WHERE 절
WHERE 절
: DML 문을 수행할 때 원하는 데이터를 조건으로 골라 수행할 수 있도록 해주는 구문
INSERT를 제외한 SELECT, UPDATE, DELETE에서 WHERE 사용 가능
1. 비교 연산자
연산자 | 의미 | 예시 |
---|
== | 같음 | where col = 10 |
< | 작음 | where col < 10 |
<= | 작거나 같음 | where col <= 10 |
> | 큼 | where col > 10 |
>= | 크거나 같음 | where col >= 10 |
2. 부정 비교 연산자
연산자 | 의미 | 예시 |
---|
!= | 같지 않음 | where col != 10 |
^= | 같지 않음 | where col ^= 10 |
<> | 같지 않음 | where col <> 10 |
not 컬럼명 = | 같지 않음 | where not col = 10 |
not 컬럼명 > | 크지 않음 | where not col > 10 |
코드 | 테이블 |
---|
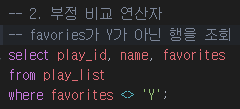 | 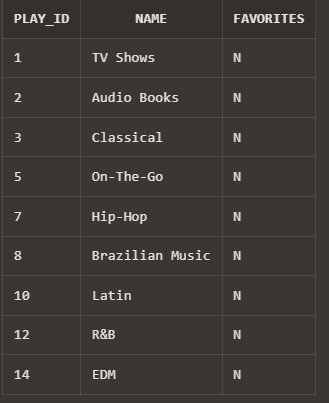 |
3. SQL 연산자
연산자 | 의미 | 예시 |
---|
BETWEEN A AND B | A와 B사이 (A, B 포함) | where col between 1 and 10 |
LIKE '비교 문자열' | 비교 문자열을 포함 | where col like '방탄%' where col like '%소년단' where col like '%탄소년%' where col like '방_소%' |
IN (LIST) | LIST 중 하나와 일치 | where col in(1, 3, 5) |
IS NULL | NULL 값 | where col is null |
4. 부정 SQL 연산자
연산자 | 의미 | 예시 |
---|
NOT BETWEEN A AND B | A와 B사이가 아님 (A, B 미포함) | where col not between 1 and 10 |
NOT IN (LIST) | LIST 중 일치하는 것이 없음 | where col not in(1, 3, 5) |
IS NOT NULL | NULL 값이 아님 | where col is not null |
5. 논리 연산자
연산자 | 의미 | 예시 |
---|
AND | 모든 조건이 TRUE여야 함 | where col>1 and col<10 |
OR | 하나 이상의 조건이 TRUE여야 함 | where col =1 or col = 10 |
NOT | TRUE면 FALSE고 FALSE면 TRUE | where not col >10 |
전체 코드
create table member(
member_no number(10),
first_name varchar2(20),
last_name varchar2(20),
city varchar2(15),
title varchar2(30),
company varchar2(30)
);
create table play_list(
play_id number (5),
name varchar2 (30),
favorites varchar (5)
);
insert into member values(1, 'Luis', 'Goncla', 'Paris', 'Sales Support Agent', 'Embraer');
insert into member values(2, 'Kim', 'Minjae', 'Seoul', 'IT staff', 'Googgle');
insert into member values(3, 'Yoshida', 'Maya', 'Tokyo', 'IT staff', 'Googgle');
insert into member values(4, 'Jimmy', 'Carter', 'New York', 'Guard', 'Gaia');
insert into member values(5, 'Eden', 'Hazard', 'Brussels', 'Driver', 'Telluscom');
insert into member values(6, 'Oh', 'Maigot', 'New York', 'Customer Service', 'Embraer');
insert into member values(7, 'Son', 'Heungmin', 'Seoul', 'HR', 'Telluscom');
insert into member values(8, 'Frank', 'Lampard', 'London', 'General Manager', 'RobotCo');
insert into member values(9, 'Leonie', 'Messia', 'Buenos Aires', 'Sales Support Agent', 'JetBrains');
insert into member values(10, 'Dominique', 'Lefebvre', 'Paris', 'Driver', 'JetBrains');
insert into member values(11, 'Mc', 'Donald', 'New York', 'Customer Service', 'RobotCo');
insert into member values(12, 'Xian', 'Juno', 'Paris', 'Sales Support Agent', 'Telus');
select * from member;
insert into play_list values(1, 'TV Shows', 'N');
insert into play_list values(2, 'Audio Books', 'N');
insert into play_list values(3, 'Classical', 'N');
insert into play_list values(4, 'Grunge', 'Y');
insert into play_list values(5, 'On-The-Go','N');
insert into play_list values(6, 'Movies', 'Y');
insert into play_list values(7, 'Hip-Hop', 'N');
insert into play_list values(8, 'Brazilian Music', 'N');
insert into play_list values(9, 'Music Videos', 'Y');
insert into play_list values(10, 'Latin', 'N');
insert into play_list values(11, 'Heavy Metal', 'Y');
insert into play_list values(12, 'R&B', 'N');
insert into play_list values(13, 'Classical 101 - Deep Cuts', 'Y');
insert into play_list values(14, 'EDM', 'N');
insert into play_list values(15, 'Skate', 'Y');
select * from play_list;
select first_name, last_name, city
from member
where city = 'Paris';
select member_no, first_name, last_name
from member
where member_no < 10;
select play_id, name, favorites
from play_list
where favorites <> 'Y';
select play_id, name, favorites
from play_list
where play_id between 1 and 5;
select play_id, name, favorites
from play_list
where play_id >=1 and play_id <=5;
select play_id, name, favorites
from play_list
where name like 'Classical%';
select play_id, name, favorites
from play_list
where name like '%Music';
select play_id, name, favorites
from play_list
where name like 'M%s';
select play_id, name, favorites
from play_list
where name like '%101%';
select play_id, name, favorites
from play_list
where name in ('Latin', 'EDM');
select play_id, name, favorites
from play_list
where (name='Latin' or name='EDM');
select play_id, name, favorites
from play_list
where play_id not between 1 and 5;
select play_id, name, favorites
from play_list
where not (play_id between 1 and 5);
select play_id, name, favorites
from play_list
where not (play_id >=1 and play_id <= 5);
select play_id, name, favorites
from play_list
where play_id < 1 or play_id > 5;
select play_id, name, favorites
from play_list
where name not in ('Latin', 'EDM');
select play_id, name, favorites
from play_list
where not (name in ('Latin', 'EDM'));
select play_id, name, favorites
from play_list
where not (name = 'Latin' or name = 'EDM');
select play_id, name, favorites
from play_list
where (name <> 'Latin' and name <> 'EDM');
select play_id, name, favorites
from play_list
where name is not null;
select last_name, first_name, city
from member
where title='Sales Support Agent' and city = 'Paris';
select last_name, first_name, city
from member
where title = 'Sales Support Agent' or city = 'Paris';
select last_name, first_name, city
from member
where not (title = 'Sales Support Agent' or city = 'Paris');
select last_name, first_name, city
from member
where title <> 'Sales Support Agent' and city <> 'Paris';