문제
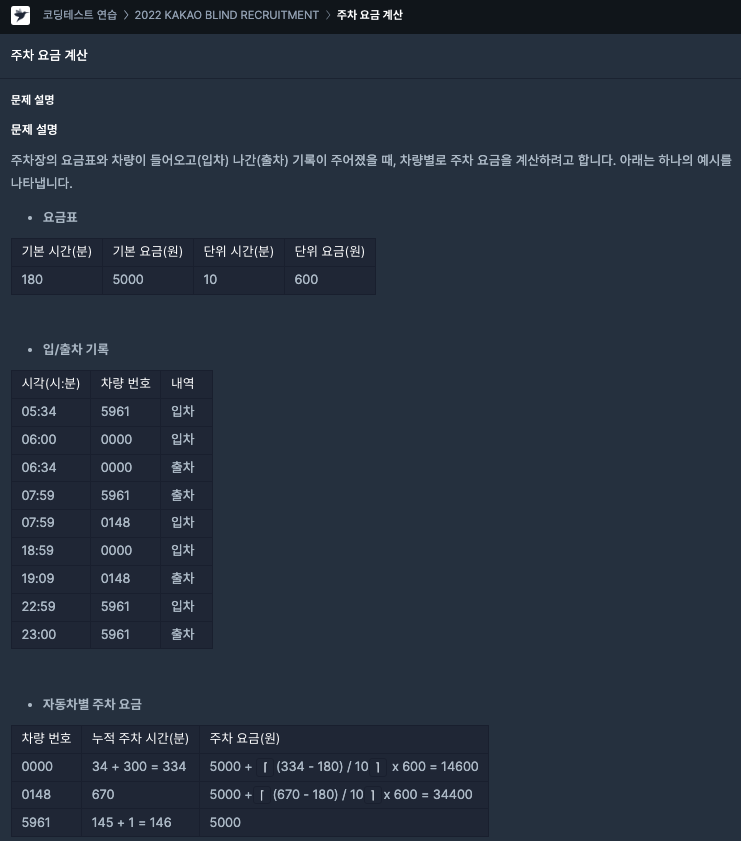
풀이
get_car_data_dict
함수로 입력받은 차량 번호별로 입차 시간, 출차 시간을 value 값을 갖는 dict으로 input data를 나누었다.
get_car_index_time
함수로 위 함수에서 차량 번호별로 분리된 딕셔너리 데이터로 시간을 추출해서 누적 주차 시간을 구하였다.
get_parking_fees
함수로 각 차량 번호별로 주차 요금을 구했다.
- 주의할 점은 초과한 시간이 단위 시간으로 나누어 떨어지지 않으면, 올림처리를 해야하는 문제이다.
코드
from math import ceil
def split_time(time: str):
return map(int, time.split(':'))
def calculate_time(in_hour: int, in_minute: int, out_hour: int, out_minute: int) -> int:
return (out_hour - in_hour) * 60 + (out_minute - in_minute)
def get_car_date_dict(records: list) -> dict:
car_data_dict = {}
for data in records:
time, car_index, car_type = data.split()
if car_index not in car_data_dict:
car_data_dict[car_index] = {car_type: [time]}
else:
if car_type not in car_data_dict[car_index]:
car_data_dict[car_index][car_type] = [time]
else:
car_data_dict[car_index][car_type].append(time)
return car_data_dict
def get_car_index_time(car_data_dict: dict) -> dict:
car_index_time = {}
for key, value in car_data_dict.items():
for index, time in enumerate((value['IN'])):
in_hour, in_minute = split_time(value['IN'][index])
try:
out_hour, out_minute = split_time(value['OUT'][index])
except:
out_hour, out_minute = 23, 59
if key not in car_index_time:
car_index_time[key] = calculate_time(in_hour, in_minute, out_hour, out_minute)
else:
car_index_time[key] += calculate_time(in_hour, in_minute, out_hour, out_minute)
return car_index_time
def get_parking_fees(fees: list, car_index_time: dict) -> list:
answer = []
for _, time in sorted(car_index_time.items(), key=lambda x: x[0]):
money = fees[1] + ceil((time - fees[0]) / fees[2]) * fees[-1] if time > fees[0] else fees[1]
answer.append(money)
return answer
def solution(fees: list, records: list) -> list:
car_data_dict = get_car_date_dict(records)
car_index_time = get_car_index_time(car_data_dict)
return get_parking_fees(fees, car_index_time)
if __name__ == '__main__':
print(solution(
fees=[180, 5000, 10, 600],
records=["05:34 5961 IN", "06:00 0000 IN", "06:34 0000 OUT", "07:59 5961 OUT", "07:59 0148 IN", "18:59 0000 IN", "19:09 0148 OUT", "22:59 5961 IN", "23:00 5961 OUT"]
))
print(solution(
fees=[120, 0, 60, 591],
records=["16:00 3961 IN", "16:00 0202 IN", "18:00 3961 OUT", "18:00 0202 OUT", "23:58 3961 IN"]
))
print(solution(
fees=[1, 461, 1, 10],
records=["00:00 1234 IN"]
))
결과
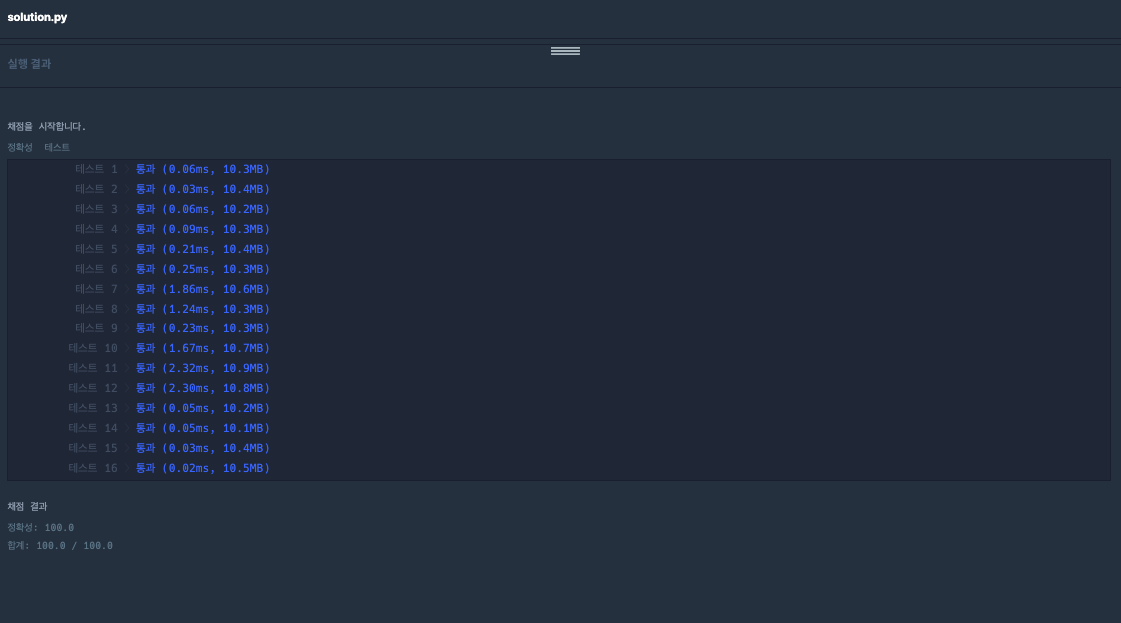
출처 & 깃허브
프로그래머스
깃허브