import android.os.Bundle
import androidx.activity.ComponentActivity
import androidx.activity.compose.setContent
import androidx.annotation.DrawableRes
import androidx.compose.foundation.Image
import androidx.compose.foundation.layout.Column
import androidx.compose.foundation.layout.Spacer
import androidx.compose.foundation.layout.fillMaxSize
import androidx.compose.foundation.layout.padding
import androidx.compose.foundation.layout.size
import androidx.compose.foundation.lazy.LazyColumn
import androidx.compose.foundation.lazy.items
import androidx.compose.material3.Card
import androidx.compose.material3.CardDefaults
import androidx.compose.material3.MaterialTheme
import androidx.compose.material3.Surface
import androidx.compose.material3.Text
import androidx.compose.runtime.Composable
import androidx.compose.ui.Modifier
import androidx.compose.ui.res.painterResource
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import com.example.compose_example.ui.theme.Compose_exampleTheme
class MainActivity : ComponentActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
Compose_exampleTheme {
Surface(
modifier = Modifier.fillMaxSize(),
color = MaterialTheme.colorScheme.background
) {
Catalog(items)
}
}
}
}
}
@Composable
fun CatalogItem(itemData: ItemData) {
Card(
elevation = CardDefaults.cardElevation(8.dp),
modifier = Modifier.padding(16.dp),
) {
Column (
modifier = Modifier.padding(8.dp)
) {
Image(
painter = painterResource(id = itemData.imageId),
contentDescription = itemData.title
)
Spacer(modifier = Modifier.size(8.dp))
Text(text = itemData.title, style = MaterialTheme.typography.headlineMedium)
Spacer(modifier = Modifier.size(8.dp))
Text(text = itemData.description)
}
}
}
@Preview(showBackground = true)
@Composable
fun ItemPreview() {
Compose_exampleTheme {
CatalogItem(
ItemData(
imageId = R.drawable.a1,
title = "스테이크",
description = "고기를 고열로 구워내어 외부는 바삭하고 내부는 부드러운 고기 요리입니다. 소금과 후추로 간을 해서 고기의 풍미를 살려보세요."
),
)
}
}
@Composable
fun Catalog(itemList: List<ItemData>) {
LazyColumn {
items(itemList) {item ->
CatalogItem(item)
}
}
}
@Preview(showBackground = true)
@Composable
fun DefaultPreview() {
Compose_exampleTheme {
Catalog(items)
}
}
data class ItemData(
@DrawableRes val imageId: Int,
val title: String,
val description: String,
)
val items = listOf(
ItemData(
imageId = R.drawable.a1,
title = "스테이크",
description = "고기를 고열로 구워내어 외부는 바삭하고 내부는 부드러운 고기 요리입니다. 소금과 후추로 간을 해서 고기의 풍미를 살려보세요."
),
ItemData(
imageId = R.drawable.a2,
title = "스시",
description = "신선한 생선과 쌀로 만든 밥을 함께 먹는 일본의 대표적인 음식입니다. 간장과 와사비, 생강을 곁들여 드세요."
),
ItemData(
imageId = R.drawable.a3,
title = "파스타",
description = "면과 소스를 함께 끓여내어 어우러진 이탈리아의 대표 음식입니다. 알 따라서 다양한 종류의 파스타와 소스를 시도해보세요."
),
ItemData(
imageId = R.drawable.a4,
title = "피자",
description = "바삭한 반죽 위에 토마토 소스와 치즈, 다양한 토핑을 얹어 구워내는 이탈리아의 대표 음식입니다. 오븐에서 구워내어 즐겨보세요."
),
ItemData(
imageId = R.drawable.a5,
title = "초밥",
description = "생선과 다양한 재료를 살짝 얹은 쌀밥 위에 싸먹는 일본의 전통 음식입니다. 신선한 재료와 간장을 묻혀 드세요."
)
)
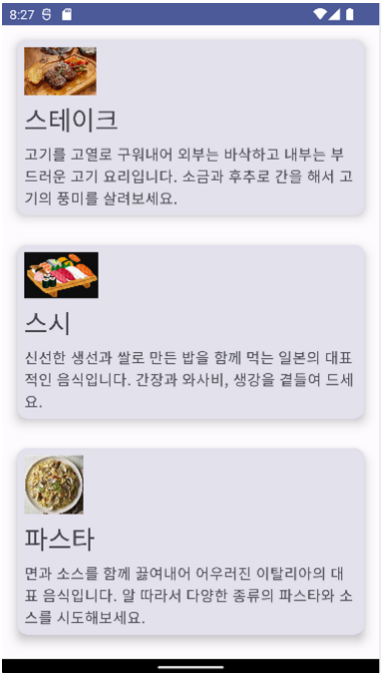