문제
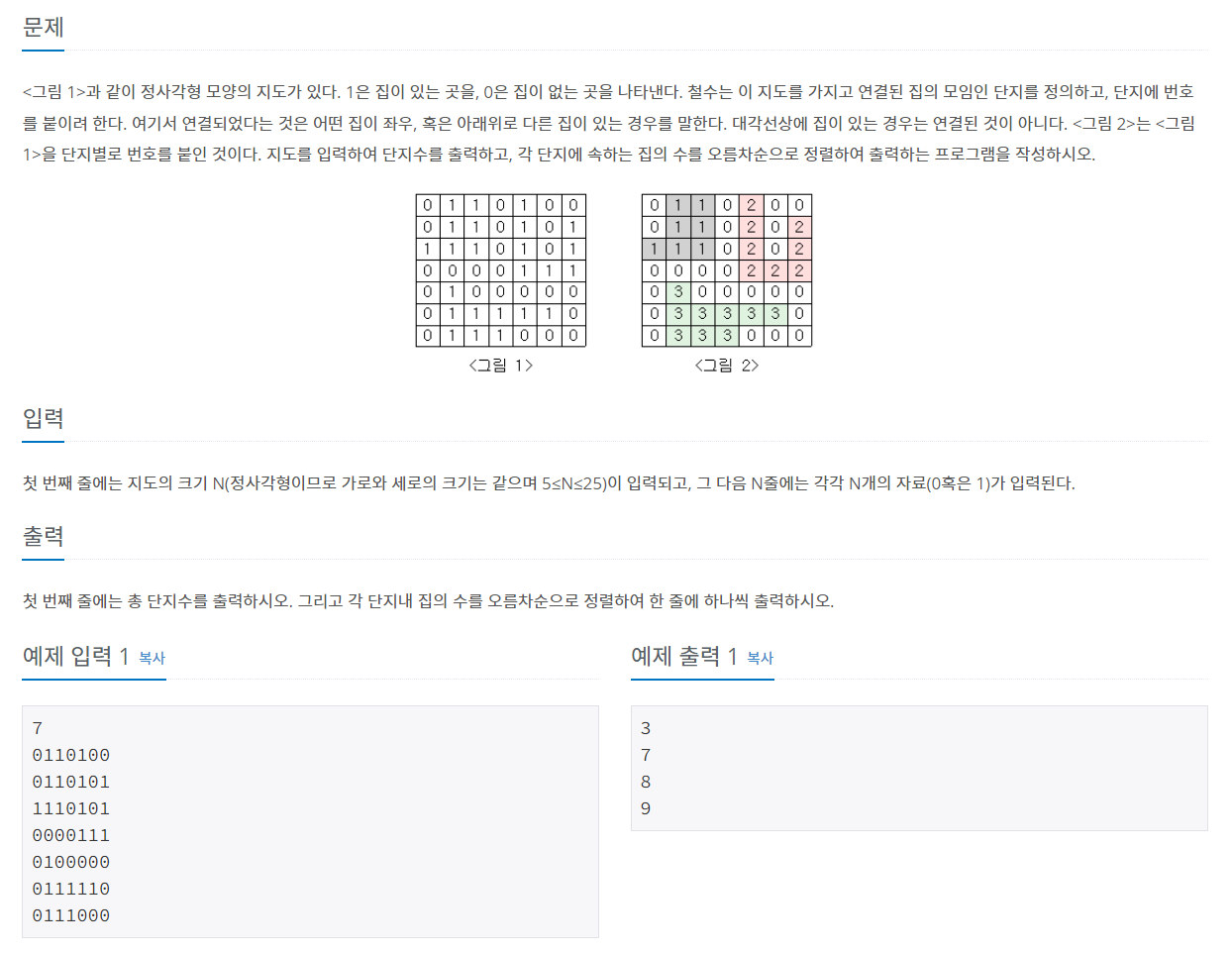
코드
import java.io.*;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collections;
public class q2667 {
static ArrayList<ArrayList<Integer>> graph;
static boolean[][] house;
static int N;
static int[] visited;
static int count = 1;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
N = Integer.parseInt(br.readLine());
house = new boolean[N][N];
visited = new int[N*N];
for(int i=0; i<N; i++) {
String[] input = br.readLine().split("");
for(int j=0; j<N; j++) {
if(input[j].equals("1")) house[i][j] = true;
}
}
graph = new ArrayList<>();
for(int i=0; i<N*N; i++) graph.add(new ArrayList<>());
for(int i=0; i<N; i++) {
for(int j=0; j<N; j++) {
if(house[i][j]) {
try {
if(house[i-1][j]) {
graph.get(i*N + j).add((i-1)*N + j);
}
} catch (Exception e) {}
try {
if(house[i+1][j]) {
graph.get(i*N + j).add((i+1)*N + j);
}
} catch (Exception e) {}
try {
if(house[i][j-1]) {
graph.get(i*N + j).add(i*N + (j-1));
}
} catch (Exception e) {}
try {
if(house[i][j+1]) {
graph.get(i*N + j).add(i*N + (j+1));
}
} catch (Exception e) {}
}
}
}
for(int i=0; i<N*N; i++) {
if(dfs(i)) count++;
}
int[] countComplex = new int[count];
for(int i=0; i<N*N; i++) {
if(visited[i] != 0) countComplex[visited[i]]++;
}
Arrays.sort(countComplex);
countComplex[0] = count-1;
for(int i=0; i<countComplex.length; i++) bw.write(countComplex[i]+"\n");
bw.flush();
}
public static boolean dfs(int R) {
if(house[R/N][R%N] && visited[R] == 0) {
visited[R] = count;
for(int v : graph.get(R)) {
if(visited[v] == 0) dfs(v);
}
return true;
}
return false;
}
}