draw contours
import cv2
import numpy as np
import matplotlib.pyplot as plt
image = cv2.imread('./data/shapes.png')
image_copy = image.copy()
imgray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
ret, imthres = cv2.threshold(imgray, 40, 255, cv2.THRESH_BINARY_INV)
plt.imshow(imthres)
plt.show()
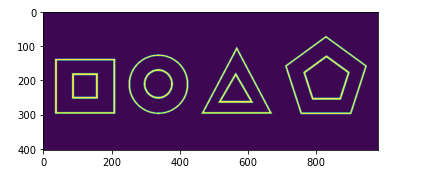
cv2.drawContours(image, contour, -1, (0, 255, 0), 4)
cv2.drawContours(image_copy, contour2, -1, (0, 255, 0), 4)
for i in contour :
for j in i :
cv2.circle(image, tuple(j[0]), 1, (255, 0, 0), -1)
cv2.imshow('CHAIN_APPROX_NONE', image)
cv2.imshow('CHAIN_APPROX_SIMPLE', image_copy)
cv2.waitKey(0)
cv2.destroyAllWindows()
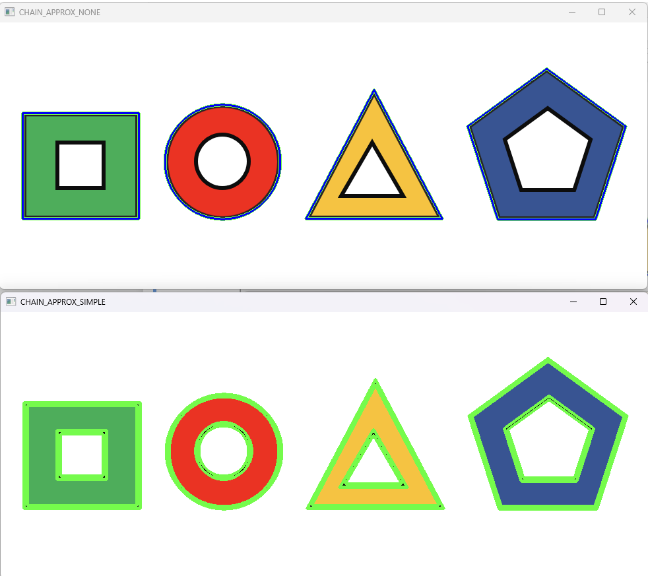
HoughCircles
image = cv2.imread('./data/ban_sign.jpg')
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (19,19), 0)
plt.imshow(cv2.cvtColor(blur, cv2.COLOR_GRAY2RGB))
plt.show()
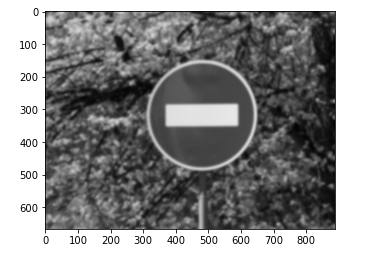
circles = cv2.HoughCircles(blur, cv2.HOUGH_GRADIENT,
1.5, 30, None, 200)
if circles is not None :
circles = np.uint16(np.around(circles))
for i in circles[0,:] :
cv2.circle(image, (i[0], i[1]), i[2], (0, 255, 0), 2)
cv2.circle(image, (i[0], i[1]), 2, (0, 0, 255), 5)
cv2.imshow('hough circle', image)
cv2.waitKey(0)
cv2.destroyAllWindows()
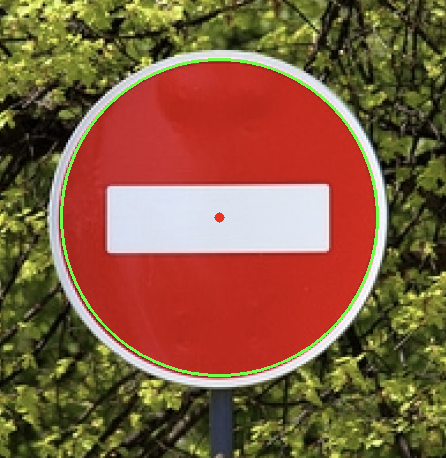
convexhull
image = cv2.imread('./data/hands.jpg')[:1100,:]
image = cv2.resize(image, (0, 0), fx=0.5, fy=0.5)
image_copy = image.copy()
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (9,9), 0)
ret, thr = cv2.threshold(blur, 170 , 255, cv2.THRESH_BINARY_INV)
plt.imshow(thr)
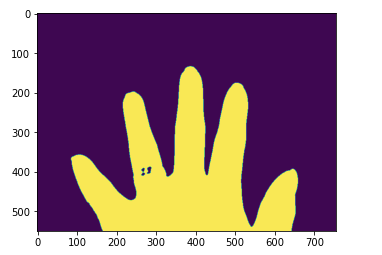
contours, heiarchy = cv2.findContours(thr, cv2.RETR_EXTERNAL,
cv2.CHAIN_APPROX_NONE)[-2:]
contour = contours[0]
cv2.drawContours(image, contour, -1, (255, 0, 255), 5)
plt.imshow(cv2.cvtColor(image, cv2.COLOR_BGR2RGB))
plt.show()
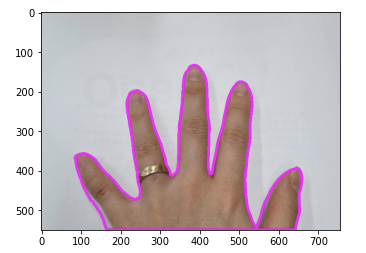
hull = cv2.convexHull(contour, returnPoints=False)
defects = cv2.convexityDefects(contour, hull)
for i in range(defects.shape[0]) :
startP, endP, farthestP, dist = defects[i, 0]
farthest = tuple(contour[farthestP][0])
dist = dist / 256.0
if dist > 1 :
cv2.circle(image_copy,farthest, 5, (0, 0, 255), -1)
cv2.imshow('contour', image)
cv2.imshow('contex hull', image_copy)
cv2.waitKey(0)
cv2.destroyAllWindows()
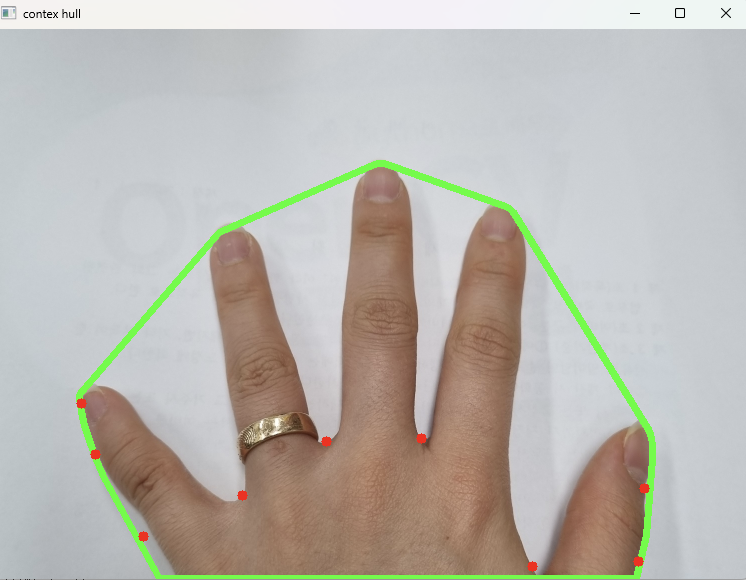
matchShapes
target = cv2.imread('./data/cross_walk_sign.jpg')
shapes = cv2.imread('./data/shapes.png')
tgray = cv2.cvtColor(target, cv2.COLOR_BGR2GRAY)
sgray = cv2.cvtColor(shapes, cv2.COLOR_BGR2GRAY)
ret, tar_thr = cv2.threshold(tgray, 120, 255, cv2.THRESH_BINARY_INV)
ret, sha_thr = cv2.threshold(sgray, 120, 255, cv2.THRESH_BINARY_INV)
contours_target, _ = cv2.findContours(tar_thr, cv2.RETR_EXTERNAL,
cv2.CHAIN_APPROX_SIMPLE)[-2:]
contours_shape, _ = cv2.findContours(sha_thr, cv2.RETR_EXTERNAL,
cv2.CHAIN_APPROX_SIMPLE)[-2:]
matches = []
for c in contours_shape :
match = cv2.matchShapes(contours_target[0], c, cv2.CONTOURS_MATCH_I2, 0.0)
matches.append((match, c))
cv2.putText(shapes, f"{match:.2f}", tuple(c[0][0]),
cv2.FONT_HERSHEY_PLAIN, 1, (0,0,255), 1)
matches.sort(key=lambda x : x[0])
cv2.drawContours(shapes, [matches[0][1]], -1, (0, 255, 0), 3)
cv2.imshow('target', target)
cv2.imshow('shapes', shapes)
cv2.waitKey(0)
cv2.destroyAllWindows()
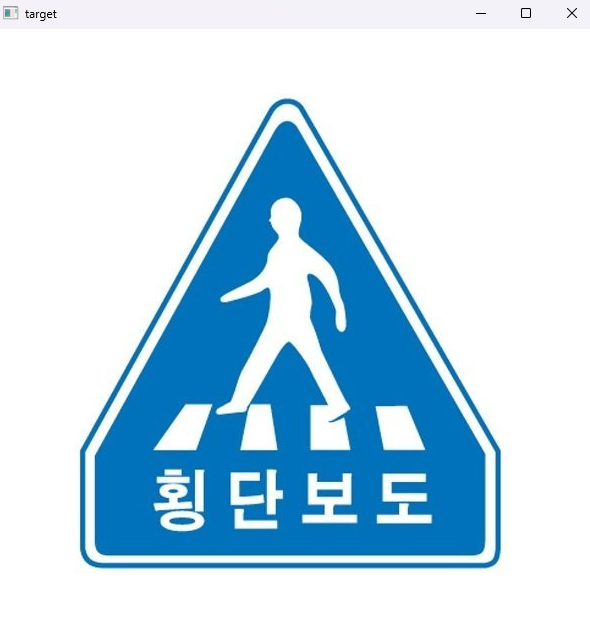
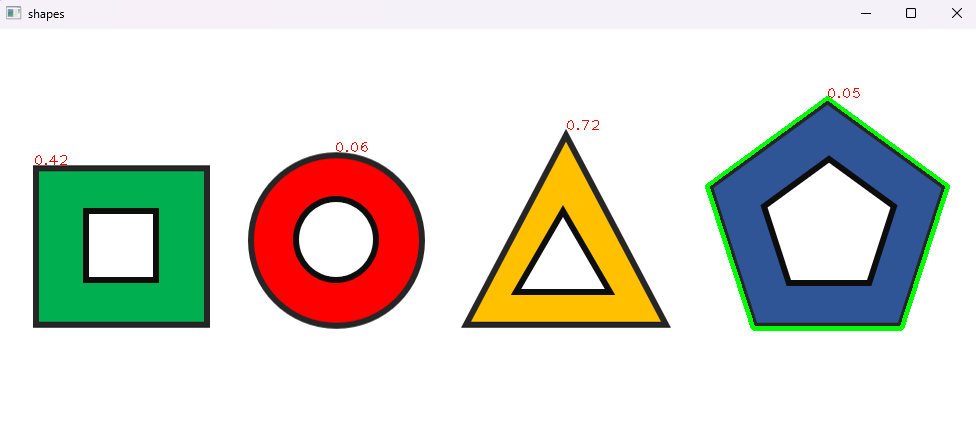
approxPolyDP
image = cv2.imread('./data/signs.jpeg')
image_copy = image.copy()
imgray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(imgray, (3,3), 0)
ret, thr = cv2.threshold(imgray, 110, 255, cv2.THRESH_BINARY_INV)
plt.imshow(cv2.cvtColor(thr, cv2.COLOR_BGR2RGB ))
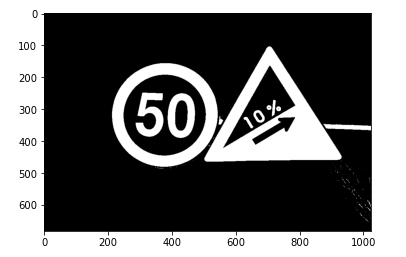
contours, _ = cv2.findContours(thr,
cv2.RETR_EXTERNAL,
cv2.CHAIN_APPROX_SIMPLE)[-2:]
for cont in contours :
approx = cv2.approxPolyDP(cont, 0.005*cv2.arcLength(contour,True), True)
vertex = len(approx)
if vertex >= 3 :
print(vertex)
cv2.drawContours(image_copy, [approx], -1, (0, 255, 0), 5)
mmt = cv2.moments(cont)
cx, cy = int(mmt['m10']/mmt['m00']), int(mmt['m01']/mmt['m00'])
if vertex == 3 :
cv2.putText(image_copy, 'Triangle', (cx, cy+200), cv2.FONT_HERSHEY_COMPLEX_SMALL,
2, (0, 255, 0 ), 2)
if vertex > 6 :
cv2.putText(image_copy, 'Circle', (cx, cy+200), cv2.FONT_HERSHEY_COMPLEX_SMALL,
2, (0, 255, 0), 2)
plt.imshow( cv2.cvtColor(image_copy, cv2.COLOR_BGR2RGB ))
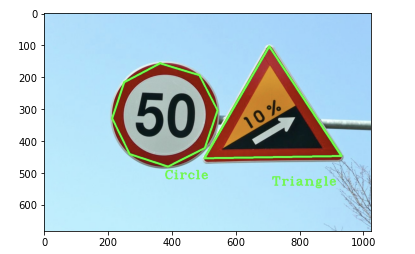