C#
static 함수
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace cs12_method
{
class Calc
{
public static int plus(int a, int b)
{ return a + b; }
public int minus(int a, int b)
{ return a - b; }
}
internal class Program
{
static void Main(string[] args)
{
int result=Calc.plus(1, 2);
Console.WriteLine(result);
int result1=new Calc().minus(1, 2);
Console.WriteLine(result1);
}
}
}
- public static int 로 선언한 plus는 객체 생성 없이 바로 calc 클래스의 plus 함수로 접근하여 main 에서 사용 할 수 있지만
- static 없이 선언하였기에 minus라는 함수를 사용하려면 new Calc().minus(1,2) 의 형태로 객체를 생성해야 클래스의 함수를 사용 할 수 있음
WPF
- .NET 콘솔앱으로 생성하면 솔루션탐색기 - 참조 우클릭 - 참조추가 - System.Windows.Forms 찾아서 추가
글자체 변경 WPF
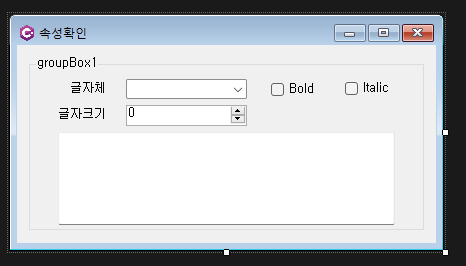
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace wf03_property
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void Form1_Load(object sender, EventArgs e)
{
gbxmain.Text = "컨트롤 학습";
var fonts = FontFamily.Families.ToList();
foreach ( var font in fonts )
{
fontname.Items.Add( font.Name );
}
fontsize.Minimum = 5;fontsize.Maximum = 40;
result.Text = "Hello, WinForm";
}
private void changefontstyle()
{
if (fontname.SelectedIndex < 0) return;
FontStyle style = FontStyle.Regular;
if(bold.Checked==true)
{
style |= FontStyle.Bold;
}
if(italic.Checked==true)
{
style |= FontStyle.Italic;
}
decimal font_size=fontsize.Value;
result.Font = new Font((string)fontname.SelectedItem, (float)font_size, style);
}
private void fontname_SelectedIndexChanged(object sender, EventArgs e)
{
changefontstyle();
}
private void bold_CheckedChanged(object sender, EventArgs e)
{
changefontstyle();
}
private void italic_CheckedChanged(object sender, EventArgs e)
{
changefontstyle();
}
private void fontsize_ValueChanged(object sender, EventArgs e)
{
changefontstyle();
}
}
}
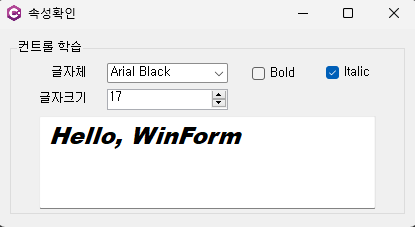