24일차 과제 링크 👉 24일차 과제
class MyClass {
final int value;
// 기본 생성자
MyClass(this.value);
// factory 생성자
// 문자열을 받고 그 문자열으로 객체를 생성하여 반환
factory MyClass.fromString(String str) {
final parts = str.split('-');
final value = int.parse(parts.last);
// 객체를 직접 생성하지 않고 이미 생성된 객체를 반환하는 방식으로 동작함
return MyClass(value);
}
}
Person 클래스
class Person { String name; int age; Person(this.name, this.age); }
import 'dart:convert';
Person person = Person('John', 30);
String jsonStr = jsonEncode(person);
print(jsonStr); // {"name":"John","age":30}
import 'dart:convert';
String jsonStr = '{"name":"John","age":30}';
Person person = Person.fromJson(jsonDecode(jsonStr));
print(person.name); // John
print(person.age); // 30
class Person {
String name;
int age;
Person(this.name, this.age);
factory Person.fromJson(Map<String, dynamic> json) {
return Person(json['name'], json['age']);
}
}
{
"data": [
"Julius Ceasar, Henri II, Charles XI, and Napoleon were all afraid of cats."
]
}
import 'package:dio/dio.dart';
class MeowFact {
List<String> data;
MeowFact({required this.data});
factory MeowFact.fromMap(Map<String, dynamic> map) {
var data = List<String>.from(map['data']);
return MeowFact(data: data);
}
String toString() => 'MeowFact($data)';
}
void main() async {
Dio dio = Dio();
var url = 'https://meowfacts.herokuapp.com/';
for (int i=0; i<10; i++) {
var res = await dio.get(url);
if (res.statusCode == 200) {
var fact1 = MeowFact.fromMap(res.data);
print(fact1);
}
}
}
{
"fact": "Cats are extremely sensitive to vibrations. Cats are said to detect earthquake tremors 10 or 15 minutes before humans can.",
"length": 122
}
import 'package:dio/dio.dart';
class CatFact {
String fact;
int length;
CatFact({required this.fact, required this.length});
factory CatFact.fromMap(Map<String, dynamic> map) {
return CatFact(fact: map['fact'], length: map['length']);
}
factory CatFact.fromFact(String fact) {
return CatFact(fact: fact, length: fact.length);
}
String toString() => 'CatFact(fact: $fact, length: $length)';
}
void main() async {
Dio dio = Dio();
var url = 'https://catfact.ninja/fact';
var res = await dio.get(url);
if (res.statusCode == 200) {
var catFact = CatFact.fromMap(res.data);
print(catFact);
var data = CatFact.fromFact(res.data['fact']);
print(data);
}
}
{
"slip": {
"id": 115,
"advice": "One of the top five regrets people have is that they didn't have the courage to be their true self."
}
}
import 'dart:convert';
import 'package:dio/dio.dart';
class Slip {
int id;
String advice;
Slip({required this.id, required this.advice});
factory Slip.fromMap(Map<String, dynamic> map) {
return Slip(id: map['id'], advice: map['advice']);
}
toString() => advice;
}
void main() async {
Dio dio = Dio();
var url = 'https://api.adviceslip.com/advice';
var res = await dio.get(url);
if (res.statusCode == 200) {
var myData = jsonDecode(res.data);
var slipData = Slip.fromMap(myData['slip']);
print(slipData);
}
}
{
"activity": "Play a volleyball match with some friends",
"type": "social",
"participants": 4,
"price": 0,
"link": "",
"key": "4306710",
"accessibility": 0.3
}
import 'package:dio/dio.dart';
class Activity {
String activity;
String type;
int participants;
double price;
String link;
String key;
double accessibility;
Activity({
required this.activity,
required this.type,
required this.participants,
required this.price,
required this.link,
required this.key,
required this.accessibility,
});
factory Activity.fromBoardApi(Map<String, dynamic> map) {
return Activity(
activity: map['activity'],
type: map['type'],
participants: map['participants'],
price: map['price'] is int ? double.parse(map['price']) : map['price'],
link: map['link'],
key: map['key'],
accessibility: map['accessibility'],
);
}
String toString() {
return 'Activity(activity: $activity, type: $type, participants: $participants, price: $price)';
}
}
void main() async {
Dio dio = Dio();
var url = 'https://www.boredapi.com/api/activity';
var res = await dio.get(url);
if (res.statusCode == 200) {
var activity = Activity.fromBoardApi(res.data);
print(activity);
}
}
```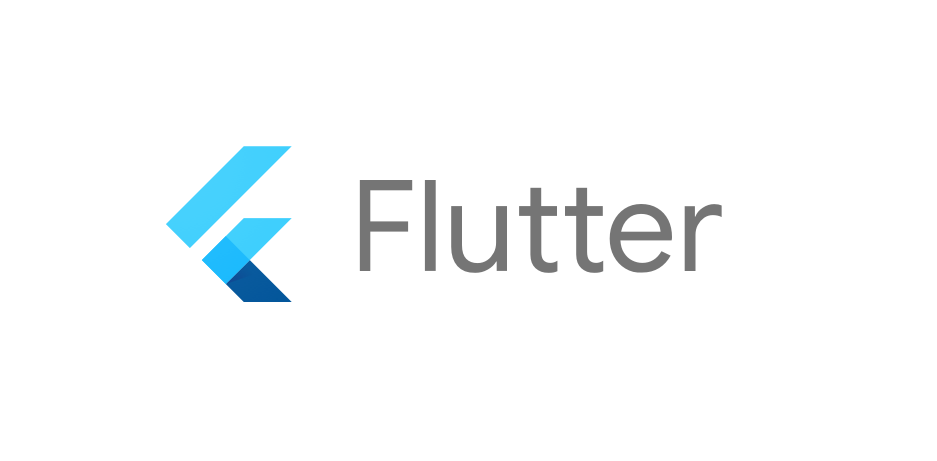