Array
- 배열의 디폴트 초기화
- 인스턴스 배열 (참조 변수 배열)은 모든 요소 null로 초기화
- 기본 자료형 배열은 모든 요소 0으로 초기화
Enhanced for문
for each
- 배열 요소 전체 출력
- Read만 되고 Write는 안된다.
int[] ar = {1,2,3,4,5};
// 일반적인 for 반복
for(int i = 0; i < ar.length; i++) {
System.out.println(ar[i]);
}
//for each
for(int e:ar) {
System.out.print(e + " ");
}
System.out.println();
int sum = 0;
//배열 요소 전체 합 출력
for(int e:ar) {
sum += e;
}
System.out.println("sum: " + sum);
2차원 배열
int[][] arr2 = new int[3][4];
// 3행 4열의 배열
int[][] arr2 = {
{1,2,3},
{4,5,6},
{7,8,9},
}
int[][] arr = { { 11 }, { 22, 33 }, { 44, 55, 66 } };
// 배열의 구조 대로 출력
for (int i = 0; i < arr.length; i++) {
for (int j = 0; j < arr[i].length; j++) { System.out.print(arr[i][j] + "\t");
}
System.out.println();
}
2차원 배열 메모리 구조도
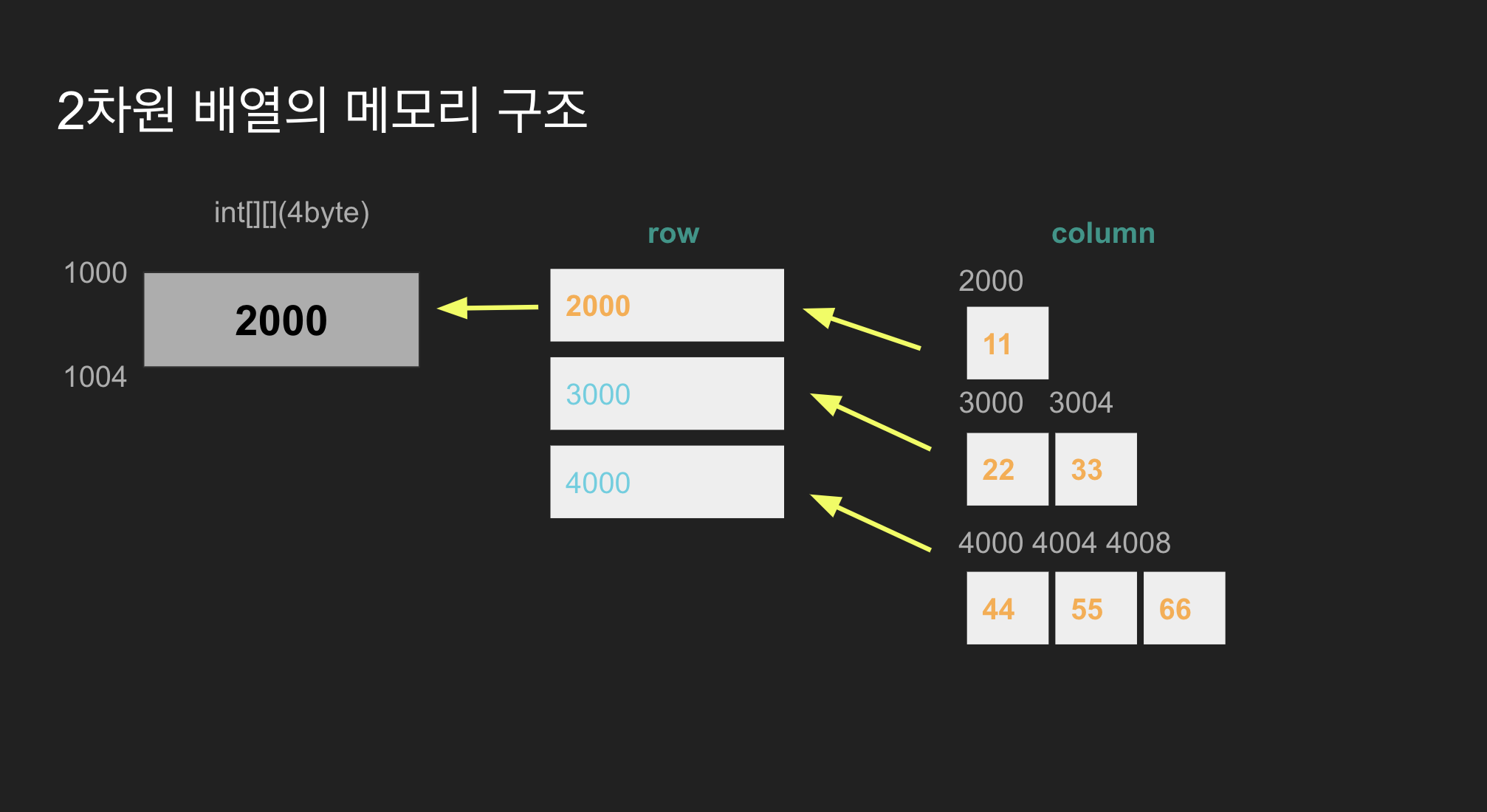
1~10까지의 행,열을 갖고, 대문자 알파벳을 랜덤으로 갖는 2차원 배열을 출력하시오.
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int rows, columns;
while (true) {
System.out.print("행의 크기를 입력하세요 (1~10): ");
rows = scanner.nextInt();
System.out.print("열의 크기를 입력하세요 (1~10): ");
columns = scanner.nextInt();
if (rows >= 1 && rows <= 10 && columns >= 1 && columns <= 10) {
break;
} else {
System.out.println("반드시 1~10 사이의 정수를 입력해야 합니다.");
}
}
char[][] array = new char[rows][columns];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
array[i][j] = (char) (65 + (int) (Math.random() * 26));
}
}
for (int i = 0; i < rows; i++) {
for (int j = 0; j < columns; j++) {
System.out.print(array[i][j] + " ");
}
System.out.println();
}
scanner.close();
}
String 2차원 배열 6행 6열을 만들고 행의 맨 위와 제일 앞 열은 각 인덱스를 저장하세요. 그리고 사용자에게 행과 열을 입력 받아 해당 좌표의 값을 'X'로 변환해 2차원 배열을 출력하세요.
public class Main{
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
String[][] array = new String[6][6];
// 행의 맨 위에 인덱스 저장
for (int i = 0; i < 6; i++) {
array[0][i] = String.valueOf(i);
}
// 열의 제일 앞에 인덱스 저장
for (int i = 0; i < 6; i++) {
array[i][0] = String.valueOf(i);
}
// 좌표 입력 받아 해당 좌표의 값을 'X'로 변환
while (true) {
System.out.print("행의 좌표를 입력하세요 (1~5): ");
int row = scanner.nextInt();
System.out.print("열의 좌표를 입력하세요 (1~5): ");
int column = scanner.nextInt();
if (row >= 1 && row <= 5 && column >= 1 && column <= 5) {
array[row][column] = "X";
break;
} else {
System.out.println("반드시 1~5 사이의 정수를 입력해야 합니다.");
}
}
// 배열 출력
printArray(array);
}
// 2차원 배열 출력 메소드
public static void printArray(String[][] array) {
for (int i = 0; i < array.length; i++) {
for (int j = 0; j < array[0].length; j++) {
if (array[i][j] == null) {
System.out.print(" "); // 빈 칸 출력
} else {
System.out.print(array[i][j] + " ");
} }
System.out.println();
}
System.out.println();
}
}