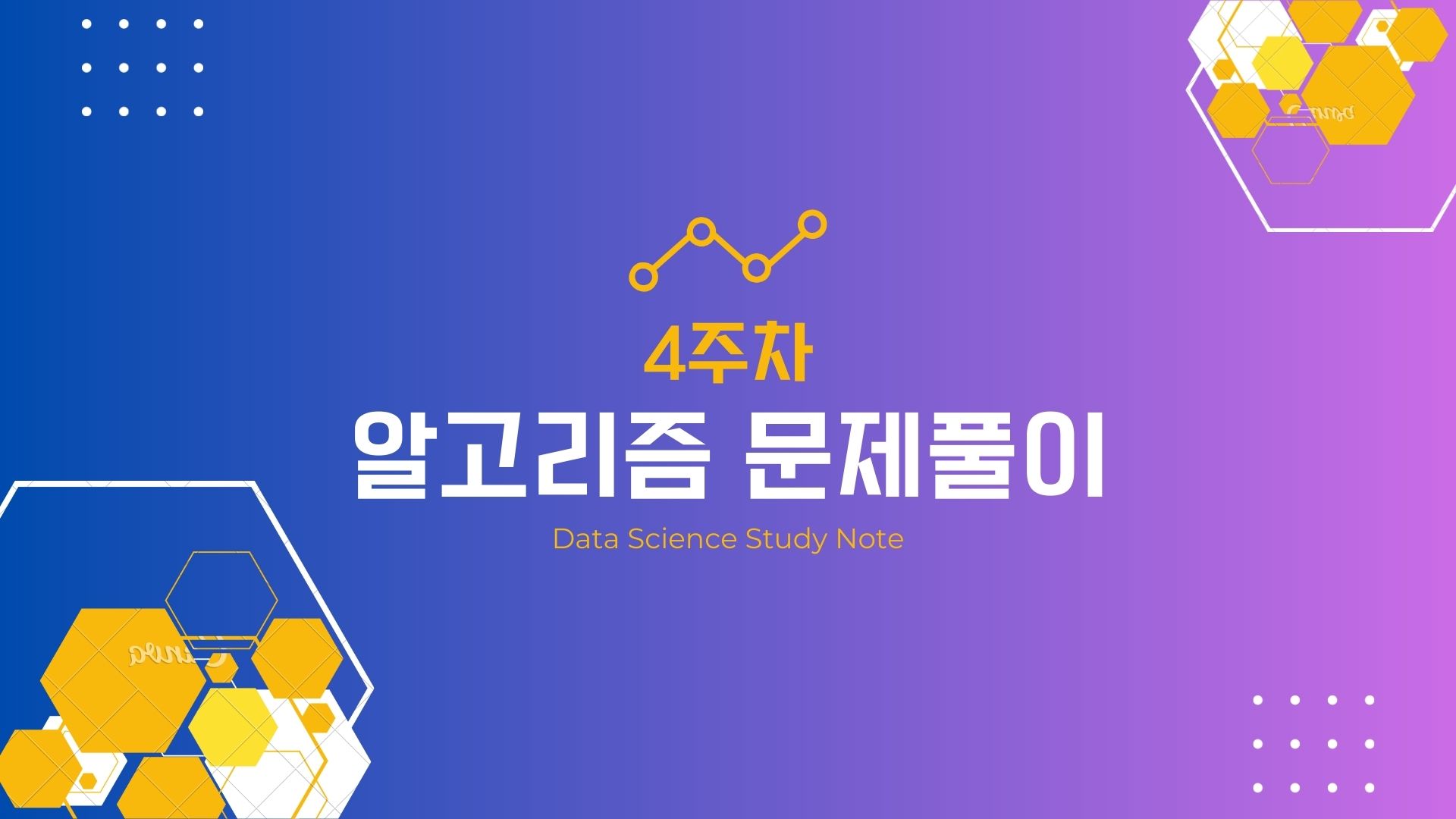
1. 최댓값(1)

(maxMod.py)
class MaxAlgorithm:
def __init__(self, ns):
self.nums = ns
self.maxNum = 0
self.maxNumCnt = 0
def setMaxNum(self):
self.maxNum = 0
for n in self.nums:
if self.maxNum < n:
self.maxNum = n
def getMaxNum(self):
self.setMaxNum()
return self.maxNum
def setMaxNumCnt(self):
self.setMaxNum()
for n in self.nums:
if self.maxNum == n:
self.maxNumCnt += 1
def getMaxNumCnt(self):
self.setMaxNumCnt()
return self.maxNumCnt
(ex.py)
import random
import maxMod
if __name__ == '__main__':
nums = []
for n in range(30):
nums.append(random.randint(1,50))
print(f'nums: \n {nums}')
ma = maxMod.MaxAlgorithm(nums)
print(f'max num: {ma.getMaxNum()}')
print(f'max num count: {ma.getMaxNumCnt()}')

2. 최댓값(2)

(mod.py)
def getAvg(ns):
total = 0
for n in ns:
total += n
return total / len(ns)
def getMax(ns):
maxN = ns[0]
for n in ns:
if maxN < n:
maxN = n
return maxN
def getDeviation(n1,n2):
return abs(n1-n2)
(ex.py)
import mod
import random
scores = random.sample(range(50,100),20)
scores_avg = mod.getAvg(scores)
scores_max = mod.getMax(scores)
deviation = mod.getDeviation(scores_avg, scores_max)
print(f'scores_avg: {scores_avg}')
print(f'scores_max: {scores_max}')
print(f'deviation: {deviation}')
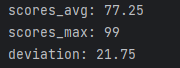
- 최솟값(1)

(minMod.py)
class MinAlgorithm:
def __init__(self, ns):
self.nums = ns
self.minNum = 0
self.minNumCnt = 0
def setMinNum(self):
self.minNum = 51
for n in self.nums:
if self.minNum > n:
self.minNum = n
def getMinNum(self):
self.setMinNum()
return self.minNum
def setMinNumCnt(self):
self.setMinNum()
for n in self.nums:
if self.minNum == n:
self.minNumCnt += 1
def getMinNumCnt(self):
self.setMinNumCnt()
return self.minNumCnt
(ex.py)
import random
import minMod
if __name__ == '__main__':
nums = []
for n in range(30):
nums.append(random.randint(1,50))
print(f'nums: \n {nums}')
ma = minMod.MinAlgorithm(nums)
print(f'min num: {ma.getMinNum()}')
print(f'min num count: {ma.getMinNumCnt()}')

4. 최솟값(2)

(mod.py)
def getAvg(ns):
total = 0
for n in ns:
total += n
return total / len(ns)
def getMin(ns):
minN = ns[0]
for n in ns:
if minN > n:
minN = n
return minN
def getDeviation(n1, n2):
return round(abs(n1-n2), 2)
def getMaxOrMin(ns, maxFlag = True):
resultN = ns[0]
for n in ns:
if maxFlag:
if resultN < n:
resultN = n
else:
if resultN > n:
resultN = n
return resultN
(mod2.py)
class ScoreManagement:
def __init__(self, ss):
self.scores = ss
self.score_tot = 0
self.score_avg = 0
self.score_min = 0
self.score_max = 0
def getMinScore(self):
if self.scores == None or len(self.scores) == 0:
return None
self.score_min = self.scores[0]
for score in self.scores:
if self.score_min > score:
self.score_min = score
return self.score_min
def getMaxScore(self):
if self.scores == None or len(self.scores) == 0:
return None
self.score_max = self.scores[0]
for score in self.scores:
if self.score_max < score:
self.score_max = score
return self.score_max
def getTotScore(self):
if self.scores == None or len(self.scores) == 0:
return None
self.score_tot = 0
for score in self.scores:
self.score_tot += score
return self.score_tot
def getAvgScore(self):
if self.scores == None or len(self.scores) == 0:
return None
self.score_avg = round(self.getTotScore() / len(self.scores), 2)
return self.score_avg
def getMaxDeviation(self):
result = abs(self.getAvgScore() - self.getMaxScore())
return round(result, 2)
def getMinDeviation(self):
result = abs(self.getAvgScore() - self.getMinScore())
return round(result, 2)
(ex.py)
import mod
import random
scores = random.sample(range(50,100),20)
scores_avg = mod.getAvg(scores)
scores_min = mod.getMaxOrMin(scores, maxFlag=False)
scores_max = mod.getMaxOrMin(scores, maxFlag=True)
print(f'scores_avg: {scores_avg}')
print(f'scores_min: {scores_min}')
print(f'scores_max: {scores_max}')
import mod2
sm = mod2.ScoreManagement(scores)
print(f'score_avg: {sm.getAvgScore()}')
print(f'score_min: {sm.getMinScore()}')
print(f'score_max: {sm.getMaxScore()}')
print(f'score_min_deviation: {sm.getMinDeviation()}')
print(f'score_max_deviation: {sm.getMaxDeviation()}')
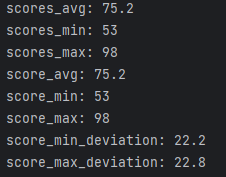
5. 최빈값(1)

(maxMod.py)
class MaxAlgorithm:
def __init__(self, ns):
self.nums = ns
self.maxNum = 0
self.maxNumIdx = 0
def setMaxIdxAndNum(self):
self.maxNum = 0
self.maxNumIdx = 0
for i, n in enumerate(self.nums):
if self.maxNum < n:
self.maxNum = n
self.maxNumIdx = i
def getMaxNum(self):
return self.maxNum
def getMaxIdx(self):
return self.maxNumIdx
(modeMod.py)
import maxMod
class ModeAlgorithm:
def __init__(self, ns, mn):
self.nums = ns
self.maxNum = mn
self.indexes = []
def setIndexList(self):
self.indexes = [0 for i in range(self.maxNum+1)]
for n in self.nums:
self.indexes[n] = self.indexes[n] + 1
def getIndexList(self):
if sum(self.indexes) == 0:
return None
else:
return self.indexes
def printAges(self):
n = 1
while True:
maxAlo = maxMod.MaxAlgorithm(self.indexes)
maxAlo.setMaxIdxAndNum()
maxNum = maxAlo.getMaxNum()
maxNumIdx = maxAlo.getMaxIdx()
if maxNum == 0:
break
print(f'[{n:0>3}] {maxNumIdx}세 빈도수: {maxNum}', end="")
print('+'*maxNum)
self.indexes[maxNumIdx]=0
n += 1
(ex.py)
import random
import modeMod
import maxMod
ages = [random.randint(20, 50) for _ in range(40)]
print(f'ages: {ages}')
print(f'employee cnt: {len(ages)}명')
maxAlo = maxMod.MaxAlgorithm(ages)
maxAlo.setMaxIdxAndNum()
maxAge = maxAlo.getMaxNum()
print(f'maxAge: {maxAge}세')
modAlo = modeMod.ModeAlgorithm(ages, maxAge)
modAlo.setIndexList()
print(f'IndexList: {modAlo.getIndexList()}')
modAlo.printAges()
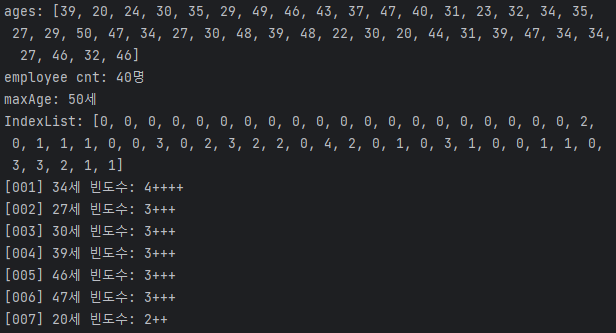
6. 최빈값(2)

(mod.py)
class LottoMode:
def __init__(self, ln):
self.lottoNums = ln
self.modeList = [0 for n in range(1, 47)]
def getLottoNumMode(self):
for roundNums in self.lottoNums:
for num in roundNums:
self.modeList[num] = self.modeList[num] + 1
return self.modeList
def printModeList(self):
if sum(self.modeList) == 0:
return None
for i, m in enumerate(self.modeList):
if i != 0:
print(f'번호: {i:0>2}, 빈도: {m}, {"*"*m}')
(ex.py)
import mod
import random
lottery_numbers = [] # 로또번호를 저장할 리스트
for draw in range(1, 31):
numbers = random.sample(range(1, 46), 6)
numbers.sort()
lottery_numbers.append(numbers)
print(lottery_numbers)
lm = mod.LottoMode(lottery_numbers)
mList = lm.getLottoNumMode()
print(f'mList: {mList}')
lm.printModeList()
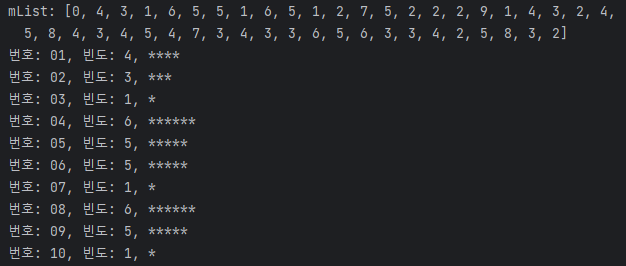
7. 근삿값(1)

(near.py)
class NearAlgorithm:
def __init__(self, d):
self.temps = {0:24, 5:22, 10:20, 15:16, 20:13, 25:10, 30:6}
self.depth = d
self.nearNum = 0
self.minNum = 24
def getNearNumbers(self):
for n in self.temps.keys():
absNum = abs(n - self.depth)
if absNum < self.minNum:
self.minNum = absNum
self.nearNum = n
return self.temps[self.nearNum]
(ex.py)
import near
depth = int(float(input('input depth: ')))
print(f'depth: {depth}m')
na = near.NearAlgorithm(depth)
temp = na.getNearNumbers()
print(f'water temperature: {temp}도')
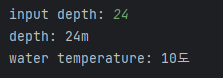
8. 근삿값(2)
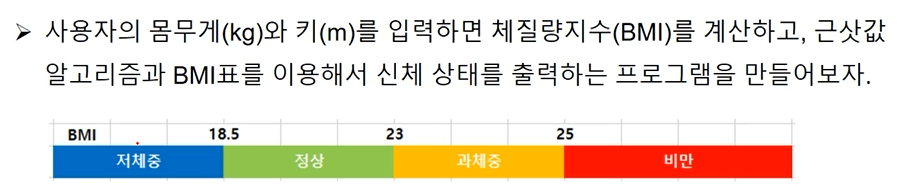
(nearMod.py)
class BmiAlgorithm:
def __init__(self, w, h):
self.BMISection={18.5:['저체중','정상'], 23:['정상','과체중'], 25:['과체중','비만']}
self.userWeight = w
self.userHeight = h
self.userBMI = 0
self.userCondition=''
self.nearNum = 0
self.minNum = 25
def calculatorBMI(self):
self.userBMI = round(self.userWeight / (self.userHeight ** 2), 2)
print(f'self.userBMI: {self.userBMI}')
def printUserCondition(self):
for n in self.BMISection.keys():
absNum = abs(n - self.userBMI)
if absNum < self.minNum:
self.minNum = absNum
self.nearNum = n
print(f'self.nearNum: {self.nearNum}')
if self.userBMI < self.nearNum:
self.userCondition = self.BMISection[self.nearNum][0]
else:
self.userCondition = self.BMISection[self.nearNum][1]
print(f'self.userCondition: {self.userCondition}')
(ex.py)
import nearMod
uWeight = float(input('input weight(kg): '))
uHeight = float(input('input height(m): '))
na = nearMod.BmiAlgorithm(uWeight, uHeight)
na.calculatorBMI()
na.printUserCondition()
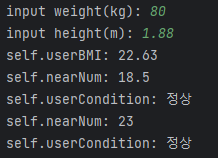
9. 재귀(1)

(ex.py)
sales = [12000,13000,12500,11000,10500,98000,91000,91500,10500,11500,12000,12500]
def salesUpAndDown(ss):
if len(ss) == 1:
return ss
print(f'salse: {ss}')
currentSales = ss.pop(0)
nextSales = ss[0]
increase = nextSales - currentSales
if increase > 0:
increase = '+' + str(increase)
print(f'매출 증감액: {increase}')
return salesUpAndDown(ss)
if __name__ == '__main__':
salesUpAndDown(sales)
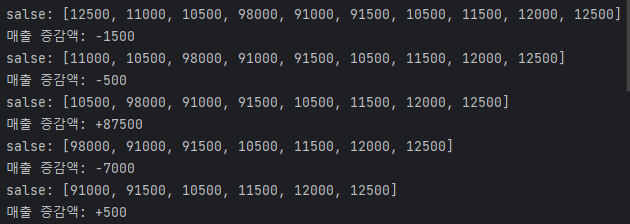
10. 재귀(2)

(mod.py)
class NumsSum:
def __init__(self, n1, n2):
self.bigNum = 0
self.smallNum = 0
self.setN1N2(n1, n2)
def setN1N2(self, n1, n2):
self.bigNum = n1
self.smallNum = n2
if n1 < n2:
self.bigNum = n2
self.smallNum = n1
else:
self.bigNum = n1
self.smallNum = n2
def addNum(self, n):
if n <= 1:
return n
return n + self.addNum(n-1)
def sumBetweenNums(self):
return self.addNum(self.bigNum-1) - self.addNum(self.smallNum)
(ex.py)
import mod
num1 = int(input(f'input number1: '))
num2 = int(input(f'input number2: '))
ns = mod.NumsSum(num1, num2)
result = ns.sumBetweenNums()
print(f'result: {result}')
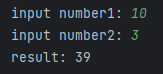
11. 평균(1)
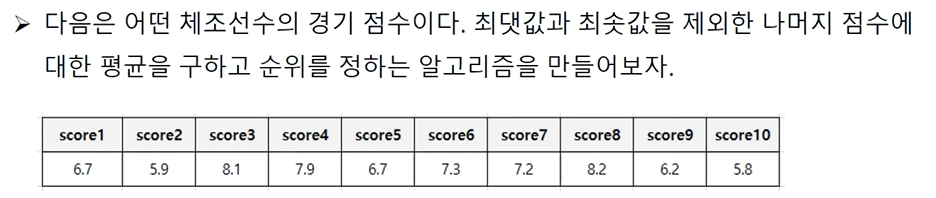
(minAlgorithm.py)
class MinAlgorithm:
def __init__(self, ss):
self.scores = ss
self.minScore = 0
self.minIdx = 0
def removeMinScore(self):
self.minScore = self.scores[0]
for i, s in enumerate(self.scores):
if self.minScore > s:
self.maincore = s
self.minIdx = i
print(f'self.minScore: {self.minScore}')
print(f'self.minIdx: {self.minIdx}')
self.scores.pop(self.minIdx)
print(f'scores: {self.scores}')
(maxAlgorithm.py)
class MaxAlgorithm:
def __init__(self, ss):
self.scores = ss
self.maxScore = 0
self.maxIdx = 0
def removeMaxScore(self):
self.maxScore = self.scores[0]
for i, s in enumerate(self.scores):
if self.maxScore < s:
self.maxScore = s
self.maxIdx = i
print(f'self.maxScore: {self.maxScore}')
print(f'self.maxIdx: {self.maxIdx}')
self.scores.pop(self.maxIdx)
print(f'scores: {self.scores}')
(nearAlgorithm.py)
class Top5Players:
def __init__(self, cts, ns):
self.currentScores = cts
self.newScore = ns
def setAlignScore(self):
nearIdx = 0
minNum = 10.0
for i, s in enumerate(self.currentScores):
absNum = abs(self.newScore - s)
if absNum < minNum:
minNum = absNum
nearIdx = i
if self.newScore >= self.currentScores[nearIdx]:
for i in range(len(self.currentScores)-1, nearIdx, -1):
self.currentScores[i] = self.currentScores[i-1]
self.currentScores[nearIdx] = self.newScore
else:
for i in range(len(self.currentScores)-1, nearIdx+1, -1):
self.currentScores[i] = self.currentScores[i-1]
self.currentScores[nearIdx+1] = self.newScore
def getFinalTop5Scores(self):
return self.currentScores
(ex.py)
import maxAlgorithm, minAlgorithm, nearAlgorithm
top5Scores = [9.12, 8.95, 8.12, 6.90, 6.18]
scores = [6.7, 5.9, 8.1, 7.9, 6.7, 7.3, 7.2, 8.2, 6.2, 5.8]
print(f'scores: {scores}')
maxA = maxAlgorithm.MaxAlgorithm(scores)
maxA.removeMaxScore()
minA = minAlgorithm.MinAlgorithm(scores)
minA.removeMinScore()
total = 0
average = 0
for n in scores:
total += n
average = round(total / len(scores), 2)
print(f'total: {round(total, 2)}')
print(f'average: {average}')
tp = nearAlgorithm.Top5Players(top5Scores, average)
tp.setAlignScore()
top5Scores = tp.getFinalTop5Scores()
print(f'top5scores: {top5Scores}')
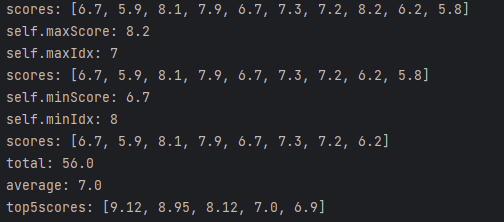