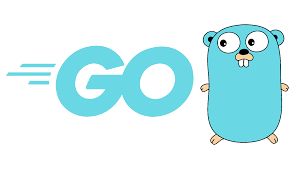
본 게시글은 Golang Tutorial for Beginners | Full Go Course을 학습하며 작성한 개인 노트입니다.
Concurrency
"time"
time.Sleep(timeAmount)
- stops/blocks the current thread execution for defined duration
Concurrency
Single thread execution
- step by step execution of the code
- later code has to wait until the earlier code finishes
Execute some functions in a separate thread >> CONCURRENCY
- main thread doesn't wait for these functions to finish
- next line of code is executed immediately
go keyword
go sendTicket(userTickets, firstName, lastName, email)
Problems
Main goroutine exits before a function had time to start & execute the code.
By default, main goroutine does not wait for other threads
Waitgroup
- waits for the launched goroutine to finish
- package "sync": provides synchronization functionality
var wg = sync.WaitGroup{}
...
// before starting another thread, specify how many threads the main goroutine will wait for
wg.Add(1)
go function()
wg.Add(#)
: sets the number of goroutines to wait for
- increases the counter by the provided number
wg.Wait()
: blocks until the WaitGroup counter is 0
wg.DOne()
: function tells main goroutine that it's done so there's no need to wait longer
How is Goroutine different?
- Go uses Green thread
- green thread: abstraction of an actual thread
- managed by the go rutime
- only interacts with high level goroutines
- no need to interact w/ kernels/hardware, etc.
- cheaper, lightweight
- easy communication between threads
- Channels: built-in functionality for goroutines to talk with one another