📌문제
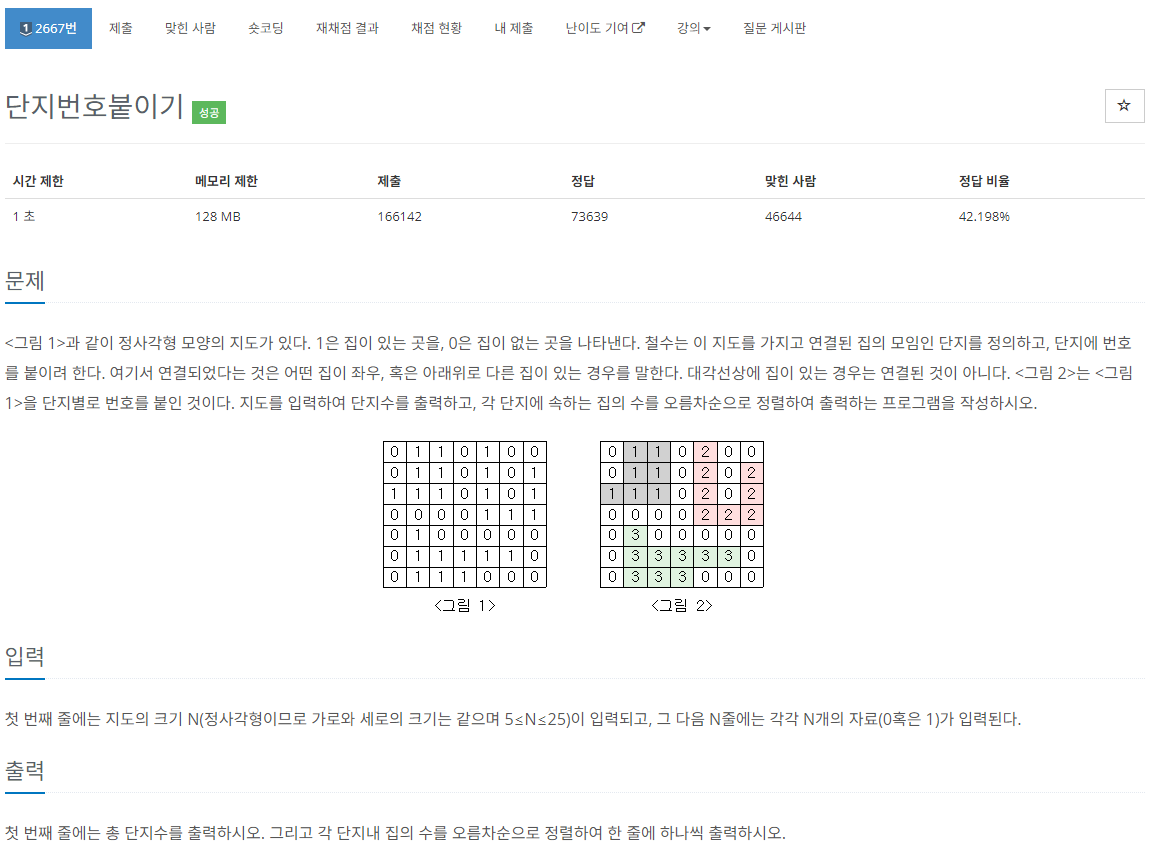
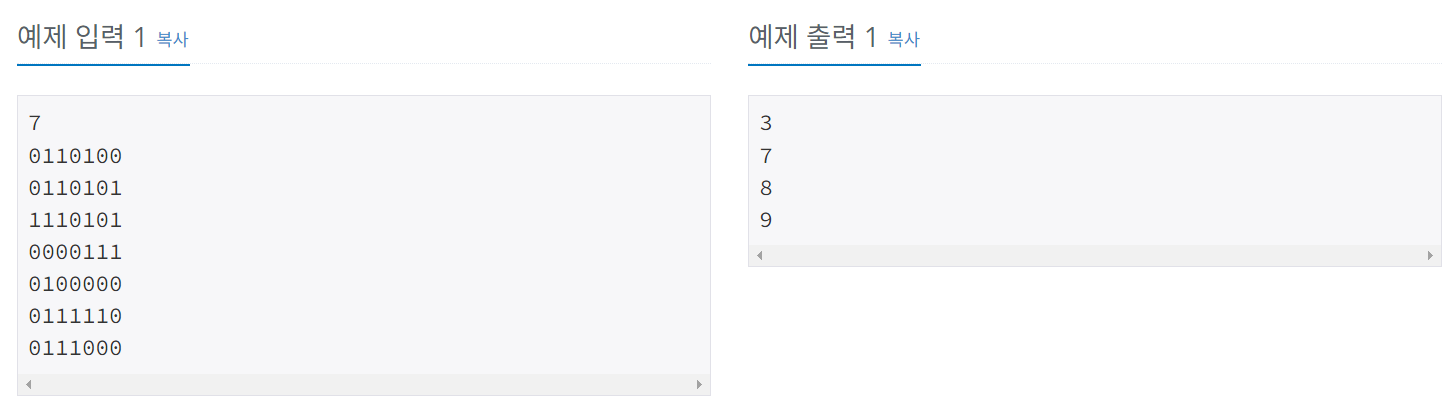
📌나의 코드
def dfs(x, y):
global cnt
if x <= -1 or x >= n or y <= -1 or y >= n:
return False
if map[x][y] == 1:
map[x][y] = 0
cnt += 1
dfs(x - 1, y)
dfs(x + 1, y)
dfs(x, y - 1)
dfs(x, y + 1)
return True, cnt
return False
n = int(input())
map = [list(map(int, input())) for _ in range(n)]
homes = 0
cnts = []
for i in range(n):
for j in range(n):
cnt = 0
if dfs(i, j):
homes += 1
cnts.append(cnt)
cnts.sort()
print(homes)
for c in cnts:
print(c)
📌다른 사람 코드
def dfs(x, y):
global cnt
if x < 0 or x >= n or y < 0 or y >= n:
return False
if map[x][y] == 1:
cnt += 1
map[x][y] = 0
for i in range(4):
nx, ny = x + dxs[i], y + dys[i]
dfs(nx, ny)
return True
return False
n = int(input())
map = [list(map(int, input())) for _ in range(n)]
dxs, dys = [-1, 1, 0, 0], [0, 0, -1, 1]
cnt = 0
cnts = []
result = 0
for i in range(n):
for j in range(n):
if dfs(i, j):
cnts.append(cnt)
result += 1
cnt = 0
cnts.sort()
print(result)
for c in cnts:
print(c)
from collections import deque
def bfs(x, y):
queue = deque()
queue.append((x, y))
map[x][y] = 0
cnt = 1
while queue:
x, y = queue.popleft()
for i in range(4):
nx, ny = x + dxs[i], y + dys[i]
if nx <= -1 or nx >= n or ny <= -1 or ny >= n:
continue
if map[nx][ny] == 1:
map[nx][ny] = 0
queue.append((nx, ny))
cnt += 1
return cnt
n = int(input())
map = [list(map(int, input())) for _ in range(n)]
dxs, dys = [-1, 1, 0, 0], [0, 0, -1, 1]
cnts = []
for i in range(n):
for j in range(n):
if map[i][j] == 1:
cnts.append(bfs(i, j))
cnts.sort()
print(len(cnts))
for c in cnts:
print(c)