문제
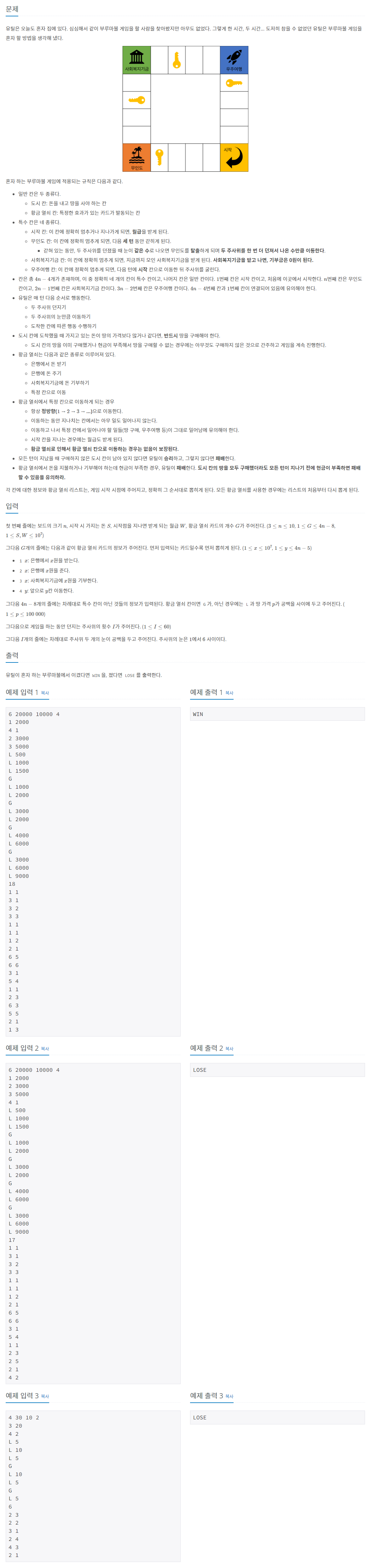
코드
import java.io.*;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.Queue;
import java.util.StringTokenizer;
public class q28457 {
public static void main(String[] args) throws IOException {
int diceCount = 2;
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
StringTokenizer tk = new StringTokenizer(br.readLine(), " ");
int n = Integer.parseInt(tk.nextToken());
BigInteger S = new BigInteger(tk.nextToken());
int W = Integer.parseInt(tk.nextToken());
int G = Integer.parseInt(tk.nextToken());
Queue<String> goldenKey = new LinkedList<>();
for(int i=0; i<G; i++) goldenKey.offer(br.readLine());
String[] board = new String[4*n - 4];
ArrayList<Integer> building = new ArrayList<>();
for(int k=0; k<board.length; k++) {
if(k % (n-1) == 0 || k % (2*n-2) == 0 || k % (3*n-3) == 0) continue;
board[k] = br.readLine();
tk = new StringTokenizer(board[k], " ");
if(tk.nextToken().equals("L")) building.add(k);
}
int I = Integer.parseInt(br.readLine());
int prePath = 0;
int currentPath = 0;
boolean island = false;
int islandCount = 0;
int social = 0;
boolean golden4 = false;
for(int k=0; k<I; k++) {
if(golden4) {
golden4 = false;
if(currentPath == 0) S = S.add(new BigInteger(String.valueOf(W)));
} else {
int[] dice = new int[diceCount];
int diceSum = 0;
tk = new StringTokenizer(br.readLine(), " ");
for(int i=0; i<diceCount; i++) {
dice[i] = Integer.parseInt(tk.nextToken());
diceSum += dice[i];
}
if(island) {
if(dice[0] == dice[1]) {
island = false;
continue;
} else {
islandCount++;
}
if(islandCount == 3) {
island = false;
islandCount = 0;
}
continue;
}
prePath = currentPath;
if((prePath + diceSum) / board.length > 0 || currentPath == 0) S = S.add(new BigInteger(String.valueOf(W * ((prePath + diceSum) / board.length))));
currentPath = (prePath + diceSum) % board.length;
}
if (currentPath == 0) {}
else if(currentPath == n-1) {
island = true;
}
else if(currentPath == 2*n-2) {
S = S.add(new BigInteger(String.valueOf(social)));
social = 0;
}
else if(currentPath == 3*n-3) {
currentPath = 0;
S = S.add(new BigInteger(String.valueOf(W)));
}
else {
tk = new StringTokenizer(board[currentPath], " ");
String command = tk.nextToken();
if(command.equals("G")) {
String goldenKeyItem = goldenKey.poll();
goldenKey.offer(goldenKeyItem);
tk = new StringTokenizer(goldenKeyItem, " ");
int goldenCommand = Integer.parseInt(tk.nextToken());
int p = Integer.parseInt(tk.nextToken());
switch(goldenCommand) {
case 1:
S = S.add(new BigInteger(String.valueOf(p)));
break;
case 2:
S = S.subtract(new BigInteger(String.valueOf(p)));
break;
case 3:
S = S.subtract(new BigInteger(String.valueOf(p)));
social += p;
break;
case 4:
golden4 = true;
if((currentPath + p) / board.length > 0 || currentPath == 0) S = S.add(new BigInteger(String.valueOf(W * ((currentPath + p) / board.length))));
currentPath = (currentPath + p) % board.length;
k--;
}
}
else {
int p = Integer.parseInt(tk.nextToken());
if(S.compareTo(BigInteger.valueOf(p)) == 0 || S.compareTo(BigInteger.valueOf(p)) == 1) {
if(building.contains(new Integer(currentPath))) {
S = S.subtract(new BigInteger(String.valueOf(p)));
building.remove(new Integer(currentPath));
}
}
}
}
if(S.compareTo(BigInteger.valueOf(0)) == -1) {
bw.write("LOSE");
bw.flush();
return;
}
}
if(building.size() > 0 || S.compareTo(BigInteger.valueOf(0)) == -1) {
bw.write("LOSE");
} else {
bw.write("WIN");
}
bw.flush();
}
}