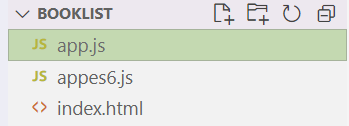
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/skeleton/2.0.4/skeleton.min.css" />
<title>북 리스트</title>
<style>
.success,
.error {
color: white;
padding: 5px;
margin: 5px 0 15px 0px;
}
.success {
background-color: green;
}
.error {
background-color: red;
}
</style>
</head>
<body>
<div class="container">
<h1>Add Book</h1>
<form action="" id="book-form">
<div>
<label for="title">제 목</label>
<input type="text" id="title" class="u-full-width" />
</div>
<div>
<label for="author">저 자</label>
<input type="text" id="author" class="u-full-width" />
</div>
<div>
<label for="isbn">도서번호</label>
<input type="text" id="isbn" class="u-full-width" />
</div>
<div>
<input type="submit" value="추 가" class="u-full-width" />
</div>
</form>
<hr />
<table class="u-full-width">
<thead>
<tr>
<th>제 목</th>
<th>저 자</th>
<th>도서번호</th>
<th></th>
</tr>
</thead>
<tbody id="book-list"></tbody>
</table>
</div>
<script src="app.js"></script>
</body>
</html>
// 책 생성자
function Book(title, author, isbn) {
this.title = title;
this.author = author;
this.isbn = isbn;
}
// UI 생성자
function UI() {}
// UI 에 book 추가 메소드 추가
UI.prototype.addBookToList = function (book) {
const list = document.getElementById('book-list');
const row = document.createElement('tr');
row.innerHTML = `
<td>${book.title}</td>
<td>${book.author}</td>
<td>${book.isbn}</td>
<td><a href="#" class="delete">X</td>
`;
list.appendChild(row);
};
// 입력창을 클리어
UI.prototype.clearFields = function () {
document.getElementById('title').value = '';
document.getElementById('author').value = '';
document.getElementById('isbn').value = '';
};
// 상태 메세지 출력
UI.prototype.showAlert = function (message, className) {
// Create div
const div = document.createElement('div');
// Add classes
div.className = `alert ${className}`;
// Add text
div.appendChild(document.createTextNode(message));
// Get parent
const container = document.querySelector('.container');
// Get form
const form = document.querySelector('#book-form');
// Insert alert
container.insertBefore(div, form);
// Timeout after 3 sec
setTimeout(function () {
document.querySelector('.alert').remove();
}, 3000);
};
// 이벤트 리스너
document.getElementById('book-form').addEventListener('submit', function (e) {
const title = document.getElementById('title').value,
author = document.getElementById('author').value,
isbn = document.getElementById('isbn').value;
const book = new Book(title, author, isbn);
const ui = new UI();
// validate
if (title === '' || author === '' || isbn === '') {
ui.showAlert('내용을 입력해 주세요', 'error');
} else {
ui.addBookToList(book);
ui.showAlert('추가되었습니다.', 'success');
ui.clearFields();
}
e.preventDefault();
});