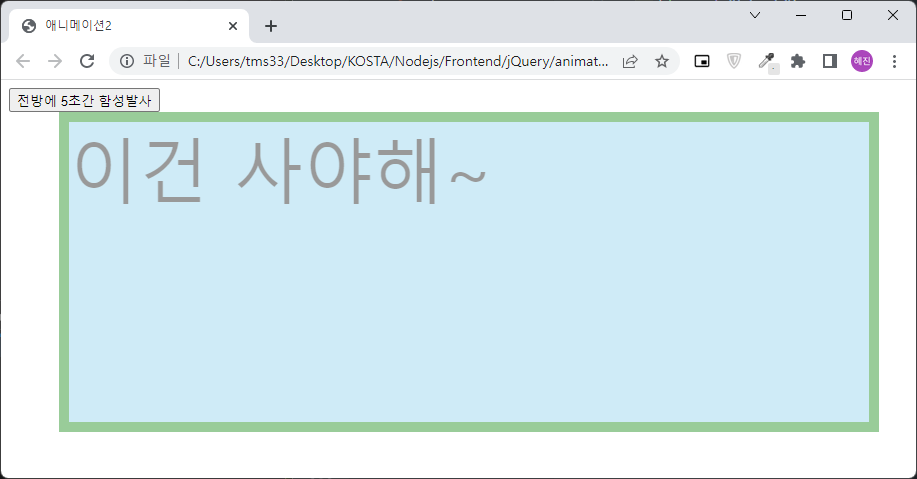