문제
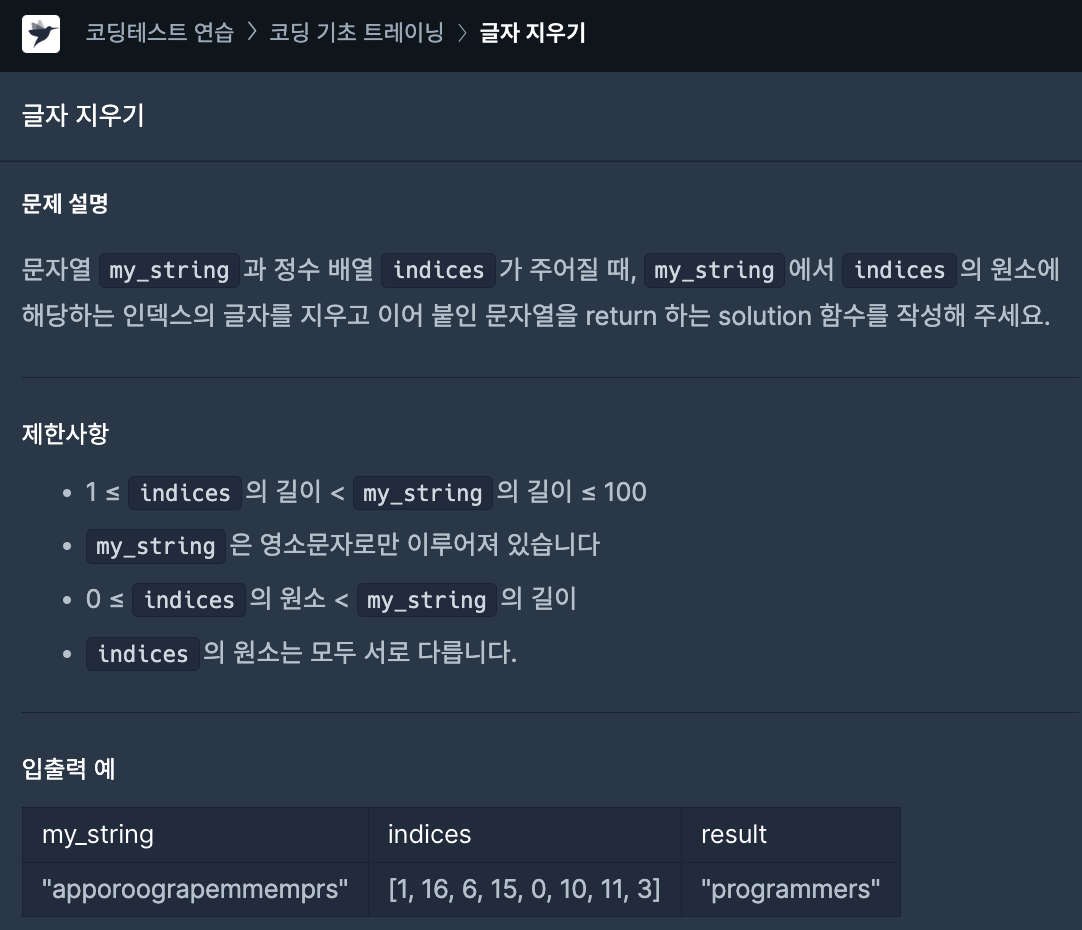
Python Code
from typing import List
def solution(my_string: str, indices: List[int]) -> str:
result = ""
for i in range(len(my_string)):
if i not in indices:
result += my_string[i]
return result
Go Code
func contains(arr []int, target int) bool {
for _, num := range arr {
if num == target {
return true
}
}
return false
}
func solution(myString string, indices []int) string {
result := ""
for i, char := range myString {
if !contains(indices, i) {
result += string(char)
}
}
return result
}
Java Code
public class Solution {
public static String solution(String myString, int[] indices) {
StringBuilder result = new StringBuilder();
for (int i = 0; i < myString.length(); i++) {
if (!contains(indices, i)) {
result.append(myString.charAt(i));
}
}
return result.toString();
}
public static boolean contains(int[] arr, int target) {
for (int num : arr) {
if (num == target) {
return true;
}
}
return false;
}
}
결과
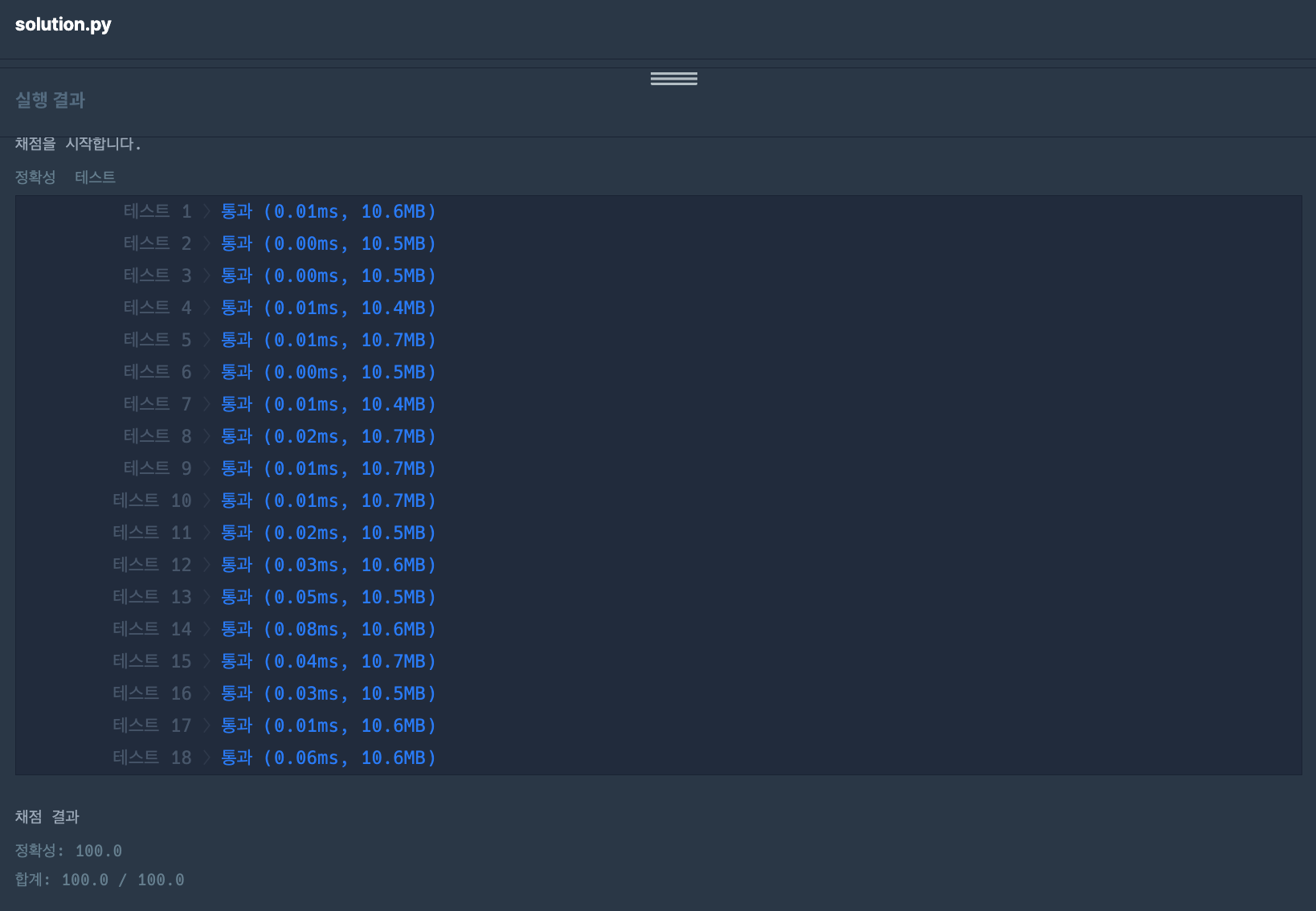
문제 출처 & 깃허브
Programmers
Github