Azure Search Document 링크
1. Search Client
object SearchClient {
private val okHttpClientBuilder = OkHttpClient().newBuilder()
.connectTimeout(30, TimeUnit.SECONDS)
.readTimeout(30, TimeUnit.SECONDS)
.writeTimeout(15, TimeUnit.SECONDS)
.addInterceptor { chain ->
val request = chain.request().newBuilder()
.addHeader("Content-Type" ,"application/json")
.addHeader("api-key", "YOUR_KEY")
chain.proceed(request.build())
}
.build()
private val moshi = Moshi.Builder()
.add(KotlinJsonAdapterFactory())
.add(OffsetDateTimeAdapter())
.build()
var retrofit = Retrofit.Builder()
.baseUrl("https://YOUR_SERVICE_NAME.search.windows.net")
.client(okHttpClientBuilder)
.addConverterFactory(MoshiConverterFactory.create(moshi))
.build()
}
2. Search Api
interface SearchApi {
@POST("indexes/{index}/docs/search?api-version=2021-04-30-Preview")
suspend fun searchHospitals(@Body body: SearchOption, @Path("index") index: String): Response<SearchHospitalResult>
@POST("indexes/{index}/docs/search?api-version=2021-04-30-Preview")
suspend fun searchDoctors(@Body body: SearchOption, @Path("index") index: String): Response<SearchDoctorResult>
@POST("indexes/{index}/docs/search?api-version=2021-04-30-Preview")
suspend fun searchDeals(@Body body: SearchOption, @Path("index") index: String): Response<SearchDealResult>
@POST("indexes/{index}/docs/search?api-version=2021-04-30-Preview")
suspend fun searchDepartments(@Body body: SearchOption, @Path("index") index: String): Response<SearchDepartmentResult>
@POST("indexes/{index}/docs/search?api-version=2021-04-30-Preview")
suspend fun searchSpecialties(@Body body: SearchOption, @Path("index") index: String): Response<SearchSpecialtyResult>
}
3. Search ViewModel
private val searchApi = SearchClient.retrofit.create(SearchApi::class.java)
fun searchAutoComplete(searchTerm: String, index: String, page: Int = 1, limit: Int = 20){
val searchOption = SearchOption(
search = "$searchTerm*",
queryType = "full",
searchFields = "Translations/Name",
skip = limit.times(page.minus(1)),
top = 3)
when (index) {
"idx-hospitals-int" -> {
searchHospitals(searchOption, index, page)
}
"idx-doctors-int" -> {
searchDoctors(searchOption, index, page)
}
"idx-deals-int" -> {
searchDeals(searchOption, index, page)
}
"idx-departments-int" -> {
searchDepartments(searchOption, index, page)
}
"idx-specialties-int" -> {
searchSpecialties(searchOption, index, page)
}
}
}
private fun searchHospitals(searchOption: SearchOption, index: String, page: Int = 1) {
val actionName = "searchHospitals"
viewModelScope.launch(Dispatchers.Main) {
val result = searchApi.searchHospitals(searchOption, index)
try {
if (result.isSuccessful) {
if (result.code() == 200) {
result.body()?.let { data ->
_hospitals.postValue(data.value)
}
}
} else {
Log.d("debug", "$actionName failed")
}
} catch (e: Exception) {
Log.d("debug", "$actionName failed: ${e.message}")
}
}
}
4. Result
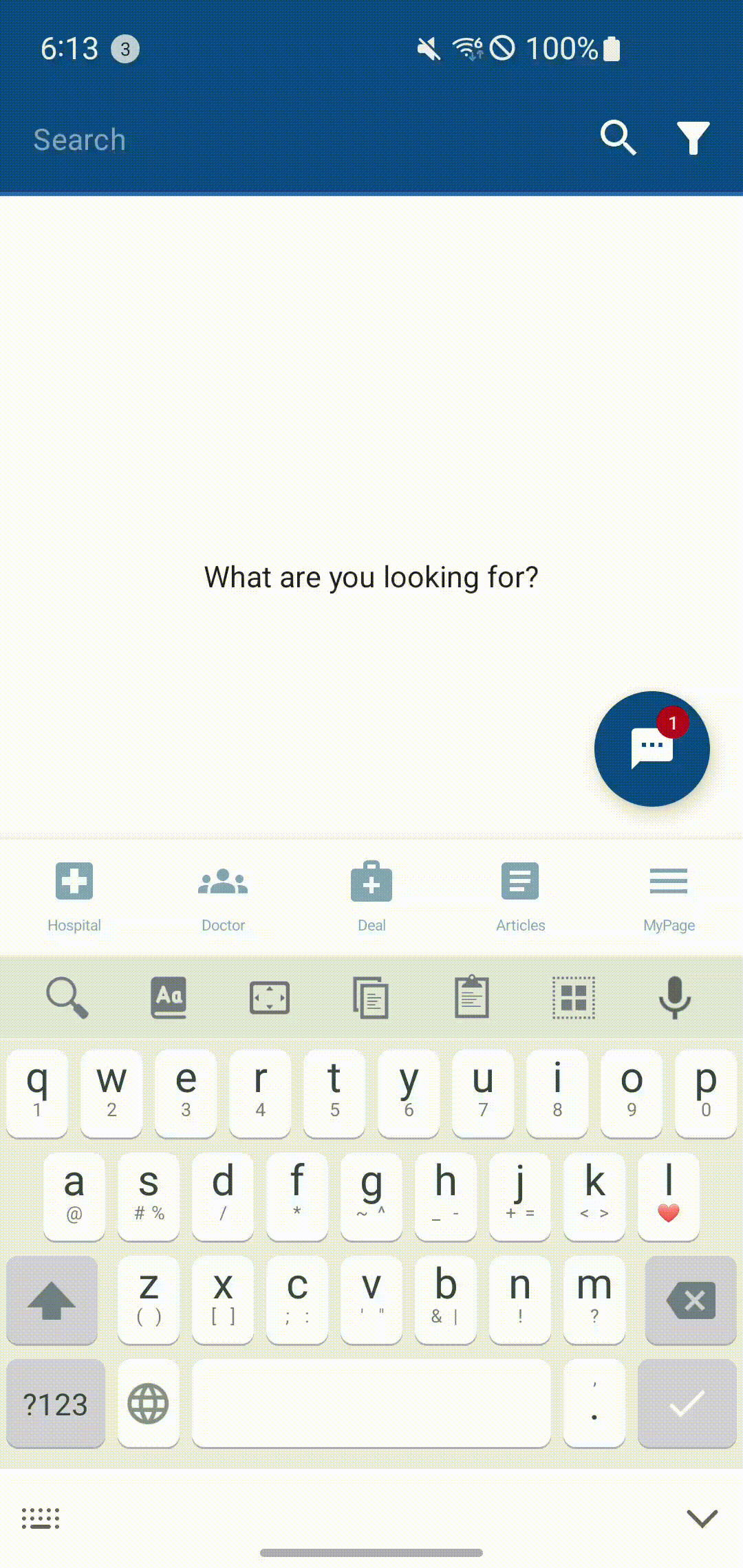