📝 입출력을 위한 포맷
- cout을 이용한 입출력 시 포맷 지정 가능
- 포맷 입출력 방법 3가지
📝포맷 플래그
- 입출력 스트림에서 입출력 형시을 지정하기 위한 ios클래스의 플래그
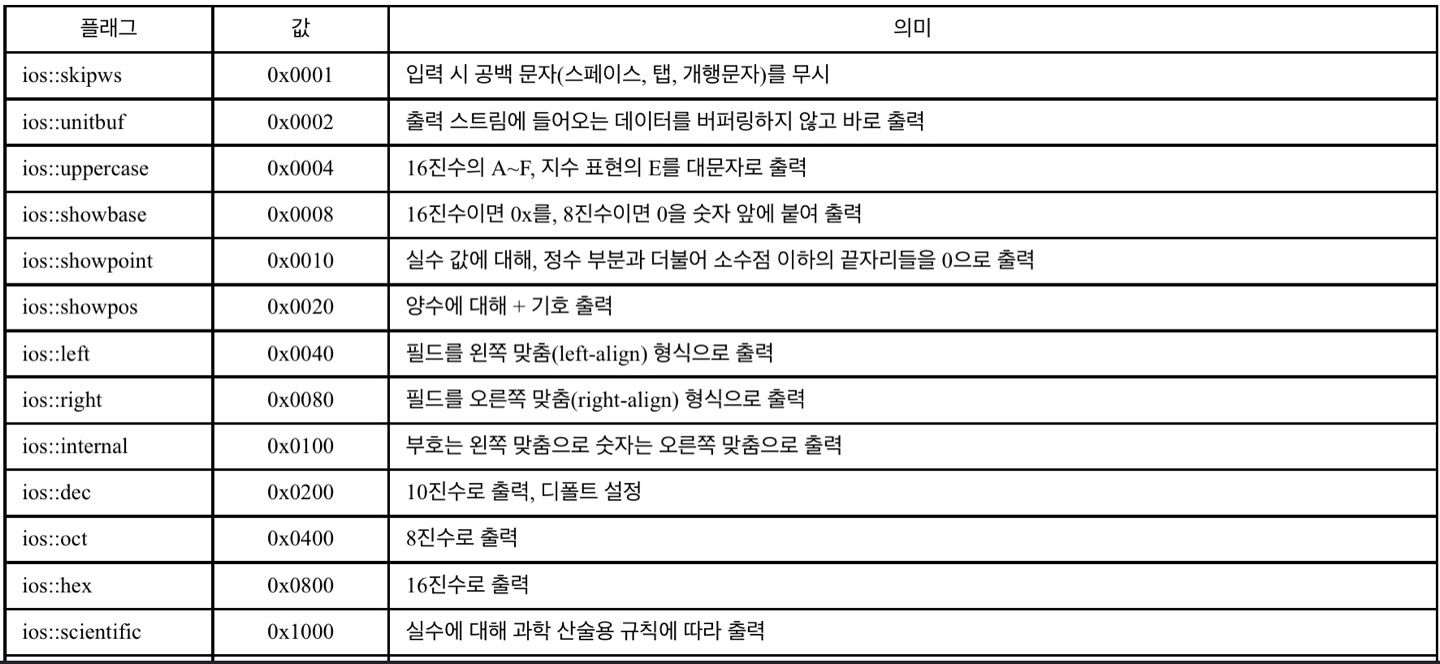
#include <iostream>
using namespace std;
int main() {
cout << 30 << endl;
cout.unsetf(ios::dec);
cout.setf(ios::hex);
cout << 30 << endl;
cout.setf(ios::showbase);
cout << 30 << endl;
cout.setf(ios::dec | ios::showpoint);
cout << 23.5 << endl;
}
📝포맷 함수
- 포맷을 변경하는 함수
- int width(int minWidth)
- 출력되는 필드의 최소 너비를 minWidth로 설정하고 이전 설정을 반환
- char fill(char cFill)
- 필드의 빈칸을 cFill 문자로 채우도록 설정하고 이전 설정을 반환
- int precision(int np)
- 출력되는 수의 유효 숫자 자리수를 np개로 설정, 소수점을 제외
#include <iostream>
using namespace std;
void showWidth() {
cout.width(10);
cout << "Hello" << endl;
cout.width(5);
cout << 12 << endl;
cout << '%';
cout.width(10);
cout << "Korea/" << "Seoul/" << "City" << endl;
}
int main() {
showWidth();
cout << endl;
cout.fill('^');
showWidth();
cout << endl;
cout.precision(5);
cout << 11. / 3. << endl;
}
📝조작자
- manipulator, 스트림 조작자
- 조작자는 함수
- C++ 표준 라이브러리에 구현된 조작자 : 입출력 포맷 지정 목적
- 개발자만의 조작자 작성 가능 : 다양한 목적
- 매개 변수 없는 조작자와 매개 변수를 가진 조작자로 구분
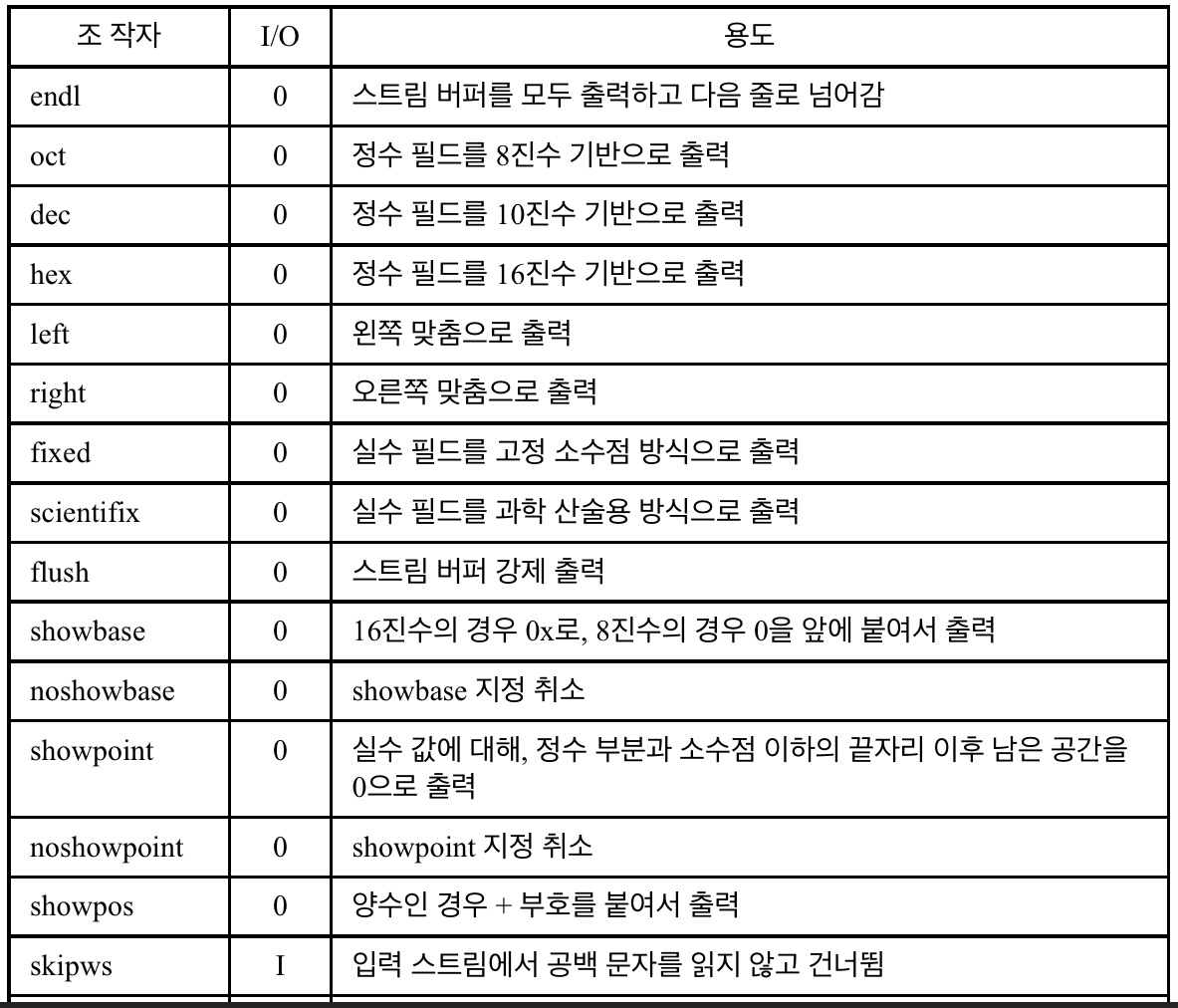
#include <iostream>
#include <iomanip>
using namespace std;
int main() {
cout << showbase;
cout << setw(8) << "Number";
cout << setw(10) << "Octal";
cout << setw(10) << "Hexa" << endl;
for (int i = 0; i < 50; i += 5) {
cout << setw(8) << setfill('.') <<dec<< i;
cout << setw(10) << setfill('.') << oct << i;
cout << setw(10) << setfill('.') << hex << i << endl;
}
}