문제
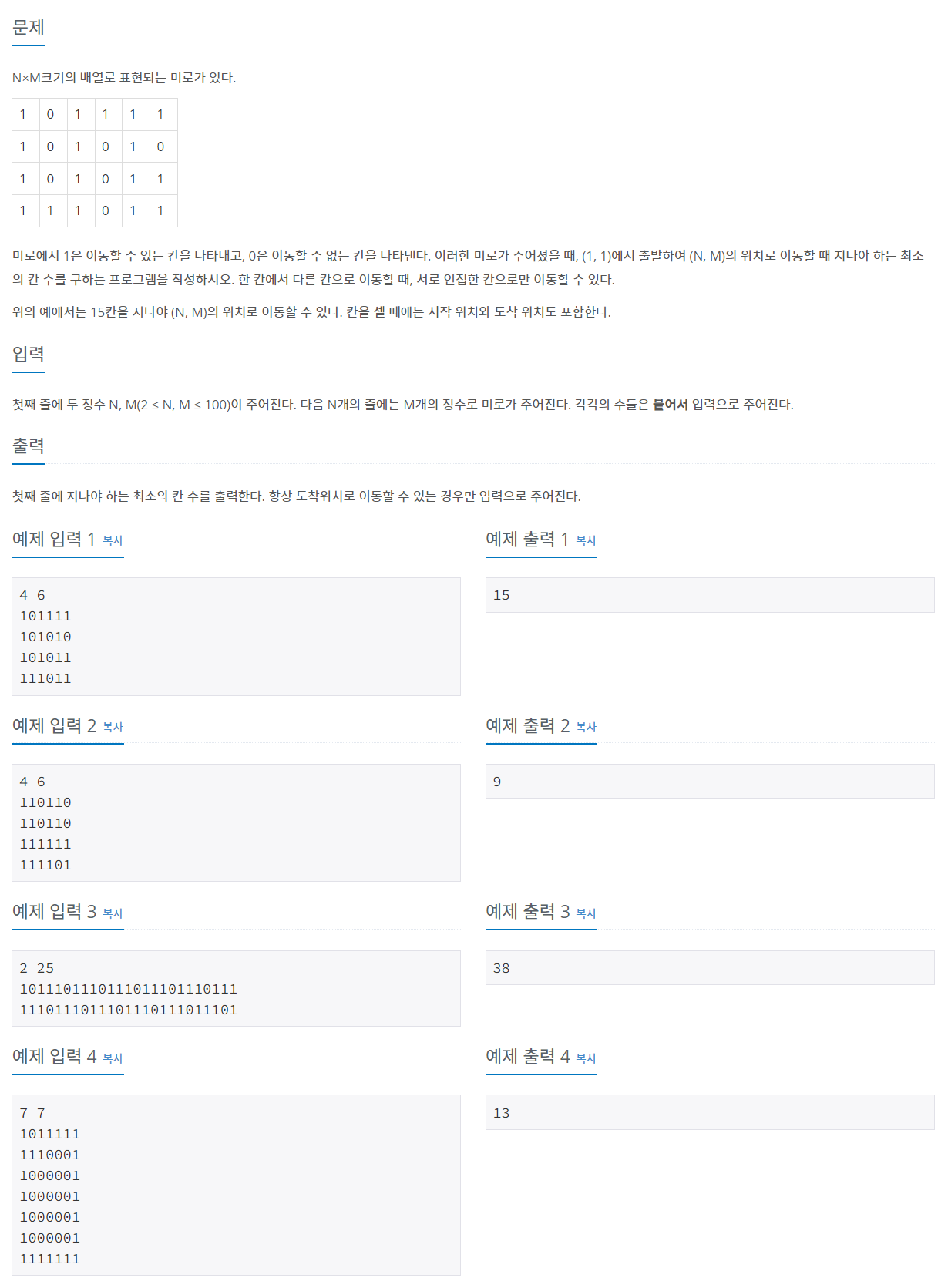
코드
import java.io.*;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
public class q2178 {
public static ArrayList<Integer> queue = new ArrayList<>();
public static ArrayList<ArrayList<Integer>> graph;
public static int[] visited;
public static boolean[][] maze;
public static int N, M;
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
String[] input = br.readLine().split(" ");
N = Integer.parseInt(input[0]);
M = Integer.parseInt(input[1]);
visited = new int[N*M];
maze = new boolean[N][M];
for(int i=0; i<N; i++) {
input = br.readLine().split("");
for(int j=0; j<M; j++) {
maze[i][j] = (Integer.parseInt(input[j]) != 0);
}
}
graph = new ArrayList<>();
for(int i=0; i<N; i++) {
for(int j=0; j<M; j++) {
graph.add(new ArrayList<>());
if(maze[i][j]) {
try {
if(maze[i-1][j]) graph.get(i*M + j).add((i-1)*M + j);
} catch (Exception e) {}
try {
if(maze[i+1][j]) graph.get(i*M + j).add((i+1)*M + j);
} catch (Exception e) {}
try {
if(maze[i][j-1]) graph.get(i*M + j).add(i*M + (j-1));
} catch (Exception e) {}
try {
if(maze[i][j+1]) graph.get(i*M + j).add(i*M + (j+1));
} catch (Exception e) {}
}
}
}
bfs(0);
bw.write(visited[N*M-1] + "");
bw.flush();
}
public static void bfs(int R) {
HashMap<Integer, Integer> parent = new HashMap<>();
if(maze[R/M][R%M]) {
visited[R] = 1;
enqueue(R);
while(queue.size() > 0) {
int u = dequeue();
for(int v : graph.get(u)) {
if(!parent.containsKey(v)) {
parent.put(v, u);
} else {
if(visited[u] < visited[parent.get(v)]) parent.put(v, u);
}
if(visited[v] == 0 || visited[v] > visited[parent.get(v)] + 1) {
visited[v] = visited[parent.get(v)] + 1;
enqueue(v);
}
}
}
}
}
public static void enqueue(int v) {
queue.add(v);
}
public static int dequeue() {
int out = queue.get(queue.size()-1);
queue.remove(queue.size()-1);
return out;
}
}