Containers with children size themselved to thier children.
- Container Widget 은 무조건 페이지 내에서 최대한의 공간을 차지하려고함
- 컨테이너는 오직 하나의 child 만 가짐
- 하위에 child 를 두고 Text 를 작성해보면, Container Widget 해당 Text 의 크기에 맞춰짐
SafeArea
: 텍스트가 화면 밖을 나가는 것을 방지하기 위해 경계를 지정해주는 것
SafeArea 적용X | SafeArea 적용O |
---|
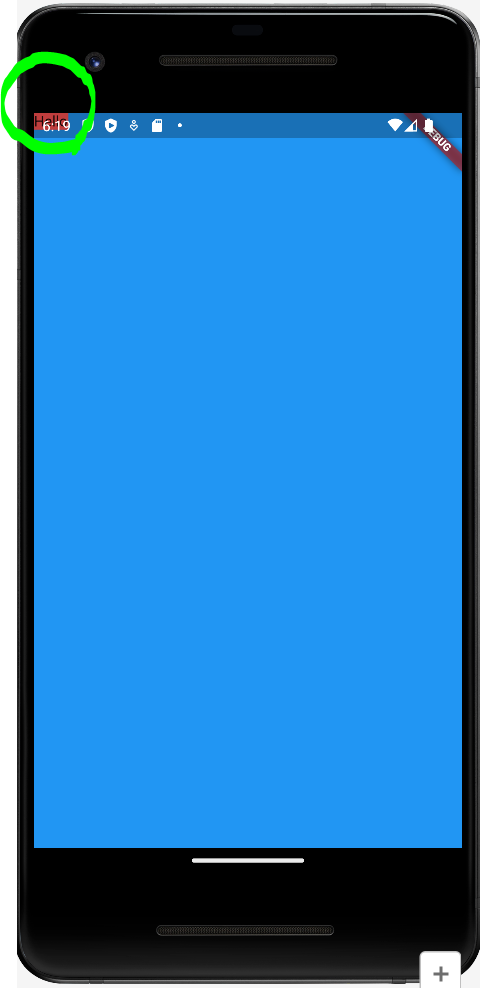 | 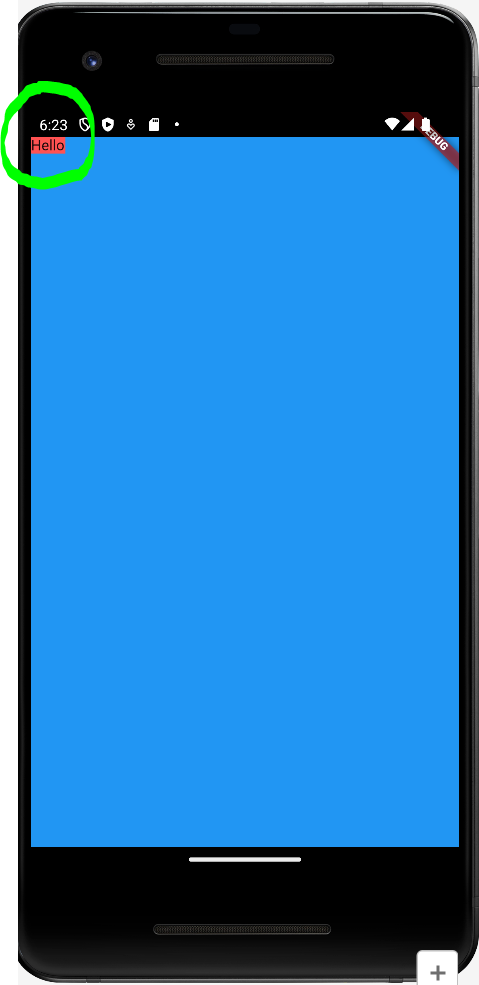 |
margin
: 컨테이너가 스크린에 가장자리에서 일정 간격을 가지게 하고 싶을 때 사용
padding
: 그 컨테이너가 포함하고 있는 요소가 컨테이너에 가장자리에서 일정 간격을 가지게 하고 싶을 때 사용
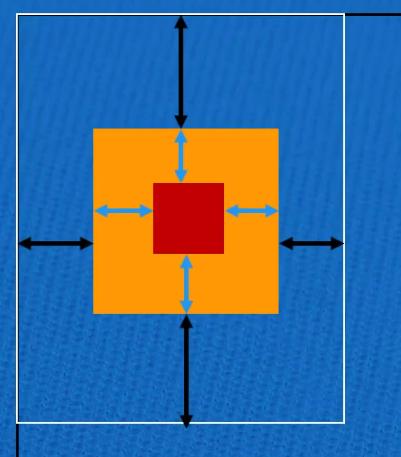
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Myapp',
theme: ThemeData(primaryColor: Colors.blue),
home: MyPage(),
);
}
}
class MyPage extends StatelessWidget {
const MyPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.blue,
body: Container(
color: Colors.redAccent,
child: Text('Hello'),
),
);
}
}
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Myapp',
theme: ThemeData(primaryColor: Colors.blue),
home: MyPage(),
);
}
}
class MyPage extends StatelessWidget {
const MyPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.blue,
body: SafeArea(
child: Container(
color: Colors.redAccent,
width: 100,
height: 100,
margin: EdgeInsets.symmetric(
vertical: 80,
horizontal: 20,
),
padding: EdgeInsets.all(20),
child: Text('Hello'),
),
),
);
}
}
mainAxisAlignment: MainAxisAlignment.center
-> 컬럼을 화면 가운데 위치하도록 하는 속성
mainAxisSize: MainAxisSize.min
-> 컬럼의 사이즈를 최소크기로 지정
verticalDirection: VerticalDirection.up
-> 컬럼 안의 요소들 내림차순 정렬해 화면에 보여줌
class MyPage extends StatelessWidget {
const MyPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.teal,
body: SafeArea(
child: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
verticalDirection: VerticalDirection.up,
children: <Widget>[
Container(
width: 100,
height: 100,
color: Colors.white,
child: Text('Container 1'),
),
Container(
width: 100,
height: 100,
color: Colors.red,
child: Text('Container 2'),
),
Container(
width: 100,
height: 100,
color: Colors.blue,
child: Text('Container 3'),
),
],
),
),
),
);
}
}
verticalDirection: VerticalDirection.down | verticalDirection: VerticalDirection.up |
---|
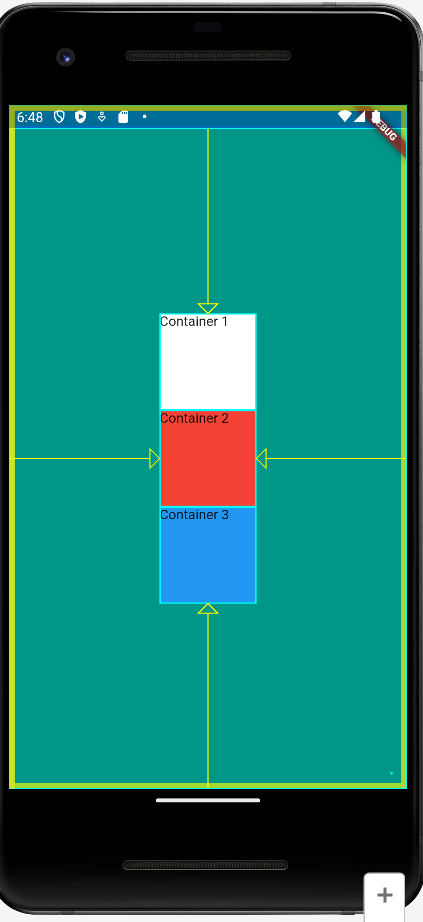 | 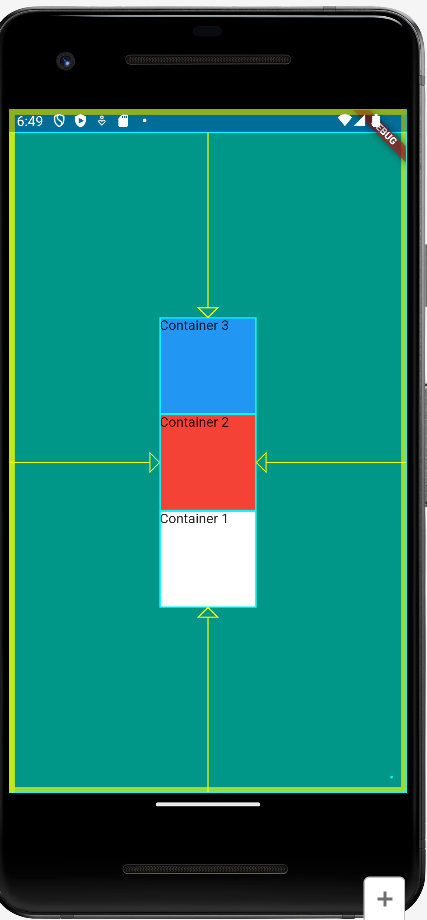 |
mainAxisAlignment: MainAxisAlignment.spaceEvenly | mainAxisAlignment: MainAxisAlignment.spaceBetween |
---|
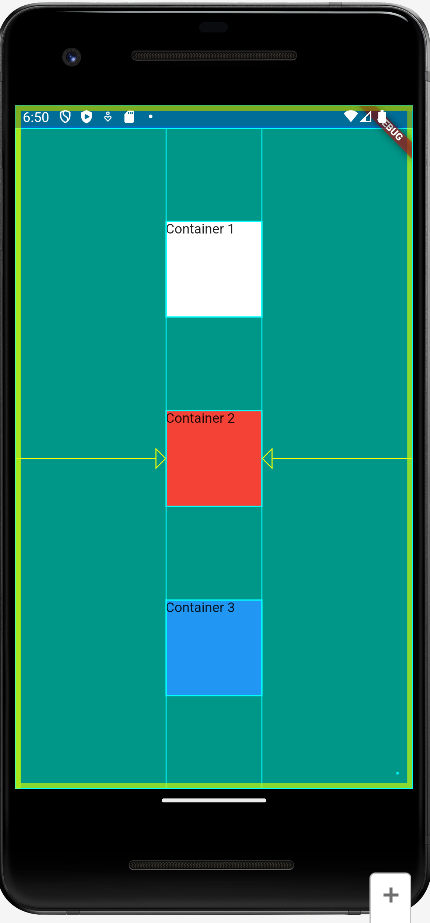 | 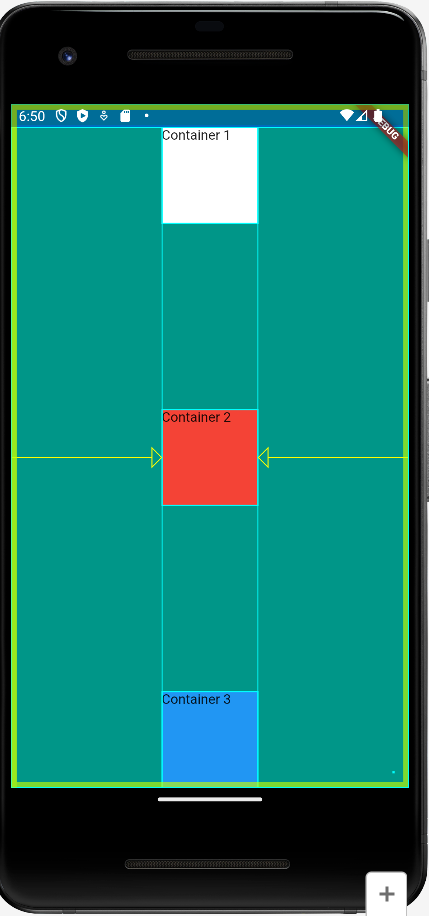 |
crossAxisAlignment: CrossAxisAlignment.end
-> 컬럼을 오른쪽 끝에 배치
Container(
width: double.infinity,
),
class MyPage extends StatelessWidget {
const MyPage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.teal,
body: SafeArea(
child: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Container(
height: 100,
color: Colors.white,
child: Text('Container 1'),
),
SizedBox(
height: 30.0,
),
Container(
height: 100,
color: Colors.red,
child: Text('Container 2'),
),
Container(
height: 100,
color: Colors.blue,
child: Text('Container 3'),
),
],
),
),
),
);
}
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.teal,
body: SafeArea(
child: Center(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: <Widget>[
Container(
height: 100,
color: Colors.white,
child: Text('Container 1'),
),
SizedBox(
height: 30.0,
),
Container(
height: 100,
color: Colors.red,
child: Text('Container 2'),
),
Container(
height: 100,
color: Colors.blue,
child: Text('Container 3'),
),
],
),
),
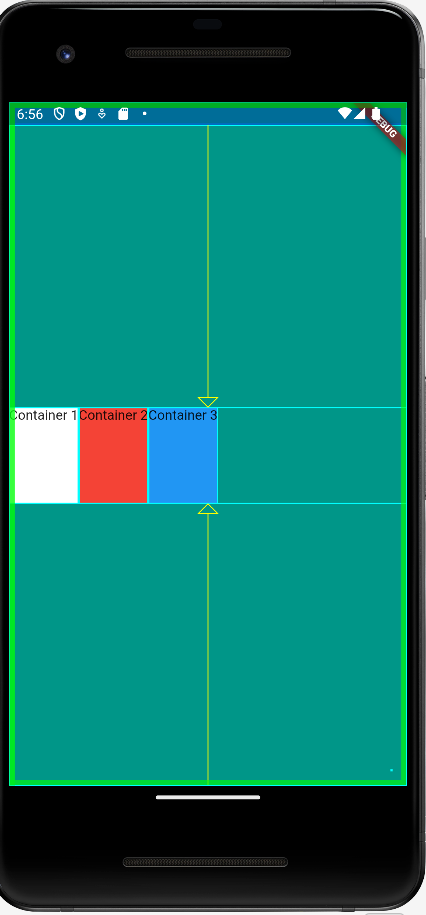
📚 코딩셰프 < 플러터(flutter) 순한맛 강좌 >